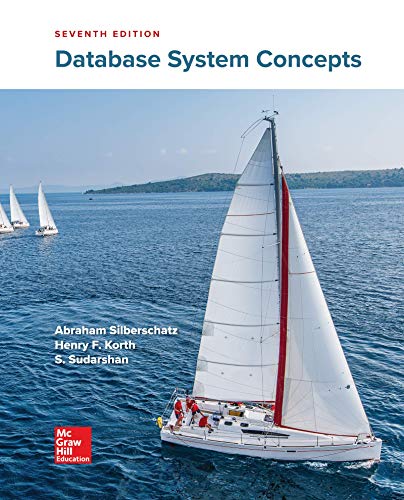
Concept explainers
This is C++ Programming
Given the following classes:
#include <iostream>
class Person
{
char* name;
public:
Person();
Person(const char*);
void setName(const char* nm);
void display(std::ostream&) const;
Person(const Person&); // copy constructor
Person& operator=(const Person& src); // copy assignment
~Person();
};
class Student : public Person
{
int no;
float* grade;
int ng;
void init(int, int, const float*);
public:
Student();
Student(int);
Student(const char*, int, const float*, int);
Student(const Student&); // copy constructor
Student& operator=(const Student& src); // copy assignment
~Student();
void display(std::ostream&) const;
};
write implementation for the following functions (you can call other functions from these classes if you want assuming that they are implemented):
~Person();
Person& Person::operator=(const Person& p);
Student(const Student&);

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- C:/Users/r1821655/CLionProjects/untitled/sequence.cpp:48:5: error: return type specification for constructor invalidtemplate <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;};} 48 | void sequence<Item>::sequence() : used(0), current_index(0) { } | ^~~~ line 47 template<class Item> line 48 void sequence<Item>::sequence() : used(0), current_index(0) { }arrow_forwardThe following struct types have been defined: typedef struct { int legs; char *sound; char *name; } pet_t; typedef struct { char *name; int age; pet_t *pet; } owner_t; A variable kid has been defined by: owner_t *kid; Functions get_owner and get_pet have the prototypes: owner_t *get_owner(char *,int,pet_t *); pet_t *get_pet (char *name, char *sound, int legs); Using kid, print a line like: Julie's pet parrot has 2 legs and says, "squawk".arrow_forwardQ2: Write a c++ program that implements the following UML diagram including functions and constructors' definitions. In addition to the user program code that declares object for each class and show how the derived class object uses the base class members. OOPCourse Student ID: int Grades[5]: int + setgrades(int arr[], int ): void + setID(int): int + setage(int): double + print():void + age:int + OOPstudent() OOPCourse OOPLAB OOPLab Reports[4]: int + setmarks(int arr[), int ): void + print():void + OOPLab()arrow_forward
- c++ programmingarrow_forwardC++ Language Create a UML Diagram for the Class Invoice with the provided code. Use <<constructor>> to define constructors, for each function's variable indicate whether it is a string or a integer. include <string> using namespace std; class Invoice{public:Invoice(string, string, int, int); void setPartNumber(string);string getPartNumber();void setPartDescription(string); string getPartDescription();void setQuantity(int); int getQuantity();void setPricePerItem(int); int getPricePerItem(); int getInvoiceAmount();private:string partNumber; string partDescription; int quantity; int pricePerItem; };arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
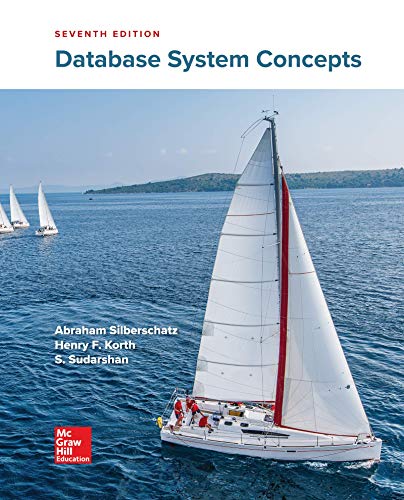
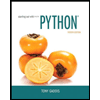
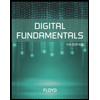
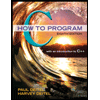
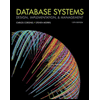
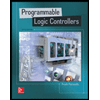