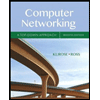
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a javascript program with explanations
- Use Bootstrap to style the table.
- The trip data needs to begin as an array literal of object literals, where each trip is an object with 3 properties.
- The button must have an id property, and using getElementById('id') you must assign the function that displays the table to the button using an anonymous function in your JavaScript code.
- Rather than use document.write(), you should concatenate the table elements as shown in the examples in the notes for JavaScript objects. If you are ambitious, though, you can get a 10% bonuse if you successfully use createElement() and appendChild(), as discussed in the DOM lecture notes offered later in the course. It is not necessary to use createElement(); concatenation is fine.
- Before being inserted into a table cell, the mpg must be calculated and not be part of the data objects.
- round the calculation to 1 decimal place
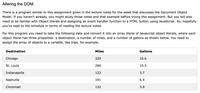
Transcribed Image Text:Altering the DOM.
There is a program similar to this assignment given in the lecture notes for the week that discusses the Document Object
Model. If you haven't already, you might study those notes and that example before trying this assignment. But you will also
need to be familar with Object literals and assigning an event handler function to a HTML button using JavaScript. So, hopefully
you've kept to the schedule in terms of reading the lecture notes.
For this program you need to take the following data and convert it into an array literal of Javascript object literals, where each
object literal has three properties: a destination, a number of miles, and a number of gallons as shown below. You need to
assign the array of objects to a variable, like trips, for example.
Destination
Miles
Gallons
Chicago
329
10.6
St. Louis
284
10.5
Indianapolis
122
3.7
Nashville
191
6.4
Cincinnati
132
3.9
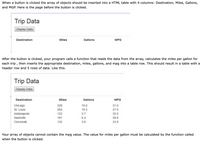
Transcribed Image Text:When a button is clicked the array of objects should be inserted into a HTML table with 4 columns: Destination, Miles, Gallons,
and MGP. Here is the page before the button is clicked.
Trip Data
Display Data
Destination
MIlles
Gallons
MPG
After the button is clicked, your program calls a function that reads the data from the array, calculates the miles per gallon for
each trip , then inserts the appropriate destination, miles, gallons, and mpg into a table row. This should result in a table with a
header row and 5 rows of data. Like this.
Trip Data
Display Data
Destination
Mlles
Gallons
MPG
Chicago
329
10.6
31.0
St. Louis
284
10.5
27.0
Indianapolis
122
3.7
33.0
Nashville
191
6.4
29.8
Cincinnati
132
3.9
33.8
Your array of objects cannot contain the mpg value. The value for miles per gallon must be calculated by the function called
when the button is clicked.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- Develop a menu-based shape-maker, using randomly generated sizes & characters: pyramid, inverted pyramid, diamond, and hourglass. Use functions to perform tasks, including separate functions for generating a random integer in a range and for generating a random character in a range. The four different shapes are made from a randomly generated printable character and a randomly generated maximum width between 4 and 12. Beneath the shape, print a descriptive caption stating the symbol and the maximum width.arrow_forwardRegister the textSize event handler to handle focus changes for the input tag. Note: The function counts the number of characters in the input. HTML JavaScript 1 let inputElement = document.getElementById("userName"); 2 3 function textSize(event) { document.getElementById("stringLength").innerHTML = event.target.value.length; 5 } 4 7 /* Your solution goes here */ 8 inputElement.addEventListenerC"text", stringLength); 2 Check Try againarrow_forwardA student report this problem when using HTML and JavaScript "On the assignment with preloaded images, I had to rewrite the example from the book. I had a problem with the syntax for linking the source file to the <form> element. I solved the problem by using this syntax document images img3.src = (source of file)." What kind of error is it? What rules were applied, and which should have been applied earlier?arrow_forward
- HTML/CSS JAVASCRIPT please help me answer this question I will give you a good rating Thank you! Given the following word search application, which searches for words in paragraphs, modify it so that it Adds two spans tab within the HTML within the designated new "Illustate" text segments as shown below. Has a new click event which searches the occurrence of the word within all span tabs residing within the first div container , then highlights the text in the span tab which contains the word c) In the same routine, count the number of times ( using a global variable) that the word is found Within the object array loop for span tabs ( where the indexOf statement is located) and after all span tabs are highlighted, send that number to a new html element ( a div, h1, span, p choice is up to you). The count should be continually accumulated and should show the total number of times a word is found in a span tab for all user activated clicks of the search button for…arrow_forwardWrite a for-loop that will write to the HTML document the value of each element in the array called myArray. Note: You only need to write a for-loop. Code on the outside of the for-loop is not necessary as we will assume that myArray has been declared and initialized with a series of array elements.arrow_forwardWrite a function named copyInput that that doesn't take any parameters and doesn't return a value. This function should find an HTML element with an id of "user_input". That element will be a text box (input element). Update the division (div element) with an id of "update_me" so that the div displays the text box's value..arrow_forward
- please use letter Marrow_forwardHi, can you help me with the indentation in this code, please. import csv# Define global variablesstudent_fields = ['ID', 'name', 'courses', 'absences', 'm1', 'm2', 'm3', 'total']student_database = 'students.csv'def display_menu():print("--------------------------------------")print(" Welcome to Student Management System")print("---------------------------------------")print("1. Add New Student")print("2. View Students")print("3. Search Student by iD")print("4. Search Student by courses")print("5. Delete Student")print("6. Quit")def add_student():print("-------------------------")print("Add Student Information")print("-------------------------")global student_fieldsglobal student_databasestudent_data = []for field in student_fields:value = input("Enter " + field + ": ")student_data.append(value)with open(student_database, "a", encoding="utf-8") as f:writer = csv.writer(f)writer.writerows([student_data])print("Data saved successfully")input("Press any key to continue")returndef…arrow_forwardI'm trying to learn CSS and it isn't easy. Can someone help me with this problem? Modify or add one CSS rule for each requirement below to change the text color property as specified. By color name: change the text color for byname class elements from black to blue. By RGB values: change the text color for byrgb class elements from black (rgb(0, 0, 0)) to green by modifying the second number to be 255. By HSL values: change the text color for byhsl class elements from black (hsl(0, 0%, 0%)) to cyan by modifying the first number to be 200, the second number to 100%, and the third number to 50%. Code: <p class="byname">The text is blue</p> <p class="byrgb">The text is green</p> <p class="byhsl">The text is cyan</p>arrow_forward
- Hi Please write this program using Javascript. Please attach or share a screenshot of the javascript 2. Create an empty <div> element with an id of your name. This element should be used to display your output from JavaScript. 3. Create a JavaScript file with your studentid.js and link it to your HTML file created above. Perform the following exercise in your JavaScript file: a. Declare an empty array that will be used to store integer numbers. b. Write a loop that iterates 30 times. In each iteration of the loop, generate a new random number between 0-50 and insert the value in your array (created above). c. After exiting your loop, display the content of your array in an h2 element inside the div element created in your HTML document, where array elements are separated by the double quote ”. d. Loop through your array and count the instances of numbers 7 and 9 found in your array. As you are looping through the array, also replace each instance of number 3 with your first…arrow_forwardPlease help for each proble stey by step and with a final code for understanding thank you.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
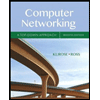
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
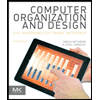
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
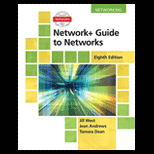
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
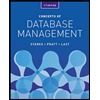
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
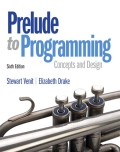
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
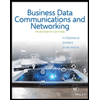
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY