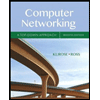
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a generic class Students.java which has a constructor that takes three parameters –
id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString()
method in this class will display these details for any student. A generic method score() will
be part of this class and it will be implemented by inherited classes. Write accessors and
mutators for all data points.
id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString()
method in this class will display these details for any student. A generic method score() will
be part of this class and it will be implemented by inherited classes. Write accessors and
mutators for all data points.
Write two classes RemoteStudents.java and InPersonStudents.java that inherits from
Student class. Show the use of constructor from parent class (mind you, RemoteStudents
have one additional parameter – discussion). Implement the abstract method score() of the
parent class to calculate the weighted score for both types of students.
Student class. Show the use of constructor from parent class (mind you, RemoteStudents
have one additional parameter – discussion). Implement the abstract method score() of the
parent class to calculate the weighted score for both types of students.
Write a driver class JavaProgramming.java which has the main method. Create one remote
student object and one in-person student object. The output should show prompts to enter
individual scores – midterm, finals, ...... etc. and the program will calculate weighted score
and display it. Format the output to display up to two decimal places.
individual scores – midterm, finals, ...... etc. and the program will calculate weighted score
and display it. Format the output to display up to two decimal places.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- in java Create a class for Student and implement all the below listed concepts in your class. Read lecture slides for reference. Class Name: Student 1. Data fields studentID name email majorDepartmentSelect proper datatypes for these variables. 2. Constructors – create at least 2 constructors No parameter With parametersSet id and name using the constructor with parameters. 3. Methods toString method : To print the details of the Student. Setter and getter methods 4. A static variable count : To keep the count of Student objects5. Visibility Modifiers: private for data fields and public for methods 6. Write some test cases in main methodarrow_forwardrod1 and rod2 are instances of the Length class. Attributes start_val and end_val of both rod1 and rod2 are read from input. In the Length class, define instance method compute_length() with self as the parameter to return the value of attributes end_val minus start_val.arrow_forwardThe Java Programming class has two types – remote and in-person. For remote students, the weighted score comprises of midterm, final, assignments and discussion. The weights for each are 30%, 30%, 30% and 10% respectively. For in-person students, the weighted score comprises of midterm, final and assignments. The weights for each are 30%, 30% and 40%. Write a generic class Students.java which has a constructor that takes three parameters – id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString() method in this class will display these details for any student. A generic method score() will be part of this class and it will be implemented by inherited classes. Write accessors and mutators for all data points. Write two classes RemoteStudents.java and InPersonStudents.java that inherits from Student class. Show the use of constructor from parent class (mind you, RemoteStudents have one additional parameter – discussion). Implement the abstract method…arrow_forward
- In Java Your uncle is trying to keep track of his new-car and used-car lots by writing a Java program. Heneeds your help in setting up his classes.Implement a superclass named Car that contains a price instance variable, a getPrice method, anda 1-parameter constructor. The getPrice method is a simple accessor method that returns the priceinstance variable’s value. The 1-parameter constructor receives a cost parameter and assigns avalue to the price instance variable based on this formula:price = cost * 2;Implement two classes named NewCar and UsedCar; they are both derived from the Carsuperclass. NewCar should contain a color instance variable (the car’s color). UsedCar shouldcontain a mileage instance variable (the car’s odometer reading). The NewCar and UsedCarclasses should each contain a 2-parameter constructor, an equals method, and a toString() method.In the interest of elegance and maintainability, don’t forget to have your subclass constructors callyour superclass constructors…arrow_forwardCreate a class called Student that models a student at Carleton University. A student's state consists of a name (String), id number (int) and a list of grades (array of double). Your class must have a constructor that has three input parameters (name, id and grade list) and sets the initial state for the object. Include a toString() method that returns a useful string representation of a student object. The string representation should contain the student's name, ID number, and the number of grades they have recorded (so NOT include all the grades). Include a gradesInRange(double lower, double upper) method that returns the number of grades that this student has that are in the range [lower,upper]. That is, the it returns the number of grades that are greater than or equal to lower and less than or equal to upper. Example Usage: double[] grades {81.2, 93.2, 76,2, 84.6, 63.11, 79.8}; Student s new Student("cat", 100123987, grades); s.gradesInRange (80,100); int num_grades_A // assert…arrow_forwardIn this java assignment, we will be creating a pay stub for an employee using classes. Each class must be implemented into their own file, a total of 5 classes/files. We are going to implement the following classes: Employee – This class represents an employee. It needs the following fields exposed via getters and setters: Employee ID (we will need to make this unique, for now, just hard code it to 1). First name, Last name, Middle initial Address, City, Zip Phone, Email Hourly Rate PayPeriod – This class represents an employee’s payment information. An employee will eventually have more than one pay period. It must contain the following fields (exposed via getters and setters): Pay period Id (hard code it for now) Employee Id (who is this for) Start Date, End Date Number of hours PayrollManager – This class provides the functionality we need to compute and display our payroll. It should implement the following methods: double CalculateGrossPay (Employee, Payperiod) – This…arrow_forward
- javaarrow_forwardThe goal of this coding exercise is to create two classes BookstoreBook and LibraryBook. Both classes have these attributes: author: Stringtiltle: Stringisbn : String- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like 20% off...etc). For a LibraryBook, we add the call number (that tells you where the book is in the library) as a string. The call number is automatically generated by the following procedure:The call number is a string with the format xx.yyy.c, where xx is the floor number that is randomly assigned (our library has 99 floors), yyy are the first three letters of the author’s name (we assume that all names are at least three letters long), and c is the last character of the isbn.- In each of the classes, add the setters, the getters, at least three constructors (of your choosing) and override the toString method (see samplerun…arrow_forwardDesign a class called Post. This class models a StackOverflow post. It should have properties for title, description and the date/time it was created. We should be able to up-vote or down-vote a post. We should also be able to see the current vote value. In the main method, create a post, up-vote and down-vote it a few times and then display the the current vote value.arrow_forward
- JAVA QUESTION REQUIRES SEPARATE CLASSES, CANNOT BE WRITTEN IN ONE MAIN METHOD E.G. ACCOUNT SECTION WRITTEN IN ACCOUNT CLASS, CUSTOMER SECTION WRITTEN IN CUSTOMER CLASS ETC. Phase 2 In this phase you’ll be writing code within the methods of your classes to make the tests pass. Account Each account should have a constant, unique id. Id numbers should start from 1000 and increment by 5. The ACCOUNT_ID attribute should be initialised when the account object is created. The id should be generated internally within the class, it should not be passed in as an argument. The deposit method should increase the balance by the value passed in as an argument. The withdraw method should reduce the balance by the value passed in as an argument. It should return the value of the argument The account should be able to go overdrawn The correctBalance method should change the balance to match the value passed in as an argument. Testing your code for Account Run TestAccountInitialisation to check…arrow_forwardWrite an interface and then modify classes to implement the interface. Write an interface, GeometricSolid, which has one method, volume. The volume method takes no arguments and returns a double. You are provided with three classes: Cylinder, Sphere, and RightCircularCone Modify the classes so that they implement the GeometricSolid interface. Supply the appropriate method for each class. Make sure that you use Math.PI in your calculations. Notice in InterfaceRunner that the objects are added to an ArrayList of GeometricSolids. Cylinder.java /*** Models a Cylinder*/public class Cylinder {private double radius;private double height; /*** Constructor for objects of class Cylinder* @param radius the radius of the Cylinder* @param height the height of this Cylinder*/public Cylinder(double radius, double height){this.radius = radius;this.height = height;}/*** Gets the radius of this Cylinder* @return the radius of the Cylinder*/public double getRadius(){return radius;}/*** Sets the radius of…arrow_forwardWrite a program in java that contain a class named office, data members include area and noOfEmployees. Two classes named regionalOffice and headQuarters are derived from office. regionalOffice contain managerName and location as data members while headQuarters contain CEO as data member. Data members of child class must be protected. Write input method, display method, a parametric constructor initializing all data members(both parent and child class). Use chaining in above all. Also define all accessor and mutator methodsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
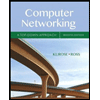
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
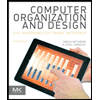
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
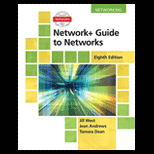
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
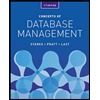
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
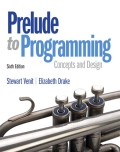
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
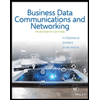
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY