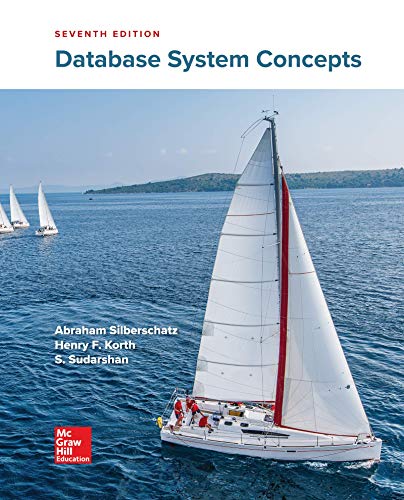
Concept explainers
Write a Python program that connects to a sqlite
Create a table called Horses with the following fields:
- id (integer): a primary key and not null
- name (text)
- breed (text)
- height (real)
- birthday (text)
Next, insert the following data row into the Horses table:
id: 1 name: 'Babe' breed: 'Quarter Horse' height: 15.3 birthday: '2015-02-10'
Output all records from the Horses table.
Ex: With the above row inserted, the output should be:
All Horses: (1, 'Babe', 'Quarter Horse', 15.3, '2015-02-10')
This is what I came up withbut it doesn't accomplish the task.
import sqlite3
from sqlite3 import Error
def create_connection(db_file):
""" create a database connection to the SQLite database
specified by db_file
:param db_file: database file
:return: Connection object or None
"""
conn = None
try:
conn = sqlite3.connect(db_file)
return conn
except Error as e:
print(e)
return conn
def create_table(conn, create_table_sql):
""" create a table from the create_table_sql statement
:param conn: Connection object
:param create_table_sql: a CREATE TABLE statement
:return:
"""
try:
cursor = conn.cursor()
cursor.execute(create_table_sql)
except Error as e:
print(e)
def insert_horse(conn_data):
cursor = conn.cursor()
cursor.execute(sql = insert_ horses(id,name,breed,height,birthday)
VALUES(?,?,?,?,?) '''
conn.commit()
def select_all_horses(conn):
cursor = conn.cursor()
cursor.execute(select_all_horses)
rows = cursor. fetchall()
for conn.fetchall
print(row)
if __name__ == '__main__':
database = "HorseTable.db"
conn = create_connection(database)
create_table_sql = """ CREATE TABLE IF NOT EXISTS Horses (
id integer PRIMARY KEY,
name text NOT NULL,
breed text,
height real,
birthday text
); """
create_table(conn, create_table_sql)
data = sql = Horses(id,name,breed,height,birthday)
VALUES(?,?,?,?,?) '''
insert_Horse(conn.data)
print(‘all .Horses)
print(‘Records Inserted’)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- with it, paste your code in a text file named Student.txt and save it in your task folder. Write the SQL code to create a table called Student. The table structure is summarised in the table below (Note that STU_NUM is the primary key): STU_NUM Attribute Name STU_SNAME STU_FNAME STU_INITIAL STU_STARTDATE COURSE_CODE PROJ_NUM STU STU_S STU_F STU_INITIAL NUM NAME NAME 01 02 Snow John E CHAR(6) After you have created the table in question 1, write the SQL code to enter the first two rows of the table as below: Stark Arya с VARCHAR(15) VARCHAR(15) CHAR(1) DATE CHAR(3) INT(2) Data Type STU_STARTDATE COURSE_ PROJ_ CODE NUM 05-Apr-14 12-Jul-17 201 305 6 11 Assuming all the data in the Student table has been entered as shown below, write the SQL code that will list all attributes for a COURSE_CODE of 305.arrow_forwardcreate database GA2gouse GA2gocreate table salesman(salesman_id int primary key, name varchar(30), city varchar(20), commission varchar(30));insert into salesman (salesman_id, name, city, commission) values (5001, 'James Hoog', 'New York', 0.15); insert into salesman (salesman_id, name, city, commission) values (5002, 'Nail Knite', 'Paris', 0.13); insert into salesman (salesman_id, name, city, commission) values (5005, 'Pit Alex', 'London', 0.11); insert into salesman (salesman_id, name, city, commission) values (5006, 'Mc Lyon', 'Paris', 0.14); insert into salesman (salesman_id, name, city, commission) values (5007, 'Paul Adam', 'Rome', 0.13); insert into salesman (salesman_id, name, city, commission) values (5003, 'Lauson Hen', 'San Jose', 0.12); Create table Customer (customer_id int primary key, cust_name varchar(30), city varchar(10), grade varchar(30), salesman_id int,CONSTRAINT FK_salesman_id FOREIGN KEY (salesman_id) REFERENCES salesman(salesman_id));insert into customer…arrow_forwardSQL CODE FOR For the players who show up in Batting, Bowling, and Fielding tables, create a list that shows their names, runs they have scored, wickets they have taken, and catches they have taken? table is in picture (bowling table is same as batting and fielding )arrow_forward
- Import the following database: CREATE DATABASE COUNTRIES; USE COUNTRIES; DROP TABLE IF EXISTS `City`; CREATE TABLE `City` ( `ID` int(11) NOT NULL AUTO_INCREMENT, `Name` char(35) NOT NULL DEFAULT '', `CountryCode` char(3) NOT NULL DEFAULT '', `District` char(20) NOT NULL DEFAULT '', `Population` int(11) NOT NULL DEFAULT '0', PRIMARY KEY (`ID`) ) ENGINE=MyISAM AUTO_INCREMENT=4080 DEFAULT CHARSET=latin1; -- -- Dumping data for table `City` -- -- ORDER BY: `ID` INSERT INTO `City` VALUES (1,'Kabul','AFG','Kabol',1780000); INSERT INTO `City` VALUES (2,'Qandahar','AFG','Qandahar',237500); INSERT INTO `City` VALUES (4,'Mazar-e-Sharif','AFG','Balkh',127800); INSERT INTO `City` VALUES (5,'Amsterdam','NLD','Noord-Holland',731200); INSERT INTO `City` VALUES (6,'Rotterdam','NLD','Zuid-Holland',593321);(3068,'Berlin','DEU','Berliini',3386667); INSERT INTO `City` VALUES (3069,'Hamburg','DEU','Hamburg',1704735); INSERT INTO `City` VALUES (3072,'Frankfurt am Main','DEU','Hessen',643821); INSERT INTO…arrow_forward1-Write the syntax to insert into a relational table called students the address column references an object table called addresses that was created using an address_type. Other columns exist in the students table, but you are only inserting into the ones below. Aliases used should be the first letter of the table name, eg students would be s, addresses would be a Relational Table Name students attribute student_id attribute surname attribute addressarrow_forwardImagine that you are a database manager at a company that makes custom shirts. One column in the database lists the wholesale price of the shirts. A second column lists the labor charge, which is currently set to 11 percent of the wholesale price of the shirt. A third column lists the retail price of the shirt, which is the combination of the wholesale price and the labor charge. Write the statements that calculate the values that belongs in the second and third columns. How could you use a query to format these columns?arrow_forward
- 1) Assume the EMPLOYEE table has the following rowsID DEPT SALARY NAME100 SALES 40000 Smith101 RD 38000 Terry102 HR 60000 David103 SALES 58000 Ellie104 RD 70000 Judya) Provide the complete PL/SQL code to implement a Virtual Private Database suchthat an employee could only view the records for employees in the samedepartment while masking coworkers’ salary with NULL.b) Based on the Virtual Private Database you implemented in step a), would anemployee be able to insert, update or delete a record for another employee whoworks in another department? If yes, what might be the potential security risk andhow would you fix the problem by setting up the VPD appropriately?arrow_forwardThird normal form says: a. No nonkey columns depend on another nonkey column. b. Every column that's not part of the primary key is fully dependent on the primary key. c. Eliminate repeated fields. d. None of the above.arrow_forwardFirst normal form says: a. No nonkey columns depend on another nonkey column b. Every column that's not part of the primary key is fully dependent on the primary key. c. Eliminate repeated fields. d. None of the abovearrow_forward
- The Sqlite.py file contains several very specific queries: select_Query = "select sqlite_version()" delete_query = "DELETE from Database where id = "+str(id) sel = 'SELECT id FROM Database WHERE name == "{0}"'.format(value) insert_query = """INSERT INTO Database (id, name, photo, html) VALUES (?, ?, ?, ?)""" sqlite_select_query = """SELECT * from Database""" table_query = '''CREATE TABLE Database ( id INTEGER PRIMARY KEY, name TEXT NOT NULL, photo text NOT NULL UNIQUE, html text NOT NULL UNIQUE)''' Write a QueryBuilder function. The QueryBuilder builds a generic Query to build ANY Query type (i.e. version, delete, select, insert, select, table). The QueryBuilder parameters require: The type of Query, the input tuple data and then constructs a query string based on the parameters.…arrow_forwardUsing php and sql: Create a PDO object to connect to your database for running select queries.Write a database query to output all the messages in the messages table.arrow_forwardcalculate_new_balance Given a starting balance (a number), and a list of transaction tuples, calculate the final balance for an account. Transaction tuples are of the shape ("description", amount, "withdrawal") , or ("description", amount, "deposit"). The last entry in the tuple will be either "withdrawal" or "deposit". Every withdrawal decreases the balance of the account by the specified amount, and every deposit increases the balance. The return value is the new account balance, as a number. (which could be negative) Sample calls should look like: >>> calculate_new_balance(100, [("payday", 20, "deposit"), ("new shoes", 50, "withdrawal"), ("illicit winnings", 200, "deposit")])270>>> calculate_new_balance(100, [])100arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
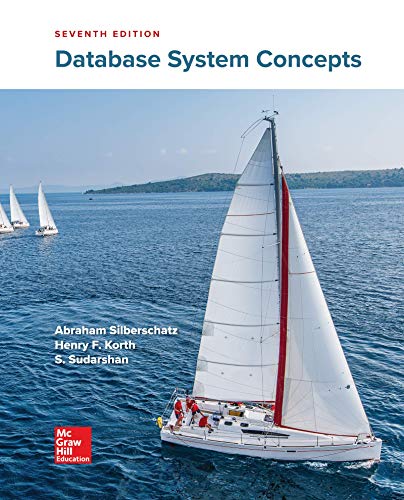
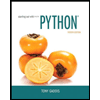
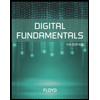
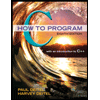
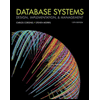
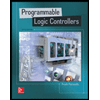