• Write a Java program that makes use of two-dimensional array. • Use if-else or switch-case statements. • Apply the corresponding looping statement. Program Specification: 1. Use two-dimensional array with size 7 columns and 5 rows. 2. Seat numbers are populated during run-time and not hard-coded. 3. User is asked to input a seat number. 4. The chosen seat number is replaced by the letter X. 5. Program displays a remark "Seat successfully reserved" when reservation is done. 6. The user is not allowed to reserve a previously reserved seat. Display "Seat is taken" remarks. 7. The user is not allowed to enter an invalid seat number. Display an error message. 8. The program continuously loops. Sample Output: 1 2 8 3 4 6 7 9 15 10 17 24 31 11 18 25 12 19 26 33 13 20 27 14 21 28 16 23 30 22 29 32 34 35 Enter seat number to reserve: 11 1 2 4 5 6 7 14 8 9 15 16 10 17 12 19 13 20 18 21 22 29 23 30 24 31 25 32 26 33 27 34 28 35 Enter seat number to reserve:
Instruction: Create a Java
Use if-else or switch-case statements.
Apply the corresponding looping statement.
Program Specification:
1. Use two-dimensional array with size 7 columns and 5 rows.
2. Seat numbers are populated during run-time and not hard-coded.
3. User is asked to input a seat number.
4. The chosen seat number is replaced by the letter X.
5. Program displays a remark “Seat successfully reserved” when reservation is done.
6. The user is not allowed to reserve a previously reserved seat. Display “Seat is
taken” remarks.
7. The user is not allowed to enter an invalid seat number. Display an error
message.
8. The program continuously loops.
Sample Output:
1 2 3 4 5 6 7
8 9 10 11 12 13 14
15 16 17 18 19 20 21
22 23 24 25 26 27 28
29 30 31 32 33 34 35
Enter seat number to reserve: 11
1 2 3 4 5 6 7
8 9 10 X 12 13 14
15 16 17 18 19 20 21
22 23 24 25 26 27 28
29 30 31 32 33 34 35
Enter seat number to reserve:
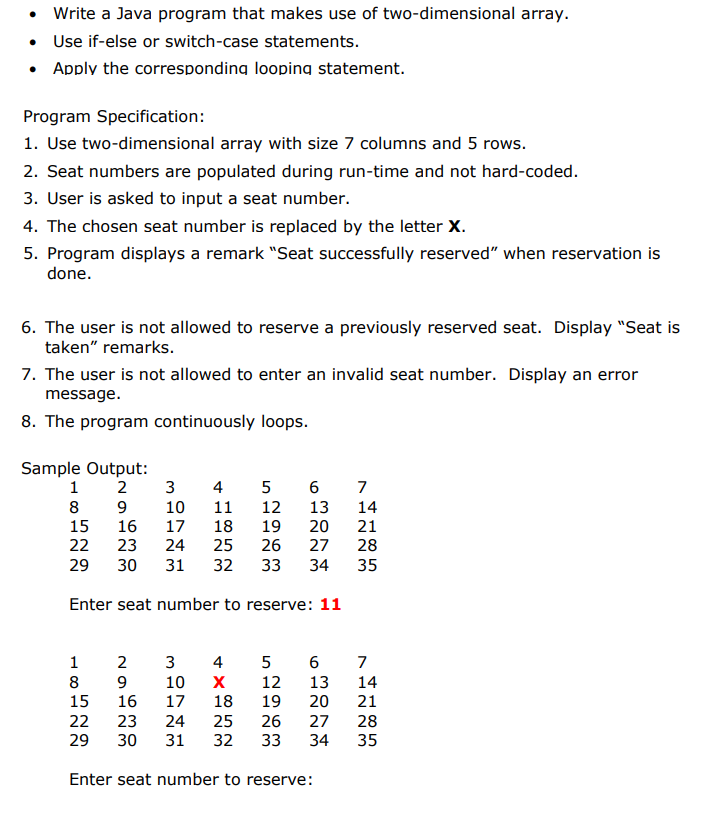

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

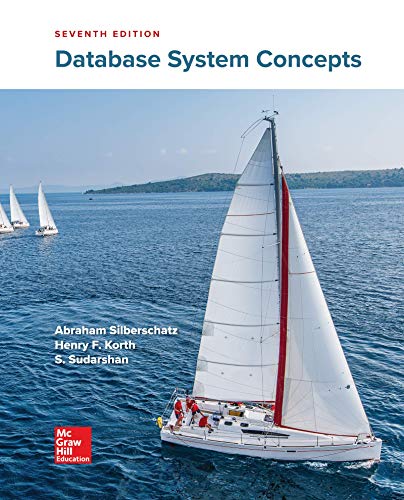
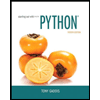
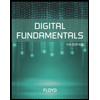
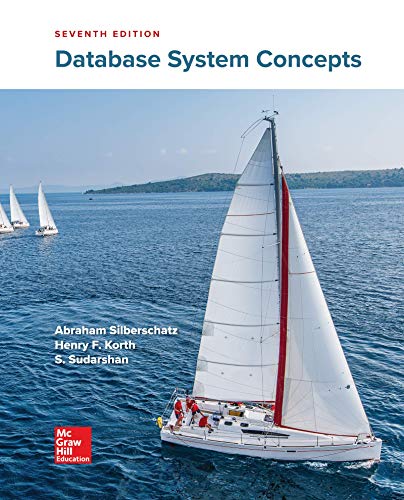
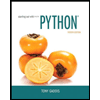
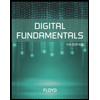
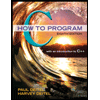
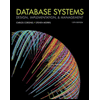
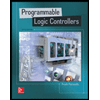