Write a Racket function "combine" that takes two functions, f and g, as parameters and evaluates to a new function. Both f and g will be functions that take one parameter and evaluate to some result. The returned function should be the composition of the two functions with f applied first and g applied to f's result. For example (combine add1 sub1) should evaluate to a function equivalent to (define (h x) (sub1 (add1 x))). You will need to use a lambda function to achieve your result. Essentially you want (combine f g) to evaluate to a lambda function with f and g embedded inside. You can test your combine with invocations such as ((combine add1 add1) 1), which should evaluate to 3 since the anonymous function returned by combine is being applied to 1. Note: This is an example of a "function closure". f and g are defined in the scope of combine, but the lambda embeds a copy of them in the lambda function that can be used outside of combine's scope. When creating a lambda function in Racket, anything in the enclosing scope can be captured in this way so that the lambda is functional outside of its defining scope.
Write a Racket function "combine" that takes two functions, f and g, as parameters and evaluates to a new function. Both f and g will be functions that take one parameter and evaluate to some result. The returned function should be the composition of the two functions with f applied first and g applied to f's result.
For example (combine add1 sub1) should evaluate to a function equivalent to (define (h x) (sub1 (add1 x))). You will need to use a lambda function to achieve your result. Essentially you want (combine f g) to evaluate to a lambda function with f and g embedded inside.
You can test your combine with invocations such as ((combine add1 add1) 1), which should evaluate to 3 since the anonymous function returned by combine is being applied to 1.
Note: This is an example of a "function closure". f and g are defined in the scope of combine, but the lambda embeds a copy of them in the lambda function that can be used outside of combine's scope. When creating a lambda function in Racket, anything in the enclosing scope can be captured in this way so that the lambda is functional outside of its defining scope.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

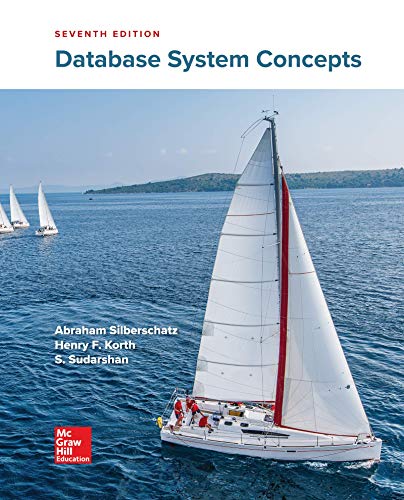
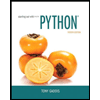
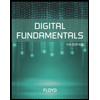
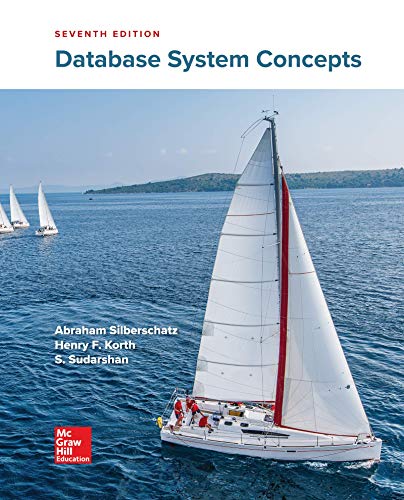
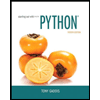
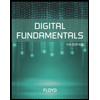
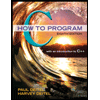
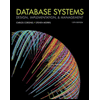
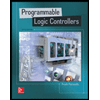