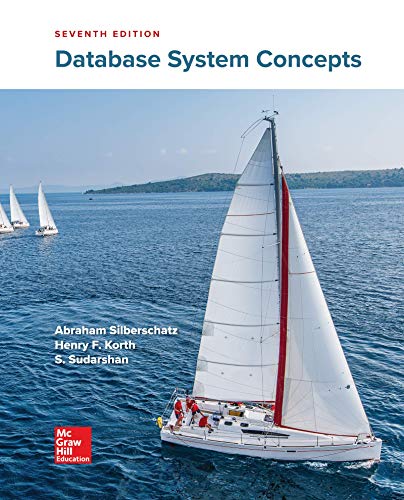
Write a code in Java programming
You are working in a game company that is developing a new RPG game. One of the core features of the game is the ability to create and manage different types of characters, such as warriors, mages, and archers.
However, your colleague, who was previously working on the implementation of the CharacterManager class, has been laid off due to excessive absences.
You have been assigned to take over the project and complete the implementation of the class based on the design specifications outlined below:
Given the following main method:
public static void main(String[] args) {
CharacterManager manager = CharacterManager.getInstance(); manager.createCharacter(CharacterType.WARRIOR).attack(); manager.createCharacter(CharacterType.MAGE).attack(); manager.createCharacter(CharacterType.ARCHER).attack();
}
Your output should be:
Warrior is attacking
Mage is attacking
Archer is attacking
Note that you should not change the method signature.
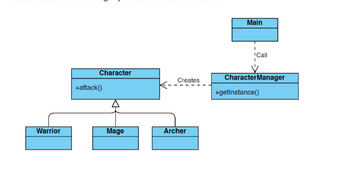

Step by stepSolved in 4 steps with 4 images

- I need help with completing my Java programming project. I will be using the software Eclipse for this project so if you could also guide me through the project like labeling what is supposed to be in whatever "4 java files" or what section is what so I'm not trying to guess around, that would be great. Thank youarrow_forward121. Developer often make implementation compromises in order to get a prototype working a. constantly b. slowly c. quickly d. randomlyarrow_forwardPlease do it Yourself.You are supposed to write an SRS document of the system scenario given in the file named “Smart health system”. In the file the information about the system and its module which are to be made are discussed. You are supposed to make an SRS document of that system by writing only the first three sections of the SRS document. The File: Read it Smart Health Monitoring SystemAs the name states Smart Health Monitoring System is a smart AI that calculates almost everything you need to take care of your health. The System helps with-BMI Level,-Diet Monitoring,-Diet Suggestions,-Footstep monitoring,-Sleep Suggestions,-Water Alarm. So the user has to just register into the system by giving his personal details and physical attributes and the rest, the system takes care of everything. The Food items with its fats, carbs and proteins level are already stored in the database and the user can also add new food, which will help the system to monitor the users diet and…arrow_forward
- Please write Java Code per the instructions and make sure code compiles before submitting. Thank you!arrow_forwardhello c++ programming Superhero battle time! Muscles and Bones are not happy with each other and need to come to some kind of understandingWhat are the odds that Muscles will come out ahead? What about Bones? Start with this runner file that will handle the simulation, and don't modify the file in any way (runner file is below)Spoiler time... When it's all said and done, Muscles should win about 72% of the time, and Bones about 28% Create files TwoAttackSupe.h and TwoAttackSupe.cpp that collectively contain the following functionality: The class name will be TwoAttackSupe The class will be a base class for what you see below in the next blurb The class has one member variable, an integer value that maintains a health value Its only constructor takes one parameter, which is the health value Make sure to set up a virtual destructor since this is a base class Develop a function called IsDefeated() which returns a bool Return true if the health value is less than or equal to 0 Return…arrow_forwardCreate pseudocode, flowchart and python code for the Patient Charges program. Thisfinal project requires multiple files (modules, drivers, and your main).Design a class named Patient that has fields for the following data:● First name, middle name, last name● Address, city, state, and ZIP code● Phone number● Name and phone number of emergency contactThe Patient class should have a constructor that accepts an argument for each field. ThePatient class should also have accessor and mutator methods for each field.Next, write a class named Procedure that represents a medical procedure that has beenperformed on a patient. The Procedure class should have fields for the following data:● Name of the procedure● Date of the procedure● Name of the practitioner who performed the procedure● Charges for the procedureThe Procedure class should have a constructor that accepts an argument for each field.The Procedure class should also have accessor and mutator methods for each field.Next, design a…arrow_forward
- URGENT In python You just started your summer internship with old jalopy auto Rentals based in Lenior. North Carolina. You will be working in a large development team in the process of building the firm's first online reservation system. your first assignment is to build the components that will check the age of the customer and then assign them a car. you will be building the business logic classes, so you do not have to worry about the forms of communicating with the user or data access classes to communicate with the database. To test your classes, you will use them in a test application. The requirements for your classes and the testing application are as follows: -You will design and build 2 components of the online reservation system(ORS). There will be one class to represent customers and another to represent vehicles. -Each class should have at least 2 members and 2 methods -The ORS will need to be able to check to make sure the customer in question is at least 25 years old. If…arrow_forwardTHIS NEEDS TO BE DONE IN C#!! The Tourtise and The Hair In this lab, we will be simulating the classic race of the tourtise and the hare. The race will take place on two paths of 70 tiles, which spans from left to right. The path will have two lanes, one for each animal. The path will be represented as an array of 70 characters, where each character will be the following: · A dash(i.e, “-“), which represents an empty tile. · An “h”, which represents the hare on the hare lane. · A “t”, which represents the tourtise on the tortise lane. All tiles will be “empty” (i.e. set to the dash) with the exception of the tiles that are occupied by the animals. As the animals move, the previous tile the animal was on will be set to empty, and the new tile will be changed to either “h” or “t”. Tile 70 will be the finishing tile, and the first animal to that tile will be declared the winner of the race. We will use randomization to determine how far each animal will move. Since this race will be…arrow_forwardIn this project, you will break the problem down into a set of requirements for your game program. Then you will design your game by creating a storyboard and pseudocode or flowcharts. Remember, in Project One, you are only designing the game. You will actually develop the code for your game in Project Two. Review the Sample Dragon Text Game Storyboard in the Supporting Materials section to see a sample storyboard for a dragon-themed game. You will begin by creating a storyboard to plan out your game. Using one of the templates located in the What to Submit section, write a short paragraph that describes the theme of your game by answering all of the following questions: What is your theme? What is the basic storyline? What rooms will you have? (Note: You need a minimum of eight.) What items will you have? (Note: You need a minimum of six.) Who is your villain? Next, you will complete your storyboard by designing a map that organizes the required elements of the game (rooms, items,…arrow_forward
- Overview In this assignment, you will gain more practice with designing a program. Specifically, you will create pseudocode for a higher/lower game. This will give you practice designing a more complex program and allow you to see more of the benefits that designing before coding can offer. The higher/lower game will combine different programming constructs that you have been learning about, such as input and output, decision branching, and a loop. Higher/Lower Game DescriptionYour friend Maria has come to you and said that she has been playing the higher/lower game with her three-year-old daughter Bella. Maria tells Bella that she is thinking of a number between 1 and 10, and then Bella tries to guess the number. When Bella guesses a number, Maria tells her whether the number she is thinking of is higher or lower or if Bella guessed it. The game continues until Bella guesses the right number. As much as Maria likes playing the game with Bella, Bella is very excited to play the game…arrow_forwardLanguage is C++ Lab14A: The Architect. Buildings can be built in many ways. Usually, the architect of the building draws up maps and schematics of a building specifying the building’s characteristics such as how tall it is, how many stories it has etc. Then the actual building itself is built based on the schematics (also known as blueprints). Now it is safe to assume that the actual building is based off the blueprint but is not the blueprint itself and vice versa. The idea of a classes and objects follows a similar ideology. The class file can be considered the blueprint and the object is the building following the analogy mentioned above. The class file contains the details of the object i.e., the object’s attributes (variables) and behavior (methods). Please keep in mind that a class is a template of an eventual object. Although the class has variables, these variables lack an assigned value since each object will have a unique value for that variable. Think of a form that you…arrow_forwardHi, this is a java programming question with direction of what to do. I'm just gonna attach the question sheet. Implement the following class diagram (diagram in attached photo)setFirstName() method should set the firstName attribute of the class.setLastName() method should set the lastName attribute of the class.setSpecialization() method should set the specialization attribute of the Doctor class.setHealthnumber() method should set the healthNumber attribute of the Patient class.setIllness() method should set the illness attribute of the Patient class.If you are not comfortable with set methods, you are free to create your own constructors to set the attribute values.Create a HospitalApplication class which contains the main method. Inside the main method the following should be done in the order mentioned. (Any other ordering would not guarantee full marks).Prompt for the following inputs for 5 Doctors. (Use for loops)• First name• Last name• SpecializationValid specializations…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
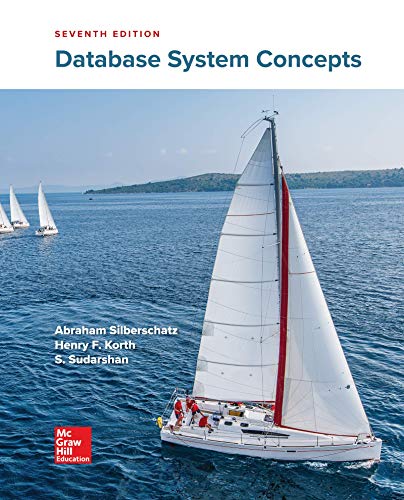
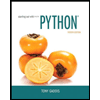
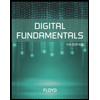
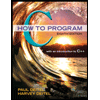
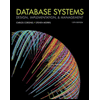
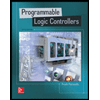