Write a Python program that will read in a text file named "input.txt" which holds varied input. A sample file has been given to you in Canvas to test your program. Read through this input file and display the following information: the total number of characters with spaces (whitespace characters) the total number of characters without spaces the total number of uppercase characters the total number of lowercase characters the total number of digits the total number of spaces (whitespace characters) the most frequent character in the file In addition to the above, be sure your code handles the following exception: any IOError exception that is thrown when the file is opened and data is read from it Requirements: use functions throughout. The function main should only call functions in the order needed. keep the functions modular and performing only one task. add a beginning and ending statement so the user will know when the program begins and ends. comment throughout the code. the program should look for the file in the current directory. An Example: If the input file contains: Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. Then the program output should look something like this: Character Analysis Opening the input file for processing.... Processing..... Number of characters with spaces: 245 Number of characters without spaces: 203 Number of uppercase characters: 4 Number of lowercase characters: 199 Number of digits: 4 Number of spaces: 42 Most frequent character in the file: e Input file closed... End of analysis.
Write a Python program that will read in a text file named "input.txt" which holds varied input. A sample file has been given to you in Canvas to test your program.
Read through this input file and display the following information:
- the total number of characters with spaces (whitespace characters)
- the total number of characters without spaces
- the total number of uppercase characters
- the total number of lowercase characters
- the total number of digits
- the total number of spaces (whitespace characters)
- the most frequent character in the file
In addition to the above, be sure your code handles the following exception:
- any IOError exception that is thrown when the file is opened and data is read from it
Requirements:
- use functions throughout. The function main should only call functions in the order needed.
- keep the functions modular and performing only one task.
- add a beginning and ending statement so the user will know when the program begins and ends.
- comment throughout the code.
- the program should look for the file in the current directory.
An Example:
If the input file contains:
Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. |
Then the program output should look something like this:
Character Analysis Opening the input file for processing.... Processing..... Number of characters with spaces: 245 Input file closed... End of analysis. |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

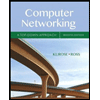
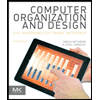
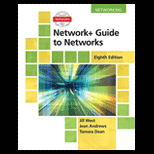
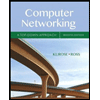
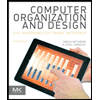
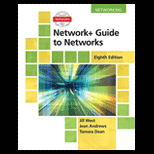
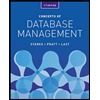
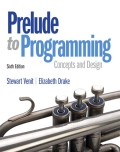
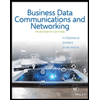