Write a program that reads a list of integers from the keyboard and creates the following information. a. Finds and prints the sum and the average of the integers b. Finds and prints the largest and the smallest integer c. Prints a Boolean (true or false) if some of them are less than 20 d. Prints a Boolean (true or false) if all of them are between 10 and 90 The input data consist of a list of integers with a sentinel. The program must prompt the user to enter the integers, one by one, and enter the sentinel when the end of the list has been reached. The prompt should look like the following: Enter the numbers with (99999 to stop): The output should be formatted as shown below: The number of integers is: xxx The sum of the integers is: xxxx The average of the integers is: xxx.xx The smallest integer is: xxx The largest integer is: xxx At least one number was < 20: All numbers were ( 10 <= n >= 90):
Write a program that reads a list of integers from the keyboard and creates the
following information.
a. Finds and prints the sum and the average of the integers
b. Finds and prints the largest and the smallest integer
c. Prints a Boolean (true or false) if some of them are less than 20
d. Prints a Boolean (true or false) if all of them are between 10 and 90
The input data consist of a list of integers with a sentinel. The program must
prompt the user to enter the integers, one by one, and enter the sentinel when
the end of the list has been reached. The prompt should look like the following:
Enter the numbers with <return>(99999 to stop):
The output should be formatted as shown below:
The number of integers is: xxx
The sum of the integers is: xxxx
The average of the integers is: xxx.xx
The smallest integer is: xxx
The largest integer is: xxx
At least one number was < 20: <true or false>
All numbers were ( 10 <= n >= 90): <true or false>

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

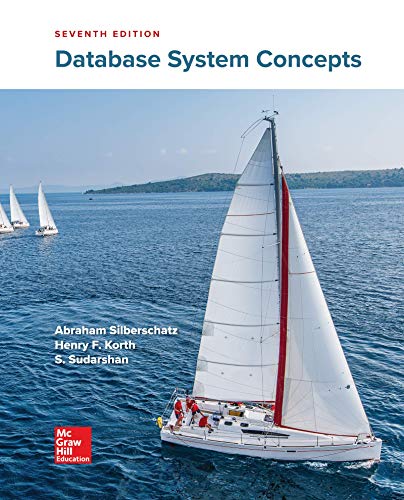
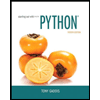
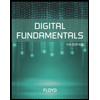
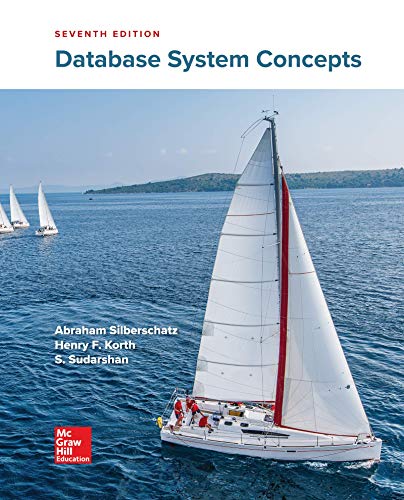
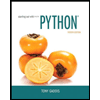
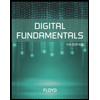
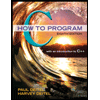
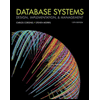
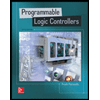