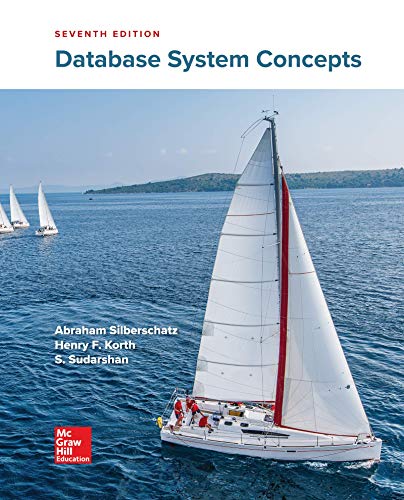
import java.util.Scanner;
public class LabProgram
{
static boolean IsArrayMult10(int inputVals[],int numVals)
{
for(int i=0;i<numVals;i++)
{
if(inputVals[i]%10!=0)
return false;
}
return true;
}
static boolean IsArrayNoMult10(int inputVals[],int numVals)
{
for(int i=0;i<numVals;i++)
{
if(inputVals[i]%10!=0)
return true;
}
return false;
}
public static void main(String[] args)
{
int n;
boolean f=false;
Scanner sc= new Scanner(System.in);
n=sc.nextInt();
int []arr=new int[20];
if(n<=20)
{
for(int i=0;i<n;i++)
{
arr[i]=sc.nextInt();
}
}
if(IsArrayMult10(arr,n)==true)
System.out.print("all multiples of 10\n");
if(IsArrayNoMult10(arr,n)==true)
{
for(int i=0;i<n;i++)
{
if(arr[i]%10==0)
{
f=true;
break;
}
}
if(f==true)
System.out.println("mixed values");
else
System.out.println("no multiples of 10");
}
}
}
![Write a program that reads a list of integers, and outputs whether the list contains all multiples of 10, no multiples of 10, or mixed values.
Define a method named isArrayMult10 that takes an array as a parameter, representing the list, and an integer as a parameter, representing
the size of the list. isArrayMult100) returns a boolean that represents whether the list contains all multiples of ten. Define a method named
İsArrayNoMult10 that takes an array as a parameter, representing the list, and an integer as a parameter, representing the size of the list.
İsArrayNoMult100 returns a boolean that represents whether the list contains no multiples of ten.
Then, write a main program that takes an integer, representing the size of the list, followed by the list values. The first integer is not in the
list. Assume that the list will always contain less than 20 integers.
Ex: If the input is:
5 20 40 60 80 100
the output is:
all multiples of 10
Ex: If the input is:
5 11 -32 53 -74 95
the output is:
no multiples of 10
Ex: If the input is:
5 10 25 30 40 55
the output is:
mixed values
The program must define and call the following two methods. isArrayMult10() returns true if all integers in the array are multiples of ten and
false otherwise. isArrayNoMult100) returns true if no integers in the array are multiples of ten and false otherwise.
public static boolean isArrayMult10 (int[] arrayValues, int arraySize)
public static boolean isArrayNoMult10(int[] arrayValues, int arraySize)](https://content.bartleby.com/qna-images/question/e6ce2240-b3fe-4331-98ee-3937416f40f5/52c265a8-8f36-47fb-a1a0-2fcd4835525c/fcexm44_thumbnail.png)
![1: Compare output a
2/2
Input
5 20 40 60 80 100
Your output
all multiples of 10
2: Compare output ^
2/2
Input
5 11 -32 53 -74 95
Your output
no multiples of 10
3: Compare output ^
2/2
Input
5 10 25 30 40 55
Your output
mixed values
4: Unit test ^
0/2
İsArrayMult10([10 200 4000)], 3)
Compilation failed
zyLabsUnitTest.java:13: error: cannot find symbol
studentResult =
studentMain.isArrayMult10 (myArray, 3);
Compilation failed
symbol:
method isArrayMult10 (int[],int)
location: variable studentMain of type LabProgram
1 error
5: Unit test ^
0/2
İsArrayNoMult10([9 11 13], 3)
Compilation failed
zyLabsUnitTest.java:13: error:
cannot find symbol
studentResult
studentMain.isArrayNoMult10 (myArray, 3);
Compilation failed
symbol:
method isArrayNoMult10 (int[],int)
location: variable studentMain of type LabProgram
1 error](https://content.bartleby.com/qna-images/question/e6ce2240-b3fe-4331-98ee-3937416f40f5/52c265a8-8f36-47fb-a1a0-2fcd4835525c/8wvu78b_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- JAVA programming languagearrow_forwardWrite code in Java: -Must be recursive import java.util.*; import java.lang.*; import java.io.*; //*Analysis goes here* //*Design goes here* class AllPermutation { public static void displayPermutation(String s) { //*Code goes here* } public static void displayPermutation(String s1, String s2) { //*Code goes here* } } //*Driver class should not be changed* class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); AllPermutation.displayPermutation(input.nextLine()); } }arrow_forwardDetermine whether a string is a palindromeA palindrome is a string of characters that reads the same from right to left as it does from left to right, regardless of punctuation and spaces.The specifications for this assignment are: •Write and test a non-recursive solution in Java that determines whether a string is a palindrome •Your program should consist of at least two methods: (1) the main method (2) the method which performs the task of determining whether the specified string is a palindrome. You should name this method isPalindrome. You should name the class that contains your “main” method and the isPalindrome method FindPalindrome. •You must use a Stack and a Queue in your solution: Write your own Stack and Queue based on the Vector in the Java API and use those in your solution. You should name those classes StackVector and QueueVector respectively. You already have access to the relevant exception classes and interfaces for the above ADTs. •All of your belong to a Java…arrow_forward
- Correct Code for Java: Enter an integer >> 11 Enter another integer >> 23 The sum is 34 The difference is -12 The product is 253 import java.util.Scanner; public class DebugTwo2 { public static void main(String args[]) { int a, b; Scanner input = new Scanner(System.in); System.out.print("Enter an integer >> "); a = nextInt (); System.out.print("Enter b = nextInt (); System.out.println("The System.out.println("The System.out.println("The } } another integer >> "); sum is " + (a + b)); difference is " + (a - b)); product is + (a*b));arrow_forwardChange Java code below so that it outputs a 9x9 board and not a 3x3 board. import java.util.*; public class Tic_Tac_Toe{ static String[] board;static String turn;static String checkWinner(){for (int a = 0; a < 8; a++) {String line = null; switch (a) {case 0:line = board[0] + board[1] + board[2];break;case 1:line = board[3] + board[4] + board[5];break;case 2:line = board[6] + board[7] + board[8];break;case 3:line = board[0] + board[3] + board[6];break;case 4:line = board[1] + board[4] + board[7];break;case 5:line = board[2] + board[5] + board[8];break;case 6:line = board[0] + board[4] + board[8];break;case 7:line = board[2] + board[4] + board[6];break;}//For X winnerif (line.equals("XXX")) {return "X";} // For O winnerelse if (line.equals("OOO")) {return "O";}} for (int a = 0; a < 9; a++) {if (Arrays.asList(board).contains(String.valueOf(a + 1))) {break;}else if (a == 8) {return "draw";}} // To enter the X Or O at the exact place on board.System.out.println(turn + "'s turn; enter…arrow_forwardAssign is Teenager with true if kidAge is 13 to 19 inclusive. Otherwise, assign is Teenager with false. 439894.2564214.qx3zqy7 1 import java.util.Scanner; □NM nor 2 3 public class TeenagerDetector { 4 5 6 7 8 9 10 11 12 13 14 15 16 17 public static void main (String [] args) { Scanner scnr = new Scanner(System.in); boolean isTeenager; int kidAge; kidAge scnr.nextInt(); * Your solution goes here if (isTeenager) { System.out.println("Teen"); } else { System.out.println("Not teen");arrow_forward
- Getting an error while running my code. import java.util.Scanner;import java.math.BigInteger; public class Main{Bigintege result;public static void computeFact(int n){BigInteger result = BigInteger.ONE;while (n>0){result = result.multiply(BigInteger.valueOf(n));n = n-1;}}public static void computeFact2(int n){BigInteger a = 4n;BigInteger fact = 1;while(n>0) {fact = fact.multiply(BigInteger.valueOf(4n));a = a - 1;}}BigInteger b=396, c, d;public static void compute(int n) {BigInteger sum = 0, final_result;public static void computeSummation() { while(n > 0) { sum = sum + (a/result) * (c); n = n-1; } final_result = d * sum;System.out.println("Final result is "+final_result);} public static void main(String[] args) { Scanner kybd = new Scanner(System.in); while ( true) { System.out.print("\n\tEnter an Integer Number e.g. 5 "); int num = kybd.nextInt(); computeFact(num); computeFact2(num); compute(num); computeSummation(num);} }}arrow_forwardJAVA PROGRAMMING LANGUAGE PLEASE. Convert the following UML to code in the image given below.arrow_forwardimport java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forward
- import java.util.Scanner; public class LabProgram { // Recursive method to draw the triangle public static void drawTriangle(int baseLength, int currentLength) { if (currentLength <= 0) { return; // Base case: stop when currentLength is 0 or negative } // Calculate the number of spaces needed for formatting int spaces = (baseLength - currentLength) / 2; if (currentLength == baseLength) { // If it's the first line, don't output spaces before the first '*' System.out.println("*".repeat(currentLength) + " "); } else { // Output spaces and asterisks System.out.println(" ".repeat(spaces) + "*".repeat(currentLength) + " "); } // Recursively call drawTriangle with the reduced currentLength drawTriangle(baseLength, currentLength - 2); } public static void drawTriangle(int baseLength) { drawTriangle(baseLength, baseLength); } public static…arrow_forwardJava programming: What's the output of the following program... a.) Compilation Error b.) 97~B~C~D~E~102~G~ c.) 65~b~C~d~E~70~G~ d.) a~66~C~68~E~f~G~arrow_forwardFix the code below so that there is a function that calculate the height of the triangle. package recursion; import javax.swing.*;import java.awt.*; /** * Draw a Sierpinski Triangle of a given order on a JPanel. * * */public class SierpinskiPanel extends JPanel { private static final int WIDTH = 810; private static final int HEIGHT = 830; private int order; /** * Construct a new SierpinskiPanel. */ public SierpinskiPanel(int order) { this.order = order; this.setMinimumSize(new Dimension(WIDTH, HEIGHT)); this.setMaximumSize(new Dimension(WIDTH, HEIGHT)); this.setPreferredSize(new Dimension(WIDTH, HEIGHT)); } public static double height(double size) { double h = (size * Math.sqrt(3)) / 2.0; return h; } /** * Draw an inverted triangle at the specified location on this JPanel. * * @param x the x coordinate of the upper left corner of the triangle * @param y the y…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
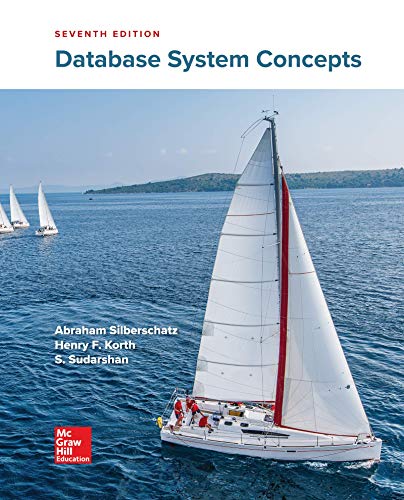
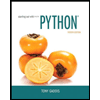
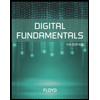
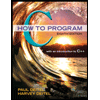
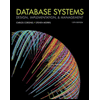
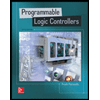