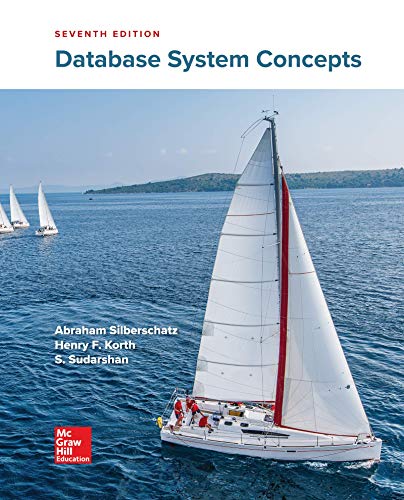
Write a Python program that does following:
(1) Prompt the user to enter a string of their choosing. Store the text in a string. Output the string.
Ex:
Enter a sample text:
we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
You entered: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
(2) Implement a print_menu() function, which has a string as a parameter, outputs a menu of user options for analyzing/editing the string, and returns the user's entered menu option and the sample text string (which can be edited inside the print_menu() function). Each option is represented by a single character.
If an invalid character is entered, continue to prompt for a valid choice.
Hint: Implement the Quit menu option before implementing other options. Call print_menu() in the main section of your code. Continue to call print_menu() until the user enters q to Quit.
Ex:
MENU
c - Number of non-whitespace characters
w - Number of words
f - Fix capitalization
r - Replace punctuation
s - Shorten spaces
q - Quit
Choose an option:
(3) Implement the get_num_of_non_WS_characters() function. get_num_of_non_WS_characters() has a string parameter and returns the number of characters in the string, excluding all whitespace. Call get_num_of_non_WS_characters() in the print_menu() function.
Ex:
Number of non-whitespace characters: 181
(4) Implement the get_num_of_words() function. get_num_of_words() has a string parameter and returns the number of words in the string. Hint: Words end when a space is reached except for the last word in a sentence. Call get_num_of_words() in the print_menu() function.
Ex:
Number of words: 35
(5) Implement the fix_capitalization() function. fix_capitalization() has a string parameter and returns an updated string, where lowercase letters at the beginning of sentences are replaced with uppercase letters. fix_capitalization() also returns the number of letters that have been capitalized. Call fix_capitalization() in the print_menu() function, and then output the the edited string followed by the number of letters capitalized. Hint 1: Look up and use Python functions .islower() and .upper() to complete this task. Hint 2: Create an empty string and use string concatenation to make edits to the string.
Ex:
Number of letters capitalized: 3
Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue!
(6) Implement the replace_punctuation() function. replace_punctuation() has a string parameter and two keyword argument parameters exclamation_count and semicolon_count. replace_punctuation() updates the string by replacing each exclamation point (!) character with a period (.) and each semicolon (;) character with a comma (,). replace_punctuation() also counts the number of times each character is replaced and outputs those counts. Lastly, replace_punctuation() returns the updated string. Call replace_punctuation() in the print_menu() function, and then output the edited string.
Ex:
Punctuation replaced
exclamation_count: 1
semicolon_count: 2
Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. nothing ends here, our hopes and our journeys continue.
(7) Implement the shorten_space() function. shorten_space() has a string parameter and updates the string by replacing all sequences of 2 or more spaces with a single space. shorten_space() returns the string. Call shorten_space() in the print_menu() function, and then output the edited string. Hint: Look up and use Python function .isspace().
Ex:
Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
# Type all other functions here
def print_menu(usr_str):
menu_op = ' '
# Complete print_menu() function
return menu_op, usr_str
if __name__ == '__main__':
# Complete main section of code

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- 1. Write a program that reads a number between 1,000 and 999,999 from the user, where the user enters a comma in the input. Then print the number without a comma. Here is a sample dialog; the user input is in color: Please enter an integer between 1,000 and 999,999: 23,456 23456 Hint: Read the input as a string. Measure the length of the string. Suppose it contains n characters. Then extract substrings consisting of the first n - 4 characters and the last three characters.arrow_forwardJava Question 5arrow_forwardWrite a program that prompts the user for a month name and a month day. Your program will examine the month and the day to determine the season. Your program will then display the date's season (Winter, Spring, Summer or Autumn). The input is a string to represent the month and an int to represent the day. See chart below for the range of dates within seasons. Ex: If the input is: April 11 the output is: Spring The dates for each season in the northern hemisphere are:Spring: March 20 - June 20Summer: June 21 - September 21Autumn: September 22 - December 20Winter: December 21 - March 19arrow_forward
- Samething this is not a graded question it's all in python.arrow_forwardPYTHON Given a string, print the sum and the average of the numbers that appear in the string and round to one decimal point. Sample Input Calc = 88 Biology = 90 History = 63 Sociology = 60 Sample Output Total points: 301, Average: 75.3arrow_forwardPasswords that are created by users are usually very simple and easy to guess. Create a Python program that takes a simpler pswrd + makes it stronger by taking characters using the rules below, and by appending "!" to the ending of the input string. i becomes 1 a becomes @ m becomes M B becomes 8 s becomes $ Example: If the input is: mypassword the output is: Myp@$$word! Hint: Python strings are immutable, but support string concatenation. Store and build the stronger password in the given password variable This is what I have so far.... word = input()password = ''arrow_forward
- (1) Prompt the user to enter a string of their choosing. Store the text in a string. Output the string. Ex: Enter a sample text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! You entered: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! (2) Implement the PrintMenu() function to print the following command menu. Ex: MENU c - Number of non-whitespace characters w - Number of words f - Find text r - Replace all !'s s - Shorten spaces q - Quit (3) Implement the ExecuteMenu() function that takes 2 parameters: a character representing the user's choice and the user provided sample text. ExecuteMenu() performs the menu options, according to the…arrow_forwardMad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways. Write a program that takes a string and an integer as input, and outputs a sentence using the input values as shown in the example below. The program repeats until the input string is quit and disregards the integer input that follows. Ex: If the input is: apples 5 shoes 2 quit 0 the output is: Eating 5 apples a day keeps the doctor away. Eating 2 shoes a day keeps the doctor away.arrow_forwardWrite a program that takes in two strings and returns the longest string. If they are the same length then return the second string. Ex. If the input is: almond pistachio the output is: pistachio python ''' Type your code here. '''arrow_forward
- I need this answered in Python with code commentsarrow_forward(1) Prompt the user to enter a string of their choosing. Store the text in a string. Output the string. Ex: Enter a sample text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! You entered: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more teachers in space. Nothing ends here; our hopes and our journeys continue! (2) Implement a PrintMenu() function, which has a string as a parameter, outputs a menu of user options for analyzing/editing the string, and returns the user's entered menu option. Each option is represented by a single character. If an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options. Call PrintMenu() in the main() function. Continue to call…arrow_forwardDo it in javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
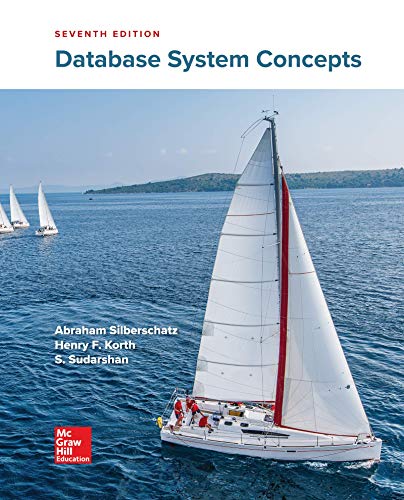
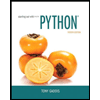
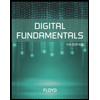
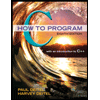
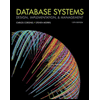
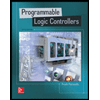