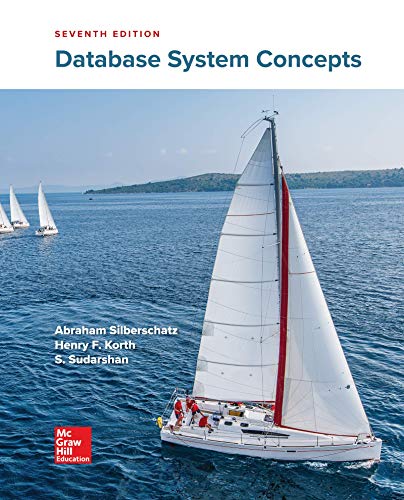
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a
That expression will multiply x by each of the numbers 1 through 12.
Display numbers on each line in order.
Produce one for loop and an identical while loop performing the identical process in one program.
( I highlighted where I get confused, and where I get the syntax error. Am I incoroprating the highlighted parts in the string. //Your Code here! (what does that mean.
![```plaintext
Java Program Code with Syntax Error:
int n=7, i, mul=0;
for(i=1; i<=12; i++) {
mul=(n*i);
System.out.println(n+"x"+i+"="+mul);
}
Error Message:
SyntaxError
Invalid syntax.
```
### Detailed Explanation
#### Code Analysis:
The code snippet on the left is written in a Java-like pseudocode and attempts to calculate and print the multiplication table for the number 7 up to 12. Here is how the code works:
1. **Initialization:**
- `int n=7, i, mul=0;` initializes the variable `n` with the value 7, which is the number to be multiplied. Variables `i` and `mul` are also initialized.
2. **For Loop:**
- `for(i=1; i<=12; i++)` iterates from 1 through 12.
- Inside the loop, `mul=(n*i);` calculates the product of `n` and `i`.
- `System.out.println(n+"x"+i+"="+mul);` prints the multiplication results in the format `n x i = mul`.
#### Error Description:
There is a syntax error highlighted by a popup message indicating "invalid syntax". This might be due to a missing part of the Java program structure, such as the enclosing `public class` and `main` method declarations, or simply the context in which this code is being executed isn't Java.
#### Correct Java Code Template:
In contrast, the corrected Java program structure is provided on the right, ensuring proper syntax:
```java
public class Main {
public static void main(String[] args) throws Exception {
int n=7, i, mul=0;
for(i=1; i<=12; i++) {
mul = (n*i);
System.out.println(n + " x " + i + " = " + mul);
}
}
}
```
- This corrected version includes the necessary `public class Main` and `public static void main` with the proper syntax needed to execute a Java program.](https://content.bartleby.com/qna-images/question/2d158991-3671-4459-aef6-bd0615668acc/70028c1d-8f2d-4c13-bf9d-a88e15b65609/sfxjsnq_thumbnail.jpeg)
Transcribed Image Text:```plaintext
Java Program Code with Syntax Error:
int n=7, i, mul=0;
for(i=1; i<=12; i++) {
mul=(n*i);
System.out.println(n+"x"+i+"="+mul);
}
Error Message:
SyntaxError
Invalid syntax.
```
### Detailed Explanation
#### Code Analysis:
The code snippet on the left is written in a Java-like pseudocode and attempts to calculate and print the multiplication table for the number 7 up to 12. Here is how the code works:
1. **Initialization:**
- `int n=7, i, mul=0;` initializes the variable `n` with the value 7, which is the number to be multiplied. Variables `i` and `mul` are also initialized.
2. **For Loop:**
- `for(i=1; i<=12; i++)` iterates from 1 through 12.
- Inside the loop, `mul=(n*i);` calculates the product of `n` and `i`.
- `System.out.println(n+"x"+i+"="+mul);` prints the multiplication results in the format `n x i = mul`.
#### Error Description:
There is a syntax error highlighted by a popup message indicating "invalid syntax". This might be due to a missing part of the Java program structure, such as the enclosing `public class` and `main` method declarations, or simply the context in which this code is being executed isn't Java.
#### Correct Java Code Template:
In contrast, the corrected Java program structure is provided on the right, ensuring proper syntax:
```java
public class Main {
public static void main(String[] args) throws Exception {
int n=7, i, mul=0;
for(i=1; i<=12; i++) {
mul = (n*i);
System.out.println(n + " x " + i + " = " + mul);
}
}
}
```
- This corrected version includes the necessary `public class Main` and `public static void main` with the proper syntax needed to execute a Java program.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In java please. thank you!arrow_forwardusing python language Using the piece of code on the previous slide, modify the code removing the x += 1 portion and run the code. What happens? Now modify the code to print the numbers but in reverse order Lastly, modify the code to print the numbers 1 through 10 which are not divisible by 3arrow_forwardWrite a program that asks a user to input the height of triangle and draw a triangle that has the following pattern. Use nested loops for this problem. If the user enter height as 4 Then the triangle output is 1 1 2 1 2 3 1 2 3 4 We make the following assumptions • There is no space between the margin and first number in each row • There could be a space after the last number on every line • There is pne space between numbers on every line • There maybe a newline at the end of last line There is one test-case to check your code, there are two hidden testcases that tests correctness of your code 376584 2469arrow_forward
- Please &-. Write a Java program to check if a given string is a palindrome or not. A palindrome is a word, phrase, number, or other sequence of characters that reads the same backward as forward, ignoring spaces, punctuation, and capitalization. For example, "racecar" and "Madam" are palindromes, while "hello" and "Java" are not. Your program should take a string as input and return true if it's a palindrome, and false otherwise. Ik.?arrow_forwardIn the following code stream, identify if there are any hazards. Crisply describe if it is possible to get around the hazards, and what the resulting steps will be. lw r2, 0(r1) add r3, r2, r3 sub r1, r1, r4arrow_forwardWrite a program that displays a table of pounds and equivalent weights in kilograms. You can find the conversion factor online if necessary. Store this factor in a properly named constant. Allow the user to specify the range of pounds for the table by prompting for the start, stop, and step values for the pounds column. Display the pounds accurate to two decimal places centered in a column 10 characters wide. The kilometers should display accurate to three decimal places and right-aligned in a column 12 characters wide (see page 75-78). Column headings should be displayed with the same alignments. See Sample Output.arrow_forward
- Create a single regular expression that matches a string of digits and contains exactly two fives. Examples of acceptable strings include: "15445 " , " 55 " , " 05563 " . However, the string is to be rejected if it contains anything other than digits.arrow_forwardProblem 2: Using HTML/JavaScript, find and display the number of multiples of a certain number entered by the user. First have the user enter a number in a prompt box from 1-10. Check if they enter a valid number - if they don't, prompt them again. Use a loop for this. Next, use a second loop to have the user enter a number in a prompt box and determine if it is a multiple of the number they entered. Keep count of how many multiples of their number they entered. Have the user enter a 0 when they are finished entering numbers. At the end, print out the number of multiples of their number they entered.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
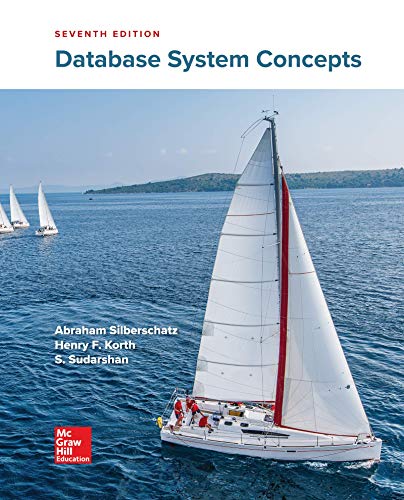
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
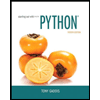
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
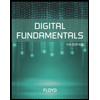
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
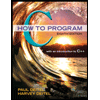
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
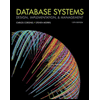
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
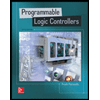
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education