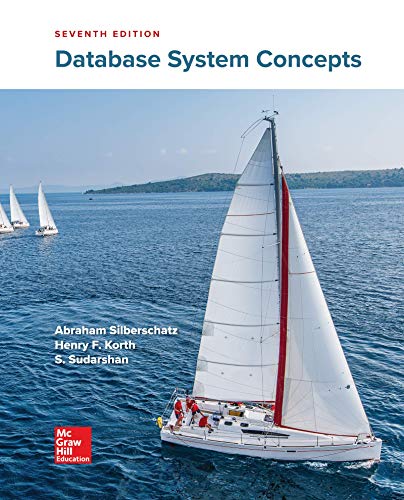
write a python program which do the following:
A simple course management system models a student’s information with a name and a set of scores. This system should be able to create a student object with a given name and a number of scores, all of which will be 0 at startup. The system should be able to access or replace a score at a given position (counting from 0), obtain the number of scores, obtain the highest score, obtain the average score, and obtain the student’s name. In addition, the system should have a method to display name, scores, highest score and average score of a student. Please define a class named Student to implement this course management system. Please use the starter code student.py.
Expected output:
The list of students:
Name: Juan
Scores: 71 75 89
Highest score: 89.00
Average score: 78.33
Name: Bill
Scores: 97 89 90
Highest score: 97.00
Average score: 92.00
Name: Stacy
Scores: 99 83 87
Highest score: 99.00
Average score: 89.67
Name: Maria
Scores: 73 97 92
Highest score: 97.00
Average score: 87.33
Name: Charley
Scores: 95 89 92
Highest score: 95.00
Average score: 92.00

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- ive been struggling with this all week. please in pythonarrow_forwardProgramming in Python Unit 3 Assignment2InstructionsWrite a program to simulate a bank transaction. There are two bank accounts: checking and savings.First, ask for the initial balances of the bank accounts; reject negative balances and set to zero. Then askfor the transaction; options are deposit, withdrawal, and transfer. Then ask for the account; options arechecking and savings. Then ask for the amount; reject transactions that overdraw an account. At theend, print the balances of both accounts.arrow_forward0 Judging rules can be difficult – even for an objective computer program. In football (orsoccer as some people call it), the official rules say that the referee can allow the playto continue ‘when the team against which an offence has been committed will benefitfrom such an advantage’ and penalize ‘the original offence if the anticipated advantagedoes not ensue at that time’ (Federation Internationale de Football Association 2003).How would you implement this rule? What difficulties are involved in it?arrow_forward
- Write a Java program that simulates a meeting reservation system. The program shall allow the user to select from the following options: Create a new meeting Show meetings on the calendar Clear all meetings Each meeting has a subject, start day/time and end day/time Subject is a short text description of the meeting Day is a date that contains month, day, and year Meeting times need only deal with hour and minute When the user wants to create a new meeting, the program asks for the subject, start and end day/times for it and adds it to the calendar For the basic requirements, meetings are not allowed to overlap. If a meeting the user wants to schedule overlaps with an existing meeting, the program presents an error message showing which meeting the one the user wants to schedule overlaps with When the user wants to show all meetings for the week, the report displays all meetings each day as follows Show all meetings in chronological order At the end of the report, show a…arrow_forwardIn Python Write a class called OrthokonBoard that represents the board for a two-player game that is played on a 4x4 grid. This class does not do everything needed to play a game - it's just responsible for handling the rules concerning the game board. Things like asking the user for moves, printing results for the user, keeping track of whose turn it is, and running the game loop would be the responsibility of one or more other classes. A large, complex program can be simplified by being broken down into multiple classes, each of which has multiple methods. Objects of these different classes then interact with each other to accomplish the desired tasks. This is known as object-oriented programming (OOP). You are only concerned with the OrthokonBoard class. The board starts with four red pieces on row 0 and four yellow pieces on row 3. A valid move consists of a player moving one of their pieces orthogonally or diagonally as far as it can go until it hits another piece or the edge of…arrow_forwardA Memory Matching Game in java code with a 4x4 grid of Squares that when you click on a square it shows a number. It would have to be 2 of each number 1-8 and if the two squares that are clicked match then the squares stay on the grid. If they do not match after picking 2 squares they flip back overarrow_forward
- Write a C++ program for a library management system that allows users to manage books. The program should include features to add books, remove books, and display the current book inventory. Each book has a title, author, publication year, and available quantity. The program should have the following functionalities: 1. A menu-based system allowing users to choose between adding a book, removing a book, and displaying the inventory. 2. When adding a book, the user should input the title, author, publication year, and quantity. 3. When removing a book, the user should input the publication year of the book to be removed. 4. Display the current inventory with details of each book, including title, author, publication year, and available quantity. Include comments in the code explaining its purpose, input and output details, and any complex logic. Use meaningful variable names, indentation, and white spaces for better readability. Test your program with sample data to ensure correct…arrow_forwardThis is Python! Instruction: The TidBit Computer Store (Chapter 3, Project 10) has a credit plan for computer purchases. Inputs are the annual interest rate and the purchase price. Monthly payments are 5% of the listed purchase price, minus the down payment, which must be 10% of the purchase price. Write GUI-based program that displays labeled fields for the inputs and a text area for the output. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to balance * rate / 12. The amount of principal for a month is equal to the monthly payment minus the interest owed. Your program should include separate classes for the…arrow_forwardIn Python IDLE: How would I write code for the two problems in the attached image?arrow_forward
- Using PYTHON You have been asked by a swimming pool company to create an application to give price quotes for swimming pool installations. This company installs 3 different types of pools: Circular pools Elliptical pools Square-shaped pools The price of each pool is the sum of the cost of the stonework that goes around the perimeter (aka circumference for round / elliptical shapes) of the pool and the installation of the pool (in square footage) plus the state sales tax. The diagram below shows the formulas for: the circumference / perimeter to determine the amount of stonework the area for each shape to determine the pool installation Here is the unit cost: price of stonework around the pool (per foot): $38.50 price of pool installation (per square foot): $53.80 tax rate: 6.35% Your main program will call a function getPoolType which will create a menu-driven prompt to ask the user the type of pool being considered. The response will be returned to the main…arrow_forwardWrite a java program to simulate a car insurance We have a problem, which is calculating the insurance price at a unified price for all categories.to solve this problem I want to calculate the insurance price in addition to the factors in the uml. For example, if the individual's age is from 18-25, the insurance rate will increase by 1% over the basic price, as well as the type of car and the gender of the person who wants insurance, for example, if a man also increases 1 % and so are all the factors. If you cannot write the entire program, it is not a problem. Write at least the equations for calculating the insurance or half of the program also You can put random numbers and random prices.arrow_forwardJAVA Programming: After Elon Musk decided to make a new business idea. He found a social network called "Friends.com". It has currently N registered users. Likewise in any social network many users can be friends. Elon Musk wants the world to be as super connected as possible, so he has this new way to suggest friendship to some pairs of users. He suggests user u to have a friendship with user v if they are not friends yet and there is a user w who is friends of both of them. Note that u, v and w are different users. Elon Musk is too busy with SpaceX these days, so he hired you to develop a JAVA program to count out how many friendship suggestions he has to send over his social network "Friends.com". Sample Input: 3 0111 1000 1000 Sample Output: 2arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
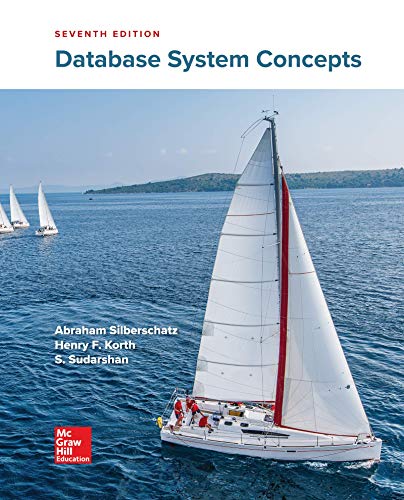
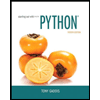
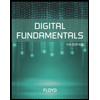
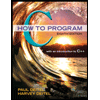
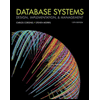
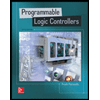