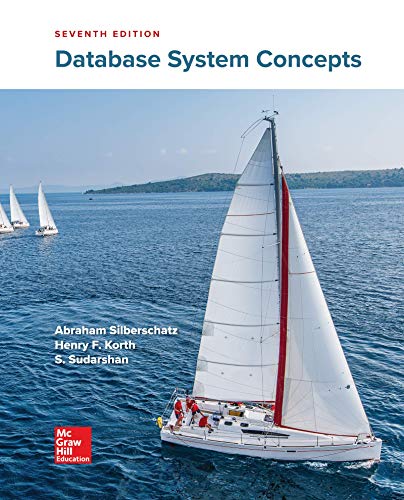
Write a python program and add a function called add_matrix() to it. This function
takes two 2-D lists of integers (matrix) as parameters and computes their sum, if a sum
can actually be computed. Otherwise, it returns an empty list. Note that, two matrices can
only be added together if they have the exact same dimensions. If the dimensions are
acceptable, then the resulting matrix will also have the same dimensions as its operands,
and the value of each element in the resulting matrix is the sum of the corresponding
elements in the input matrices.
Hint: test rectangularity (the number of rows and the number of elements in each row
must be the same in two matrices) before attempting addition.
Sample output:
>>> matrix1 = [[2,3], [4,5]]
>>> matrix2 = [[9,0], [8,6]]
>>> print(add_matrix(matrix1, matrix2))
[[11, 3], [12, 11]]

Step by stepSolved in 3 steps with 1 images

- In the following questions you need to import functions from Numpy. For example the computation of matrix determinants can be done with the help of the function det of the library numpy.linalg. Please list the commands required for the function to work The first photo is 3a. The other photo has the remaining questions.arrow_forwardWrite a function that takes two inputs: - An array of doubles named data. - A scalar double (single number) named threshold. Your function should have one output: - A double named below. The value of below should be the number of elements in data that have a value less than threshold. Please use MATLABarrow_forwardDevelop a function that finds the starting index of the longest subsequence of values that is strictly increasing. For example, given the array {12, 3, 7, 5, 9, 8, 1, 4, 6}, the function would return 6, since the subsequence {1, 4, 6} is the longest that is strictly increasing. Develop a function that takes a string and a single character, then returns a list of the indexes in the string where the character occurs. For example, given the string “bookkeeper” and ‘e’, the function would return {5, 6, 8} since ‘e’ occurs at those positions. Return an empty list if the character does not appear in the string even once. Develop a function that takes two lists of integers and returns a list containing any value that appears in both parameter lists.arrow_forward
- Write a program in PYTHON with a function max(L) which examines the argument list L, and returns the largest object of type float. If there is no float object in the list, then the function returns None. For example,max([100, 'blue', 3.5, 'sugar on the rocks', 7.0]) would return 7.0, andmax([7, 2, 9, 1]) would return None. Note that type(element) == float is a way to check if element is a floatarrow_forwardWrite a Python function (give it any name you choose) to analyze a text string given to it. The function will receive one single string as argument It will return two tuples of two elements each: One tuple contains the word with the maximum occurrence in the input text and the number of its occurrence. The other tuple contains the longest word with in the input text and the number of characters in it. The function should be called like function1(text), where text is a string variable. Use the paragraph below to test your code: text = ‘’’During a wet autumnal walk, I was explaining to my girlfriend about my recent presentations. I've been doing my 'Getting Started with Python' talk at Aruba Airheads meet-ups. I recorded an early version of it, see below. One point I mentioned is that the reaction is always mixed. When I ask who is learning Python, about 5% of each audience put their hands up. Regularly people object to the idea of network engineers learning Python. The arguments…arrow_forwardWrite a function lis_rec(arr) that outputs the length of the longest increasing sequence and the actual longest increasing sequence. This function should use divide and conquer strategy to the solve the problem, by using an auxiliary function that is recursive. For example, one possibility is to define a recursive function, lis_rec(arr, i, prev), that takes the array arr, an index i, and the previous element index prev of LIS (which is part of the array arr before index i), and returns the length of the LIS that can be obtained by considering the subarray arr[i:]. Write a dynamic programming version ofthe function, lis_dp(arr), that outputs the length of the longest increasing sequence and the actual longest increasing sequence by using a table to store the results of subproblems in a bottom-up manner. Test the performance of the two functions on arrays of length n = 100, 500, 1000, 5000, 10000. Compare the running times and memory usage of the two functions.arrow_forward
- In python write a program that computes and prints the average of the numbers in a text file. Because the numbers are read in from a text file, the number inputs are considered text. For example, there is the number 5 and there is a text 5. You can't do arithmetic operations on text. It must be converted to the number equivalent. You will make use of a few higher-order functions, map(), lambda and reduce(), to simplify the design. The basic outline of this program is as follows: Ask user for input file name and check to be sure it is valid Prepare input file for reading Get the numbers from the input file in to a list variable Convert the list variable string items to float numbers using the map and list functions Compute the sum of the numbers using the reduce and lambda functions Print the average of the numbers in the list. If the length of the list is zero then the average is zero.arrow_forwardImplement a function pairsThatSum that accepts two arguments: 1. a target number2. a list of numbers the function then returns a list of tuple s that contains all pairs of numbers from the list that sum to the given target number. Note: the pair can be two numbers with the same value, e.g. (2,2) but these must be different items (have different locations) in the list. See the last example below. each pair should be reported only once (don't include a pair and its reverse), and each pair's values should be listed in the order that they occur in the list. E.g., the first example records the pair (0,3) instead of the pair (3,0) , because 0 occurs in the list before 4 . the list of pairs should be ordered by the order of the first value in the list, e.g., (0,3) comes before (1,2) because 0 comes before 1 in the list. (note: if you take the right approach, you won't have to do anything extra for this requirement) Below is an example of the output from the code: >>>…arrow_forwardWrite a function using Java Function Name: winInRowParameters: board: 2D integer array, row: integer, piece: integerReturn: booleanAssume board is valid 2D int array, row is valid index in the board, piece is X==1/O==2Look at indicated row at given index in board. If that row has at least 3 consecutive entries withgiven type of piece (X/O) (3 in a row XXX, OOO), then return true, otherwise false.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
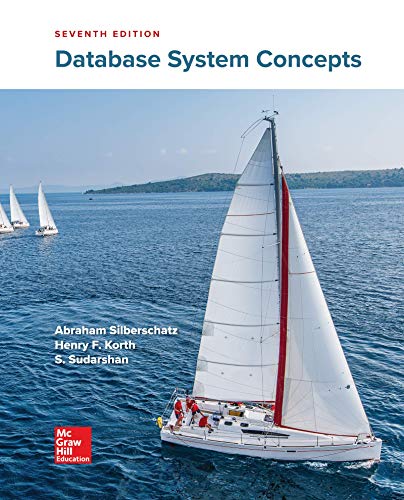
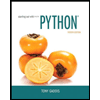
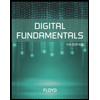
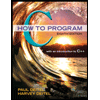
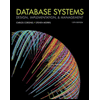
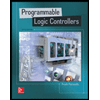