Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x > 0: Output x % 2 (remainder is either 0 or 1) x = x // 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Your program must define and call the following two functions. The function integer_to_reverse_binary() should return a string of 1's and 0's representing the integer in binary (in reverse). The function reverse_string() should return a string representing the input string in reverse. def integer_to_reverse_binary(integer_value) def reverse_string(input_string) Using Python please!!
Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the
As long as x > 0:
Output x % 2 (remainder is either 0 or 1)
x = x // 2
Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string.
Your program must define and call the following two functions. The function integer_to_reverse_binary() should return a string of 1's and 0's representing the integer in binary (in reverse). The function reverse_string() should return a string representing the input string in reverse.
def integer_to_reverse_binary(integer_value)
def reverse_string(input_string)
Using Python please!!
Here is the code I currently have, the error states that 'input_string is not defined'.
def integer_to_reverse_binary(integer_value):
integer_value = int(input())
integer_string = []
while integer_value > 0:
x == integer_value % 2
integer_string.append(x)
integer_value == integer_value // 2
return integer_string
def reverse_string(input_string):
y = len(integer_to_reverse_binary(integer_value))
while y > 0:
print(integer_value[y], end = '')
y == y - 1
if __name__ == '__main__':
reverse_string(input_string)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

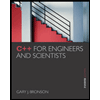
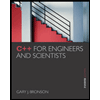