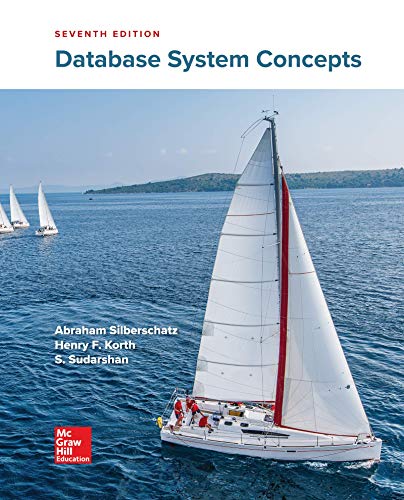
Concept explainers
write this in pseudocode
#4 – In pseudocode, write a for-loop that decreases from 100 to 2 by 2s. Inside the loop, print the value of the loop variable unless the value is from 60 to 80 (inclusive). Use only 1
if-statement. Do NOT write Python code!
Write your answer below:
#5 – Review the decision structure given below.
If myAnswer > 20 Then
myAnswer = myAnswer - 25
Else If myAnswer <= 5 Then
If myAnswer < 0 Then
myAnswer = 10
If myAnswer < 3 Then
myAnswer += 10
Else
myAnswer += 1
Else
myAnswer = 9
If myAnswer < 10 Then
myAnswer += 1
Display myAnswer
What are the results displayed when the decision structure above executes for each given value below:
If myAnswer = 6 then myAnswer =
myAnswer = 15 then myAnswer =
myAnswer = 30 then myAnswer =
myAnswer = 2 then myAnswer =
myAnswer = -1 then myAnswer =
#6 – 2-dimensional arrays can be thought of as containing rows and columns. What are the subscript values for a 2-dimensional array?
Enter them in the array below (first set given)
[0,0] [ ] [ ]
[ ] [ ] [ ]
[ ] [ ] [ ]
[ ] [ ] [ ]
[ ] [ ] [ ]

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

In Pseudocode
#7 – List 4 main data types we used in this course and describe the data that is stored in each one. List examples of variable names that make sense for each data type.
#8 – Debug this input validation function and fix it. There is a lot wrong with it!
Function Integer getNumberGreaterThanX(msg, x)
myInteger = getInteger(msg)
While myInteger < x
Display "The number must be < “, myInteger , “. Try again."
Input x
End While
End Function
Write the corrected function below:
#9 – Write a validation function based on the provided variables and call statement below. The validation function should return an Integer value greater than zero or print an error message until an acceptable value is entered.
Declare Integer positiveValue = 0
Declare String message = "Enter the year you were born (no negative numbers!)"
positiveValue = getNumberGreaterThanZero(message)
Write the function definition below:
In Pseudocode
#7 – List 4 main data types we used in this course and describe the data that is stored in each one. List examples of variable names that make sense for each data type.
#8 – Debug this input validation function and fix it. There is a lot wrong with it!
Function Integer getNumberGreaterThanX(msg, x)
myInteger = getInteger(msg)
While myInteger < x
Display "The number must be < “, myInteger , “. Try again."
Input x
End While
End Function
Write the corrected function below:
#9 – Write a validation function based on the provided variables and call statement below. The validation function should return an Integer value greater than zero or print an error message until an acceptable value is entered.
Declare Integer positiveValue = 0
Declare String message = "Enter the year you were born (no negative numbers!)"
positiveValue = getNumberGreaterThanZero(message)
Write the function definition below:
- C++ with Text Artsarrow_forwardValidating User Input Summary In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number. If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop. Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number. Instructions Ensure the file named GuessNumber.java is open. Write loops…arrow_forwardin xojo The statement For J = 7 To 13 causes the loop to iterate ______ timesarrow_forward
- Q1. FizzBuzz problem:- Write a program which return "fizz" if the number is a multiplier of 3, return "buzz" if its multiplier of 5 and return "fizzbuzz" if the number is divisible by both 3 and 5. If the number is not divisible by either 3 or 5 then it should just return the number itself? .arrow_forwardMUST BE IN PYTHON PROGRAMMING CODE!!!!! NO C++ or Java!! Directions: Design a program with a loop that lets the user enter a series of names (in no particular order). After the final person’s name has been entered, the program should display the name that is first alphabetically and the name that is last alphabetically. For example, if the user enters the names Kristin, Joel, Adam, Beth, Zeb, and Chris, the program would display Adam and Zeb. Additional requirements: Note that the possible set of input values can include negative, 0, or positive values, but not the sentinel value of -99. DESIGN HINT: • When determining the largest (or smallest) value in a set of numbers, remember to use variables to keep track of the current largest (or smallest) number found thus far. These variables can then be used as a comparison to each subsequent input number to see if the new number is larger (or smaller) than the current largest (or smallest) number and updated appropriately. At the end of all…arrow_forwardA retail company assigns a $5000 store bonus if monthly sales are more than $100,000; otherwise a $500 store bonus is awarded. Additionally, they are doing away with the previous day off program and now using a percent of sales increase to determine if employees get individual bonuses. If sales increased by at least 4% then all employees get a $50 bonus. If they do not, then individual bonuses are 0. Step 7: The final step in completing the pseudocode is to call all the modules with the proper arguments. Complete the missing lines. Module main () //Declare local variables Declare Real monthlySales Declare Real storeAmount Declare Real empAmount Declare Real salesIncrease //Function calls Call getSales(monthlySales) Call getIncrease(salesIncrease) Call ______________(_______________, _____________) Call ______________(_______________, _____________) Call ______________(_______________, _____________) End Modulearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
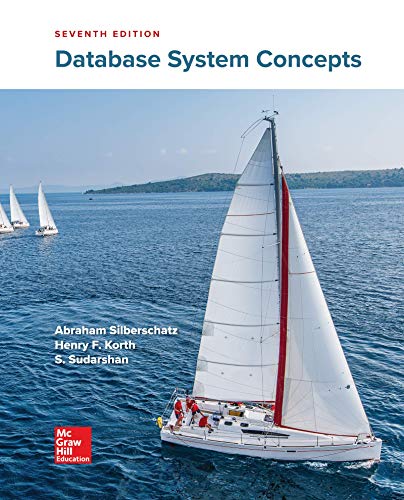
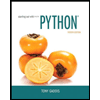
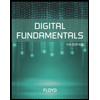
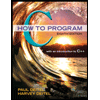
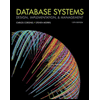
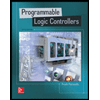