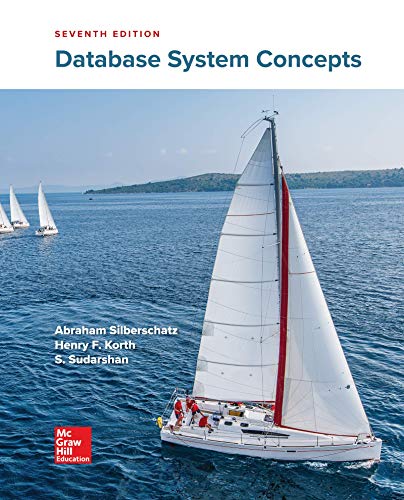
Concept explainers
Write a program that first reads in the name of an input file, followed by two strings representing the lower and upper bounds of a search range. The file should be read using the file.readlines() method. The input file contains a list of alphabetical, ten-letter strings, each on a separate line. Your program should output all strings from the list that are within that range (inclusive of the bounds).
Ex: If the input is:
input1.txt
ammoniated
millennium
and the contents of input1.txt are:
aspiration
classified
federation
graduation
millennium
philosophy
quadratics
transcript
wilderness
zoologists
the output is:
aspiration
classified
federation
graduation
millennium
Notes:
There is a newline at the end of the output.
All input files are hosted in the zyLab and file names can be directly referred to. input1.txt is available to download so that the contents of the file can be seen.
In the tests, the first word input always comes alphabetically before the second word input.
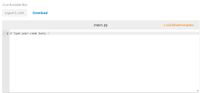

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- In this project, you will develop algorithms that find road routes through the bridges to travel between islands. The input is a text file containing data about the given map. Each file begins with the number of rows and columns in the map considered as maximum latitudes and maximum longitudes respectively on the map. The character "X" in the file represents the water that means if a cell contains "X" then the traveler is not allowed to occupy that cell as this car is not drivable on water. The character "0" in the file represents the road connected island. That means if a cell contains "0" then the traveler is allowed to occupy that cell as this car can drive on roads. The traveler starts at the island located at latitude = 0 and longitude = 0 (i.e., (0,0)) in the upper left corner, and the goal is to drive to the island located at (MaxLattitude-1, MaxLongitudes-1) in the lower right corner. A legal move from an island is to move left, right, up, or down to an immediately adjacent…arrow_forwardThe function print_last_line in python takes one parameter, fname, the name of a text file. The function should open the file for reading, read the lines of the file until it reaches the end, print out the last line in the file, report the number of lines contained in the file, and close the file. Hint: Use a for loop to iterate over the lines and accumulate the count. For example: Test Result print_last_line("wordlist1.txt") maudlin The file has 5 lines.arrow_forwardi have already asked this question and the answer did not work for me: Write a program that first reads in the name of an input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. Your program should output the words and their frequencies (the number of times each word appears in the file) without any duplicates. Ex: If the input is: input1.csv and the contents of input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy the output is: hello 1 cat 2 man 2 hey 2 dog 2 boy 2 Hello 1 woman 1 Cat 1 Note: There is a newline at the end of the output, and input1.csv is available to download.arrow_forward
- Hello, can someone help me with this assignment? I am trying to do it on Python language: Develop a program that first reads in the name of an input file, followed by two strings representing the lower and upper bounds of a search range. The file should be read using the file.readlines() method. The input file contains a list of alphabetical, ten-letter strings, each on a separate line. Your program should output all strings from the list that are within that range (inclusive of the bounds). Ex: If the input is: input1.txt ammoniated millennium and the contents of input1.txt are: aspiration classified federation…arrow_forwardPython please: Given the input file input1.csv write a program that first reads in the name of the input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. Your program should output the words in a sorted list. The contents of the input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy Example: If the input is input1.csv the output is: ['Cat', 'Hello', 'boy', 'boy', 'cat', 'cat', 'dog', 'dog', 'hello', 'hey', 'hey', 'man', 'man', 'woman'] Note: There is a newline at the end of the output.arrow_forwardcan you do it pythonarrow_forward
- Java Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardSuppose you are given a text file that contains the names of people. Every name in the file consists of a first name and last name. Unfortunately, the programmer that created the file of names did not guarantee that each name was on a single line of the file. Read this file of names and write them to a new text file sorted according to first name, one name per line. For example, if the input file contains Ed Marston Bob Jones Jeff Williams Fred Charles The output file should be Bob Jones Ed Marston Fred Charles Jeff Williams Use arrays to solve the problem.arrow_forwardCreate a list of 5 words, phrases and company names commonly found in phishing messages. Assign a point value to each based on your estimate of its likelihood to be in a phishing message (e.g., one point if it's something likely, two points if modereratly, or three points if highly likely). Write an application that scans a file of text for these terms and phrases. For each occurance of a keyword or phrase within the text file, add the assigned point value to the total points for the number of occurences and the point total. Show the point total for the entire message. (Write the program in Java)arrow_forward
- Write a program called p1.py that contains a function called filereader() that takes a number n (int) and a filename (string) as input. The function should read and store every nth line of the file in a list and return it.Test your function inside the main function (don’t forget the main guard).Sample output with “samplefile_t8.txt” file. It contains the following lines of text.kangaroo, 5capybara, 7wombat, 2koala, 3wallaby, 4quokka, 5platypus, 9dingo, 2kookaburra, 1>>> filereader(“samplefile_t8.txt”, 3) returns[“wombat, 2”, “quokka, 5”, “kookaburra, 1”]arrow_forwardJAVA PPROGRAM Write a program that prompts the user to enter a file name, then opens the file in text mode and reads names. The file contains one name on each line. The program then compares each name with the name that is at the end of the file in a symmetrical position. For example if the file contains 10 names, the name #1 is compared with name #10, name #2 is compared with name #9, and so on. If you find matches you should print the name and the line numbers where the match was found. While entering the file name, the program should allow the user to type quit to exit the program. If the file with a given name does not exist, then display a message and allow the user to re-enter the file name. The file may contain up to 100 names. You can use an array or ArrayList object of your choosing, however you can only have one array or ArrayList. Input validation: a) If the file does not exist, then you should display a message "File 'somefile.txt' is not found." and allow the…arrow_forwardThe next part of the program reads the file one line at a time and adds the scores, as a floating point numbers, to the list scores. scores = [] # empty list of scores for line in infile: line = line.strip() print (scores) # get score #convert to float # add to list of scores In the space below, complete the program fragment so that it prints the list of floating point scores as follows: [98.5, 97.4, 99.1, 97.2]arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
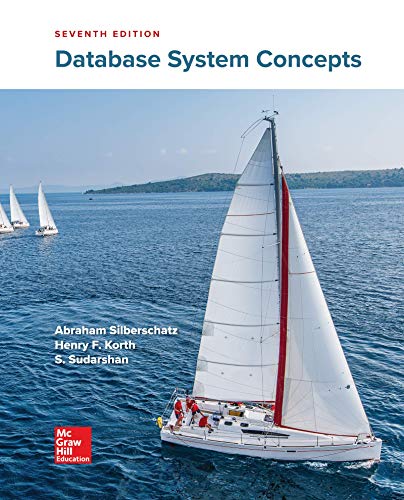
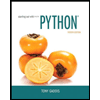
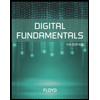
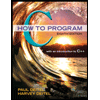
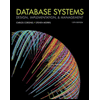
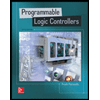