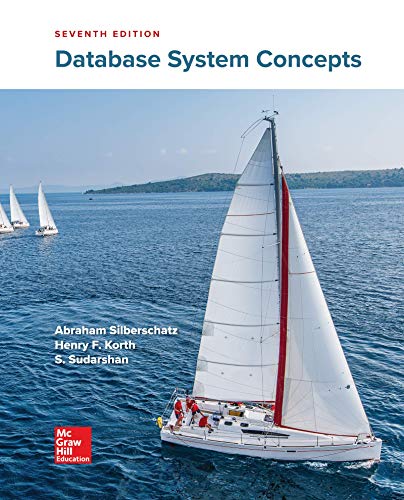
Concept explainers
Write a program to allow a user to play the game, Hangman. DO NOT USE AN ARRAY
The program will generate a random number (between 1 and 4581) to pick a word from the file - this is the word you then have to guess.
Note: if the random number you generate is 42, you need the 42nd word - so loop 41 times picking up the word from the file and not doing anything with it, it is the 42nd you need. Here is how you will do this:
String word:
for (int k=0; k<41; k++)
{word=scnr.nextLine();
}//end loop and now pick up the word you want
word=scnr.nextLine() //this is the one you want
The user will be allowed to guess a letter as many times as it takes - but 10 wrong guesses and they lose!!
Eventually either they won or lost. In case they lost print out the answer.
Java

The code for the given problem is written below in java-
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Random;
import java.util.Scanner;
public class Main{
/*
* Creating a Scanner class object which is used to get the inputs
* entered by the user
*/
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) throws FileNotFoundException {
int size=0;
char ch;
String word,randWord = null;
//Creating a random Class object
Random r = new Random();
Scanner file=new Scanner(new File("wordList.txt"));
while(file.hasNext())
{
word=file.next();
size++;
}
file.close();
while(true)
{
int num=r.nextInt((size - 1) + 1) + 1;
file=new Scanner(new File("wordList.txt"));
for(int i=1;i<=num;i++)
{
randWord=file.next();
}
file.close();
// calling a Method
guessWord(randWord.toLowerCase());
// Prompting the user to run again
System.out.print("\nDo you Want to play again (Y/N):");
ch = sc.next(".").charAt(0);
if (ch == 'y' || ch == 'Y') {
continue;
} else {
break;
}
}
}
//This method will ask the user to enter characters until user won or lost the game
private static void guessWord(String string) {
final int NO_OF_MISSES = 10;
char ch;
int miss = 0, flag, match = 0;
int len = 0;
char arr[] = new char[string.length()];
for (int i = 0; i < string.length(); i++) {
if ((string.charAt(i) >= 97 && string.charAt(i) <= 122) || (string.charAt(i) >= 65 && string.charAt(i) <= 90)) {
arr[i] = '_';
len++;
} else {
arr[i] = string.charAt(i);
}
}
while (len != 0) {
flag = 0;
match = 0;
System.out.print("Guess:");
for (int i = 0; i < string.length(); i++) {
System.out.print(arr[i] + " ");
}
System.out.print("\nPick a letter:");
ch = sc.next(".").charAt(0);
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) {
if (Character.isUpperCase(ch)) {
ch = Character.toLowerCase(ch);
}
} else {
System.out.println("** Not a letter **");
continue;
}
for (int i = 0; i < string.length(); i++) {
if (string.charAt(i) == ch) {
if(arr[i]=='_')
{
arr[i] = ch;
len--;
}
flag = 1;
match++;
}
}
if (flag == 0) {
System.out.println("There is no letter " + ch + ".");
miss++;
System.out.println("No of Chances left :"+(NO_OF_MISSES-miss));
if (miss == NO_OF_MISSES) {
System.out.println("You Lost the game.");
System.out.println("The Word is :" + string);
break;
}
} else {
System.out.println("There is " + match + " letter " + ch + ".");
}
}
if (len == 0)
System.out.println("You won the game.");
}
}
Step by stepSolved in 2 steps with 5 images

- • • Asks the user for their name and store it in an array Asks the user for their age and store it in a variable Determines how many years before they can retire at age 67. If user is 67 then it will indicate that the user should retire now. If user is over 67 it will indicate that the user should have already retired If the user is under 67 then it will indicate number of years before retirement Ends with a thank you message. Example of Output Welcome to nameage Please enter name: (user enters a value like Pat) Please enter your age: (user enters a value like 69) Hi Pat. You should already be retired. Thank you for using nameage. Bye!arrow_forwardDesign a program that will use a pair of nested loop to read the company's sales amounts. The details are as follows: -KDS Company has 3 departments: 1. Dept X 2. Dept Y 3. Dept Z. Design a program that will read as input each department's sales for each quarter of the year, and display the result for all divisions. Use a two dimensional array that will have 3 rows and 4 columns and show how the data will be organized. Please provide solution using Python. Thank you.arrow_forwardWhen using two loops to traverse an array, you can use the For...Next statement to code the outer loop and use the For Each...Next statement to code the nested loop. True or False?arrow_forward
- The Fibonacci numbers are 0, 1, 1, 2, 3, 5, 8, 13, ... where each number (after the first two values) is the sum of the previous two numbers. Write a program that declares an integer array called fib_numbers with a length of 25 elements. The program then fills the array with the first 25 Fibonacci numbers using a loop. The program then prints the values to the screen using a separate loop.arrow_forwardUse an array. The program should read 25 integers from a user. Each value should be between 5- 100 (5<-value<-100). As the user inputs values store all the values which are not a duplicate. Print all the array values at the end. Use the smallest possible array.arrow_forwardPlease use java as the programing language and write comments! Thanks!arrow_forward
- Hello, Stuck on this question. All programming is in Python. 2.) Design a program that generates a 7-digit lottery number. The program should have an INTEGER array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then write another loop that displays the contents of the array. All programming is in Python.arrow_forwardCreate a Console application that does the following:This program must use a single array to store integers and find the maximum value.1. Create an array and initialize it internally with the values 5, 8, 9, 4, and 2.2. Use a loop to iterate through the array and determine the maximum value.arrow_forwardThere are 2 arrays, the first contains item numbers in inventory and the second parallel array contains the price of the items. The user will enter the item number they want to purchase, the program checks to see if it is a valid entry. If it is valid, it will tell the user the price of the item. If it is an invalid item, a message will display telling the user they entered an invalid item. Type up the code below, execute the program a few times.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
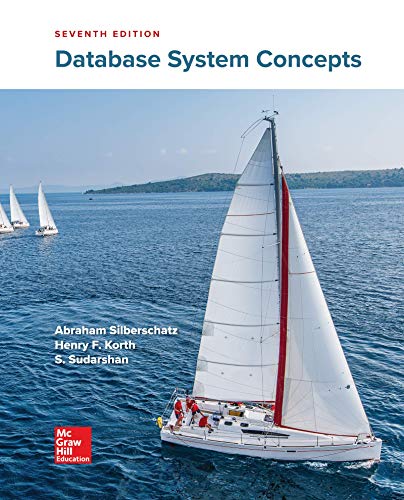
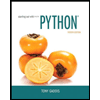
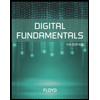
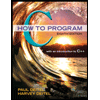
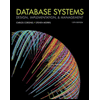
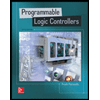