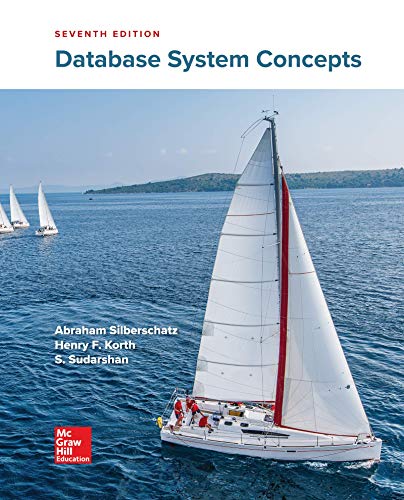
Write a method sameVowelsStrings that takes three Strings as input. The method returns true if the three Strings contains the same unique vowels, false otherwise. Note that vowels are 'a', 'e', 'i', 'o' and 'u'.
For example:
• sameVowelsString("apple", "cattle", "accept") returns true. All strings have a and e as their vowels.
• sameVowelsStrings("apple", "banana", "assume") returns false. The second string is missing the vowel e.
The first and second strings are missing the vowel u
• sameVowelsStrings("apple", "orange", "recordable" ) returns false. The first string is missing the vowel o.
• sameVowelsStrings("e", "ee", "eee" ) returns true. They all have vowel e.
Subject: Java

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Determine whether each statement below is true or false. Assume no index is out of bounds. Write either "true" or "false" on each line. Note: The Math.random() method returns a pseudorandom double type number greater than or equal to 0.0 and less than 1.0. int[] a = new int[6]; int[] b = new int[6]; int[] c = a; c[0] = 3;arrow_forwardWrite a method named sumOfStarts, which takes two Stringparameters named s1 and s2 and returns an int. The methodshould do the following:1. Return the sum of all the starting indices where s1 canbe found in s2.2. For example, if s1 is "goo" and s2 is "agoodegoodgo" thenthe method would sum 1 + 6 = 7.3. If s1 or s2 is null, or s1 cannot be found in s2, throw anIllegalArgumentException with the message "Null or notfound".4. You can assume any needed imports have already beenprovided.arrow_forwardYou're given a string of words. You need to find the word "Nemo", and return a string like this: "I found Nemo at [the order of the word you find Nemo]!". If you can't find Nemo, return "I can't find Nemo :(". Examples findNemo ("I am finding Nemo !") "I found Nemo a findNemo ("Nemo is me") → "I found Nemo at 1!" findNemo ("I Nemo am") → "I found Nemo at 2!" Ctarrow_forward
- Java Method called reverse that reverses a string passed to this method as a parameter and returns the reversed string. You should use for loop. You should not use external methods. E.g. reverse("string") should return gnirts. class Main {public static void main(String[] args) {}}arrow_forwardWrite a Java method that takes a variable of type String and returns a boolean value. The method returns true if the taken string contains a valid password, false otherwise. A valid password should satisfy all the following conditions: • It can contain any character • It contains at least 6 but not more than 20 characters • The number of letters (both uppercase and lowercase) is equal to the number of digits The method's header is as follows. public static boolean password (String s) Sample run Result String s = "Hello12345"; password(s); String s = "hellohil234567"; password(s); "hi21"; password(s); true boolean b = true boolean b = String s = boolean b = false Paragraph B.arrow_forwardImplement a new concat method in the String class you worked on for HW2. The method should return a new String object that represents the text you would end up with if the argument String were "added onto the end of" this String. For example, in the code below, the String object answer should represent the text "bookstore" and true should be printed the screen. char[] bookA = {'b', 'o', 'o', 'k'}; char[] storeA = {'s', 't', 'o', 'r', 'e'}; char[] bookstoreA = {'b', 'o', 'o', 'k', 's', 't', 'o', 'r', 'e'}; String book = new String(bookA); String store = new String(storeA); String bookstore = new String(bookstoreA); String answer = book.concat(store); System.out.println(answer.equals(bookstore)); In the text area below, type in your definition of the concat method. (In other words, type in the code that should replace the //TODO comment.) Your code cannot call any methods. public class String { private char[] data; public String(char[] value) { data = new…arrow_forward
- creat a method in (java) that do the following: This will prompt the user for initial list of letters(a word).Then it will generate all permutations of these letters .Here it has to be mentioned that the permutations can also be of shorter length than the list of letters . For example, if the initial list of letters is "STOP", then "TOP" and "TOPS" are both valid permutations, but "STOPS" isn'tarrow_forwardWrite a method myConcat that takes a string of multiple words and it returns a string made up of all the words concatenated together with the whitespace removed.arrow_forwardThe method printGroupSize() has an integer parameter. Define a second printGroupSize() method that has a string parameter. The second method outputs the following in order, all on one line: "A group of " the value of the string parameter "." End with a newline. Ex: If the input is 7 seven, then the output is: Order for size: 7 A group of seven. 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 public class GroupSize { publicstaticvoidprintGroupSize(intgroupSize) { System.out.println("Order for size: "+groupSize); } /* Your code goes here */ publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intsizeOfGroup; StringsizeInWord; sizeOfGroup=scnr.nextInt(); sizeInWord=scnr.next(); printGroupSize(sizeOfGroup); printGroupSize(sizeInWord); } }arrow_forward
- Write a method to replace all spaces in a string with '%20'. You may assume that the stringhas sufficient space at the end to hold the additional characters, and that you are given the "true"length of the string. (Note: If implementing in Java, please use a character array so that you canperform this operation in place.)EXAMPLEInput: "Mr John Smith ", 13Output: "Mr%20John%20Smith"arrow_forwardWrite a method called subLR take a String number and return an integer that is the subtraction of left part and right part of the numbers. If the number has odd number of digits, then we ignore the middle one. Examples: • subLR ("123456") should return -333. Because left part is 123 and right part is 456, thus 123 - 456 = -333 • subLR ("92834") should return 58. Because left part is 92 and right part is 34 (we ignore 8 because of odd number of digits), thus 92 - 34 = 58 • subLR ("-123") should return -4. Because left part is -1 and right part is 3 (we ignore 2 because of odd number of digits), thus -1 - 3 = -4 subLR ("-345020") should return -365. Because left part is -345 and right part is 020, thus -345 - 20 = -365arrow_forwardDefine a method printStateInfo() that takes two string parameters and outputs as follows, ending with a newline. The method should not return any value. Ex: If the input is MA Massachusetts, then the output is: ++ MA ++ is Massachusetts's state codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
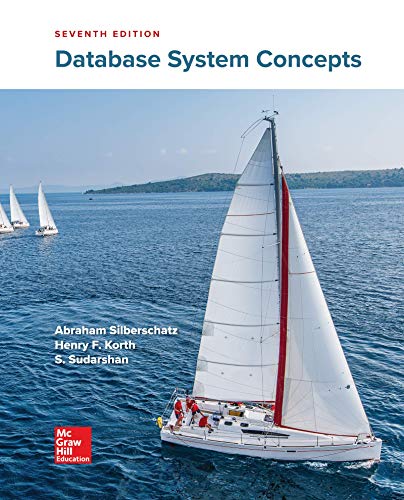
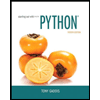
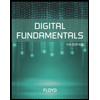
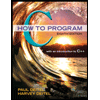
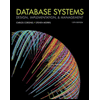
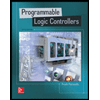