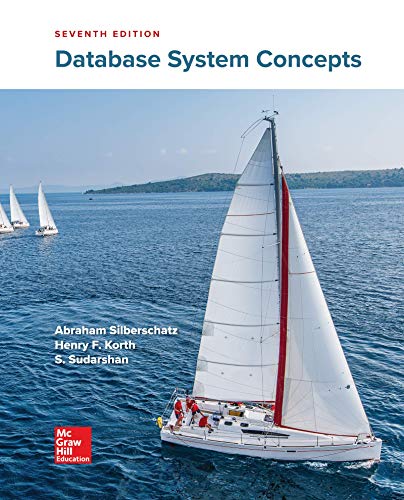
Write a
The numbers function generates 18 random integers, it is required you use the random.randint method to generate these numbers as the unitTest requires this. The range would be 7 to 61 inclusive, (duplicates are okay), and prints them all on one line separated by spaces.
A loop is required for this, and the loop should also total up the integers so the sum can be displayed when the loop ends.
The main() function calls the numbers() function which prints the random numbers and the total as shown below. Do not forget that numbers() function must return variable containing total that was printed.
This is the Python code I have tried ...( I have attached my error at the bottom... )
import random
def number():
total: int = 0
num: list = []
for i in range(18):
num = random.randint(7, 61)
print(num, end=" ")
total = total + num
print("")
print("The total is", total)
return total
def main(): # Alternatively if __name__ == '__main__': can be used to begin execution at main.
number()
main()
This is the ERROR i get when I test it: Please help- Thank you.
Test 1: stimulus:: 0 expected: '7 61 12 56 13 55 14 54 15 53 16 52 17 51 18 50 19 49 ', 'The total is 612' Test 1 Passed.
Test 2 Access numbers function directly
Test 2: stimulus:: expected: 612 Test 2 FailedE
======================================================================
ERROR: test_2_fn_case_1 (__main__.programTestCase)
----------------------------------------------------------------------
Traceback (most recent call last):
AttributeError: module 'program52' has no attribute 'numbers'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
ValueError: Program Output != Expected
----------------------------------------------------------------------
Ran 2 tests in 0.374s
FAILED (errors=1)
>>>

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- An automated donation machine accepts donations via 10 or 20 bills to a maximum of $990. The machine needs to print a receipt with the donation amount in English, rather than numerically. Complete the following function which converts any number between 10 to 990 dollars to English.NOTE: You may define and use another function if necessary.NOTE: You should use arrays for this task. Use of switch statements or very long if/else if statements for printing is not allowed. However, using if/else if/else statements for the logic of your algorithm is permitted.NOTE: This function returns the result, and does not print it. Make sure the result is not lost when you return. Example function input and output (donation is 110):Input: 110Output: “one hundred ten dollars” string convertToEnglish (int donation); in C++ #include <iostream>#include <string>using namespace std; string convertToEnglish (int donation); int main() {int donation;cin>>donation;…arrow_forwardWhat value will be returned by the function if a = 8, b=12? %3D int func(int a, int b){ if(a>b){ return a+b; } else if(aarrow_forwardComplete the code that returns the value of f(x), which has the value 1 inside the range −1 ≤ x ≤ 1 otherwise it is the value 0. f(x) [ ] # complete the function to return the value of 1, x = [-1, 1] 0, otherwise #f(x) given x. # return as the value called variable def function_f(x): # YOUR CODE HERE return fval "fval" ## Check your code below using print command ← → +0 0+arrow_forward
- In C++ i need the fill in the functions that will complete this code for the program to work.arrow_forwardPython code Screenshot and output is mustarrow_forwardNeed python help running code and others. Functions are ideal for use in menu-driven programs. When the user selects an item from a menu, the program can call the appropriate function. Write a menu-driven program for Burgers. Display the food menu to a user (Just show the 5 options' names and prices - No need to show the details!) Use numbers for the options and the number "6" to exit. If the user enters "6", your code should not ask any other questions, just show a message like "Thank you, hope to see you again!" Ask the user what he/she wants and how many of them. (Check the user inputs) Keep asking the user until he/she chooses the exit option. Use a data structure to save the quantities of the orders. You should use ArrayLists/Array Bags, or LinkedLists/LinkedBags (one or more of them) to save data. Calculate the price. Ask the user whether he/she is a student or a staff. There is no tax for students and a 9% tax for staff. Add the tax price to the total price.…arrow_forward
- in pythonarrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
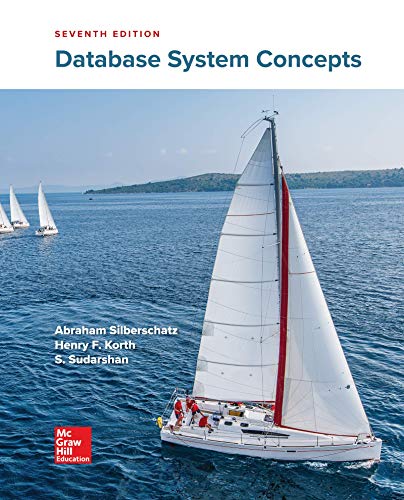
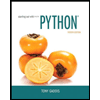
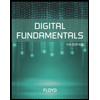
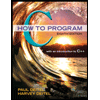
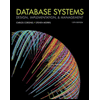
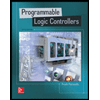