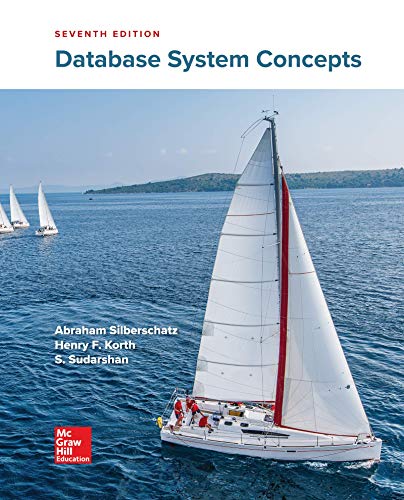
Write a function named “hasDashOnBothSides” that accepts parameters of the
same as command line arguments (argc and argv). It returns true if all of the
arguments have a '-' on both the beginning and ending of the arguments and false
otherwise. If it has no argument, it will return true.
In addition, this function also returns another boolean value of true when one of
the arguments is just a dash ('-') and false otherwise.
Please note that you cannot use string class, string methods such length() or
size() or any string function such as strlen(). Please use only array notation,
pointer to character, and/or pointer arithmetic and make use of the fact that this is
a C-string.
Please show how you would call and test this function. One simple way is to
accept command line arguments or the other is to simply hard-code values of the
array and call this function. Please print out the results of what this function
returns.
If there is no argument, it will return true and false
If the argument is just "-", it will return true and true
If the arguments are "-", "-help-" and "--", it will return true and true
If the arguments are "-help-" and "--", it will return true and false
If the arguments are "-help" and "-help-", it will return false and false.
If the argument is just "-h", it will return false and false.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Create a function called getHex() as follows: • This function should return a value of type char and have a parameter of type int. • The function should use either an iflelse or a switch to return the corresponding hex value of the number as a char value. o If the number is between 0 and 9, add '0' to the parameter. o If the number is between 10 and 15, a local char variable should be set equal to the correct letter ('A', 'B', 'C', 'D', 'E', or 'F').arrow_forwardWrite a full program that display the area of a rectangle. The program calls the following two functions: • getLengthWidth – This function uses a reference parameter variables to accept an int arguments length and width. This function should ask the user to enter the rectangle’s length and width. Input validation: length and width should be positive integers. • calArea – This function should accept the rectangle’s length and width as arguments and return the rectangle’s area. The area is calculated by multiplying the (length * width).arrow_forwardWrite a function named “HasTheSameEndingChar” that accepts 2parameters the same as command line arguments (argc and argv). It will return true if command line arguments that has the same ending character. If the user has provided no argument or only one argument, it will also return true. If any of the arguments does not end with same character, it will return false. This function should not have cin or cout. This function cannot use string class, either. Write a complete C++ program that accepts command line arguments and call the above function. It will print out “Yes” if the function returns true and “No” if it returns false. Important requirement: This program cannot include header file and cannot use string class and its methods such as “length” or “size” This program requires only the use of array, C-string and pointers No global variable is allowed to be declared and used For example, the following runs will print Yes, Yes, Yes, Yes, No, No, Noand…arrow_forward
- Write a function named “endingWithSlashSlash” that accepts the same parameters as command line arguments (argc and argv). It returns true if at least one of the arguments have a “//” at the end and false otherwise. This function also returns the number of arguments that have “//” at the end. For example, “//” will return true and 1 “one// two three// four” will return true and 2 “one” will return false and 0 “one two” will return false and 0 Please note that for question #5, you cannot refer or use string class, string methods such length() or size() or any string function such as strlen(), strcmp or strstr(). Please use only array notation, pointer to character, and/or pointer arithmetic and make use of the fact that this is a C-string.arrow_forwardExamples Input 8 Output How about visiting Gibraltar Rock? It is about 8 miles away. Input 30 Output How about visiting Oxnard? It is about 30 miles away. Input 120 Output You provided an invalid distance that is not between 0 and 100 miles. Input -100 Output You provided an invalid distance that is not between 0 and 100 miles. Hints • you can check the type of an input parameter by using the type () function.arrow_forwardDefine a function drawCircle. This function should expect a Turtle object, the coordinates of the circle’s center point, and the circle’s radius as arguments. The function should draw the specified circle. The algorithm should draw the circle’s circumference by turning 3 degrees and moving a given distance 120 times. Calculate the distance moved with the formula 2.0 × π × radius ÷ 120.0. Define a function main that will draw a circle with the following parameters when the program is run: X = 50 Y = 75 Radius = 100arrow_forward
- Here is the main function in a program int main () { int x = 30; //more code goes here someFunction (x) ; cout « x; return 0; } Assume the heading for someFunction is written correctly with an integer parameter named x, and that this is the body of that function: { x = x * 20; //more code goes here } What will be the output? Just enter a number. If there would be an error, enter -999arrow_forwardQuestion: In C#, how can I recreate this into a Windows form Application instead of a console application? Please show a photo of the display and show the code that you used, thank you! Problem: Write a function Seperate( number ) that separates an integer number (ranging from 0 to 99999) into its digits. For example, if the number is 42329, the function finds 4, 2, 3, 2, 9. If the number is 323, the function finds 0, 0, 3, 2, 3. (Hint: use modulus and integer division operations.) Code: using System;public class RecExercise4{static void Main(){Console.Write("Input any number (ranging from 0 to 99999) : ");int num = Convert.ToInt32(Console.ReadLine());Console.Write(" The digits in the number are : ");separateDigits(num);} static void separateDigits(int n){int i = 4;int[] arr;arr = new int[5];while (i>-1){arr[i] = n%10;n = n/10;i--;}foreach(int j in arr)Console.Write(" " + j);}}arrow_forwardWrite a function called factorial that computes the factorial of a given number x passed in as an argument. By default it returns the factorial of 6, which is 720. It also takes a optional boolean argument called 'to_print'. If to_print is True, the factorial value is printed instead of being returned. It is False by default, meaning the result is returned, not printed. >>> result = factorial()>>> print(result)Output: 720>>> result = factorial(to_print = True)Output: 720>>> print(result) Output: # no output>>> result = factorial(4)>>> print(result) Output: 24>>> factorial(4, to_print = True)Output: 24>>> print(result) Output:arrow_forward
- Add a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forwardIn C++ Write a function named find_bigfoot which takes a string as an argument, and then determines whether the string "bigfoot" is found in the parameter. Then it returns true or false, depending on whether it was found or not. For example: find_bigfoot("There is no yeti");find_bigfoot("footbig not here"); would both return false, since the string bigfoot isn't part of the string while: find_bigfoot("I believe in bigfoot");find_bigfoot("bigfoot is around here"); would both return true, since the string bigfoot is part of the string.arrow_forwardTask 2: The formula for the area, A, of a triangle with sides of length a, b, and c is V[s(s – a)(s – b)(s – c)] A = Where (a + b + c) s = Write, test, and execute a function that accepts the values of a, b, and c as parameters from a calling function, and then calculates the values of s and [s(s - a)(s - b)(s - c)]. If this quantity is positive, the function calculates A. If the quantity is negative, a, b, and c do not form a triangle, and the function should set A = -1. The value of A should be returned by the function. Test the function by passing various data to it and verifying the returned value.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
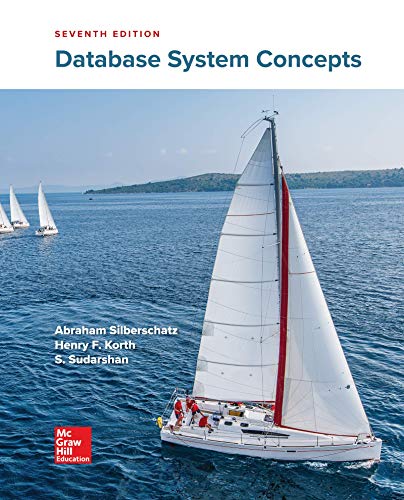
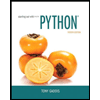
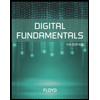
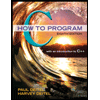
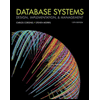
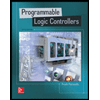