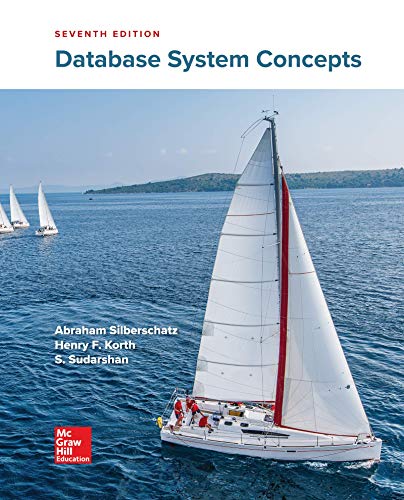
write a java program
A course has a name, a course number, a department code
(for example, CS, MATH) and a room number.
• Include a static variable used to assign the course number to each Course in the
constructor. The value of the variable will start at 1000.
• Write the instance variables, the accessors (getters), the mutators (setters) and two
constructors for the class. One of the constructors should be the no args constructor
Write a second class, XXX_ TestCourse (XXX is your Kean Id) ,which will have a
main method.
• Create an Array of Course objects, which can hold 3 elements.
• Using a loop:
• Ask the user for the name, department and room number for 3 courses.
• Create course objects.
• Store the objects in the Array
• At the end, traverse the array of courses and print each course on a separate line

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In python please! Define the Artist class in Artist.py with a constructor to initialize an artist's information. The constructor should by default initialize the artist's name to "None" and the years of birth and death to 0. Define the Artwork class in Artwork.py with a constructor to initialize an artwork's information. The constructor should by default initialize the title to "None", the year created to 0, and the artist to use the Artist default constructor parameter values. Add an import statement to import the Artist class. Add import statements to main.py to import the Artist and Artwork classes. Ex: If the input is: Pablo Picasso 1881 1973 Three Musicians 1921 the output is: Artist: Pablo Picasso (1881-1973) Title: Three Musicians, 1921 If the input is: Brice Marden 1938 -1 Distant Muses 2000 the output is: Artist: Brice Marden, born 1938 Title: Distant Muses, 2000arrow_forwardA class called Author (as shown in the class diagram) is designed to model a book's author. It contains: Three private instance variables: name (String), email (String), and gender (char of either 'm' or 'f'); One constructor to initialize the name, email and gender with the given values; public Author (String name, String email, char gender) {......} (There is no default constructor for Author, as there are no defaults for name, email and gender.) public getters/setters: getName(), getEmail(), setEmail(), and getGender();(There are no setters for name and gender, as these attributes cannot be changed.) A toString() method that returns string representation of author. A class called Book is designed (as shown in the class diagram) to model a book written by one author. It contains: Four private instance variables: name (String), author (of the class Author you have just created, assume that a book has one and only one author), price (double), and qty (int); Two constructors:…arrow_forwardThis class is used to store a time of day and output it.It also stores the current time of day and manipulates it. class Time{ // instance variables for an object to store a time of day: private int hours; private int minutes; // static Time object to store the current time of day, // defaults to midnight: private static Time curTime = new Time(); // Default Constructor // initialize time to 00:00 (midnight) public Time() { hours = minutes = 0; } // Constructor to parse a string hh:mm as the Time public Time(String hourColonMinute) { int colonIdx = hourColonMinute.indexOf(':'); hours = Integer.parseInt(hourColonMinute.substring(0, colonIdx)); minutes = Integer.parseInt(hourColonMinute.substring(colonIdx+1)); normalize(); } // initialize time using parameters for hour and minute public Time(int hr, int min) { hours = hr; minutes = min; normalize(); } // set current time to parameters public static void setCurTime(int hr, int min) {…arrow_forward
- Write in Javaarrow_forwardInternally your program should have two Java classes: ValidatorNumeric (superclass) ValidatorString (subclass) The subclass ValidatorString extends ValidatorNumeric class, forming a hierarchy of two classes. Add a separate main class with the main() method to test all public methods in both ValidatorNumeric and ValidatorString classes. The main() method should create the necessary objects and run the user interaction as shown above. The ValidatorNumeric class is responsible for capturing user prompt and a range of valid numeric inputs. Its methods display the prompt and accept input from the user. It should provide the following public interface: // Default constructor ValidatorNumeric() // Specific constructor taking params as follows: ValidatorNumeric( String prompt, int min, int max ) // Another specific constructor taking params as follows: ValidatorNumeric( String prompt, double min, double max ) // Method that shows user prompt and gets an int from the user: public…arrow_forwardAssignment: Write the class XXXX_Worker with constructors, accessors, mutuators, and a toString method. A Worker has a Worker Name and Number. Write the class XXXX_ProductionWorker which is a subclass of Worker. The production worker has a shift number (values: 1 or 2) and an Hourly pay rate. A shift number of 1 means the day shift and 2 means the night shift. Write the class XXXX_ShiftSupervisor which is a subclass of Worker. The shift supervisor is a salaried worker who supervises a shift. The shift supervisor has a yearly bonus field. The yearly bonus is earned at year end based on performance. Write a class, XXXX_TestWorker, which does the following: Creates one Shift Supervisor object from information entered by the user. Creates an Array of Production Workers that can hold 3 objects. Itcreates3ProductionWorkerobjectsfrominformationenteredbytheuser Prints the information about each object in the format shown below using the toString methods of the…arrow_forward
- Write a code for a banking program.a) In this question, first, you need to create a Customer class, this class should have:• 2 private attributes: name (String) and balance (double)• Parametrized constructor to initialize the attributes• Methods:i. public String toString() that gives back the name and balanceii. public void addPercentage; this method will take a percentage value andadd it to the balanceb) Second, you will create a driver class and ask the user to enter 6 customers’ informationand then you will create an array of Customer objects.c) Then you use this array used for various operations as shown in the output.• Using the array of customer objects, you need to search for all customers whohave less than $150• Using the array of customer objects, you need to get the average balance of thebalances in this array• Using the array of customer objects, you need to get the customer with thehighest balance and lowest balance• Using the array of customer objects, you need to show all…arrow_forwardWrite a class named Commission with the following features: O It extends the Hourly class. O It has two instance variables (in addition to those inherited): one is the total sales the employee has made (type double) and the second is the commission rate for the employee (the commission rate will be type double and will represent the percent (in decimal form) commission the employee earns on sales (so .2 would mean the employee earns 20% commission on sales)). O The constructor takes 6 parameters: the first 5 are the same as for Hourly (name, address, phone mumber, social security number, hourly pay rate) and the 6th is the commission rate for the employee. The constructor should call the constructor of the parent class with the first 5 parameters then use the 6th to set the commission rate. O One additional method is needed: public void addSales (double totalSales) that adds the parameter to the instance variable representing total sales. O The pay method must call the pay method of…arrow_forwardJava:arrow_forward
- Java Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to…arrow_forwardNo written by hand solution Must be coded in Java.arrow_forwardDesign a console program that will print details of DVDs available to buy. Make use of an abstract class DVD with variables that will store the title, yearReleased, running time and price of a DVD. Create a constructor that accepts the title, yearReleased and running time through parameters and assign these to the class variables. Create an abstract set method for the price of a DVD; also create get methods for the variables.Create a subclass InstructionalDVD that extends the DVD class and implements an iPrintable interface. The interface that must be added is shown below:public interface iPrintable { String ShowDetails();}The InstructionalDVD subclass has a private variable named category for which a get method must be written. The constructor of the InstructionalDVD class must accept the title, yearReleased, running time and category through parameters. Write the code for the setPrice() and ShowDetails() methods.Write a useDVD class and instantiate 2 objects of the InstructionalDVD…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
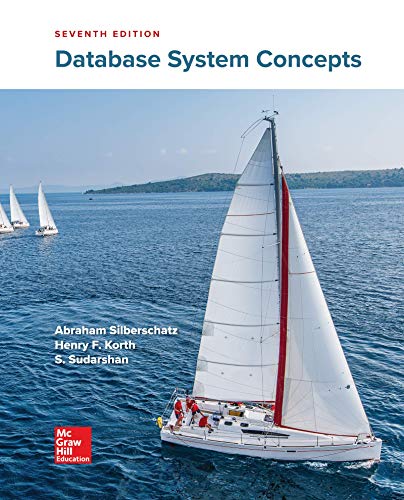
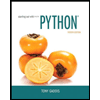
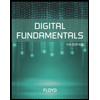
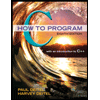
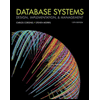
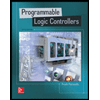