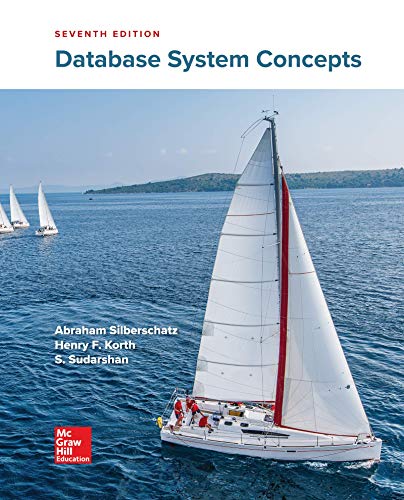
Write a
program should then output the number of lines in the file.test the program by creating a text file with at least 10 lines of text (of any composition). Use notepad++ to create the data file.
#include<stdio.h>
#include<stdlib.h>
//Structure
struct Person{
char name[10];
int age;
float wage;
};
//Main
int main (int argc, char **argv)
{
FILE* f;
if (argc != 2)
{
printf("No filename in the argument");
return 1;
}
f = fopen(argv[1], "r");
if (f == NULL) {
printf("The file cannot be opened successfully: %s\n",argv[1]);
return 1;
}
printf("The file has been opened successfully\n\n");
struct Person p[10];
int lines=0;
int i=0;
while( fscanf(f, "%s %d %f\n", p[i].name, &p[i].age, &p[i].wage) != EOF )
{
lines++;
i++;
}
i=0;
while(i<lines)
{
printf("Name: %s\t\Age: %d\t\Wage: %.2f\n", p[i].name, p[i].age ,p[i].wage);
i++;
}
printf("Number of Records: %d",lines);
fclose(f);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Write a program in the GO programming language that creates a CSV file to store and retrieve data such as name, age, and salary. You should be able to read the data out of the file and display the data sorted by name. Hint: Make use of the Sort package to sort the data. Make sure you check the existence of the file, closing the file when there is an error Use defer, panic, and recover as needed.arrow_forwardA photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Ex: If the input of the program is: ParkPhotos.txtand the contents of ParkPhotos.txt are:…arrow_forwardThe file disney_characters.csv contains a list of some Disney characters with their names, birthdays (in the format day/month) and franchise/production. The first few lines of the file look like this: Forename(s), Surname, Day of Birth, Production Grant Douglas, Ward, 7/1, Agents of S.H.I.E.L.D. Minnie, Mouse, 18/11, Mickey Mouse Michelle, Jones-Watson, 10/6,Spider-Man Riley Elphaba, Andersen, 22/1, Inside Out Della, Duck, 9/6, Donald Duck 1. Load the data into a pandas DataFrame and assign it to the variable disney_characters. 2. Define a second Data Frame second_half_characters that contains the rows of all characters whose first name starts with a letter from the second half of the alphabet. (You can assume that all first names start with a capital letter A-Z.) 3. Create a list of the full names of all characters whose birthday is in February and assign it to the variable list_by_birthday. The names should be in the format "Forename(s) Surname", e.g., "Donald Duck" or "Peter…arrow_forward
- each line in the input file will represent a record of data. There will be 10 lines, thus 10 records. Each record (line) will consist of 3 space separated fields: a name (1 word as a string), and age (as an integer), and a wage (as a float). Write a C program that: takes an input file name from the command line; opens that file if possible; declares a C struct with three variables; these will have data types that correspond to the data types read from the file (i.e. string, int, float); declares an array of C structs (i.e. the same struct type declared in point c); reads a record from the file and stores the values read into the appropriate struct variable at the appropriate array index (for that record); continues reading each record into a struct (as in point 1e), and stores each struct containing the 3 values into the array of structs declared in point d; closes the file when all records have been read. should print an error message in the event that the file cannot be…arrow_forwardThe international Olympics Committee has asked you to write a program to process their data and determine the medal winners for the pairs figure skating. You will be given a file named Pairs.txt.This file contains the data for each pair of skaters. The data consists of each skater’s name, their country and the score from each of eight judges on the technical aspects and on the performance aspects. A typical record in the file would be as follows: SmithJonesAustralia5.0 4.9 5.1 5.2 5.0 5.1 5.2 4.84.3 4.7 4.8 4.9 4.6 4.8 4.9 4.5 LennonMurrayEngland2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.83.1 3.2 3.3 3.4 3.5 3.6 3.7 3.8GustoPetitotItalia4.1 4.2 4.3 4.4 4.5 4.6 4.7 4.85.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8LahaiePetitFrance1.1 1.2 1.3 1.4 1.5 1.6 1.7 1.85.1 5.2 5.3 5.4 5.5 5.6 5.7 5.8BilodeauBernardCanada2.1 2.2 2.3 2.4 2.5 2.6 2.7 2.84.1 4.2 4.3 4.8 4.9 4.6 4.0 4.5LahorePedroMexico3.2 3.1 3.8 3.9 3.0 3.6 3.9 3.35.9 5.8 5.8 5.8 5.9 5.6 5.0 5.5MaliakKolikovRussia4.2 4.1 4.8 4.9 4.0 4.6 4.9 4.31.9 1.8 1.8 1.8…arrow_forwardRewrite the code in Figure 8-93 to read each line of a text file into an array named strFordModel. Do While objReader.Peek = -1 strFordModellintCount) = objReader.ReadLine() intCount +=1 Loop FIGURE 8-93arrow_forward
- write in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forwardUse Java programarrow_forwardWrite the code segments based on the given descriptions: Declare a file pointer called input. Open a file called location.txt for reading using this pointer. If the file is not available, display “File does not exist.”. The content of the text file location.txt is as shown below. It includes the location, latitude and longitude values. FILE location.txt CONTENTS <Location> <Latitude> <Longitude> UTM 1.5523763 103.63322 KLCC 3.153889 101.71333 UM 3.1185 101.665 UMS 6.0367 116.1186 UNIMAS 1.465174 110.4270601 Ask the user to enter a location. Check if the user’s location can be found in the text file. If found, display the location, latitude and longitude as shown below. SAMPLE OUTPUT Enter a location: UM Location : UM Latitude : 3.1185 Longitude : 101.6650 Otherwise display “Sorry, location could not be found”. SAMPLE OUTPUT Enter a location : MMU Sorry, location could not be foundarrow_forward
- Computer Science Please use go language programma to write this code. Write a program that creates a CSV file to store and retrieve data such as name, age, and salary. You should be able to read the data out of the file and display the data sorted by name. Hint: Make use of the Sort package to sort the data. Make sure you check the existence of the file, closing the file when there is an error Use defer, panic, and recover as needed.arrow_forwardjust do the first step. and the program should be in python and includ as many different functions as possible .arrow_forwardRewrite the code in Figure 8-93 to read each line of a text file into an array named strFordModel.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
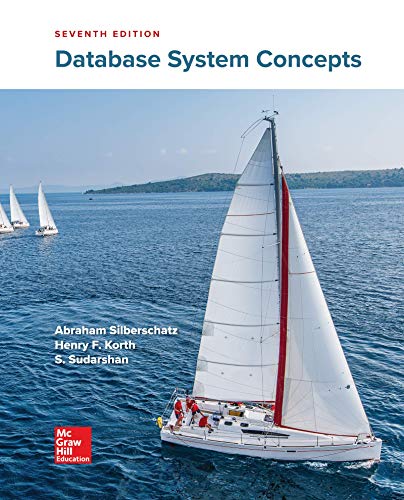
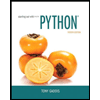
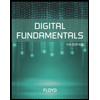
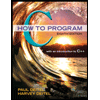
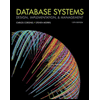
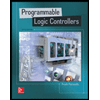