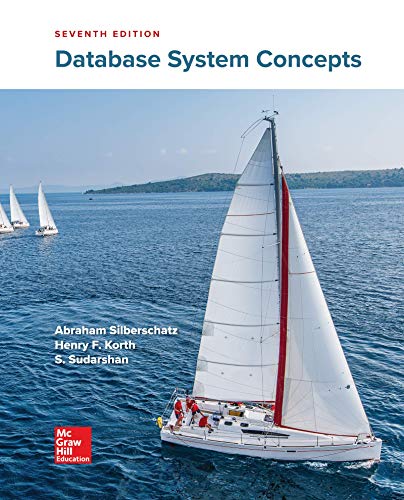
Write three statements to print the first three elements of array runTimes. Follow each statement with a newline. Ex: If runTime = {800, 775, 790, 805, 808}, print:
800
775
790
Also note: If the submitted code tries to access an invalid array element, such as runTimes[9] for a 5-element array, the test may generate strange results. Or the test may crash and report "
import java.util.Scanner;
public class PrintRunTimes {
public static void main (String [] args) {
Scanner scnr = new Scanner(System.in);
final int NUM_ELEMENTS = 5;
int [] runTimes = new int[NUM_ELEMENTS];
int i;
for (i = 0; i < runTimes.length; ++i) {
runTimes[i] = scnr.nextInt();
}
/* Your solution goes here */
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- use c code to develop the condition and function for the following items and then redevelop the code by adding the function as shown below, the original code has been given below. Hint: a)the function need to be made and develop and than add to the code 1. print Reverse 2. void reverseIt 3. void search Array 4.void search array 5. void array copy and void arrayDiff) b) the answer must be include and output and c code void printReverse(int array[], size); //prints the array reverselyvoid reverseIt(int array[], size); //reverses an arrayvoid searchArray(int array[], int size, int num);// returns the first index of the array whose value is num.//returns -1 if the number was not found in the array// optional for lab 6:void searchnArray(int array[], int size, int num, int n);// returns the nth index of the array whose value is num.//returns -1 if the number was not found in the arrayvoid arrayCopy (int arDest[], int arSource[], int commonSize);// copies cells from arSourc to arDestvoid…arrow_forwardProgramming language: Processing from Java Question attached as photo Topic: Use of Patial- Full Arraysarrow_forward1. Use the following array definition: int arr[10] = {1,9,7,0,8, 3,7,6,3,8 }; 2. Print all values stored in the array using the subscript operator (i.e.[ ]) 3. Print all values stored in the array using the dereference operator (i. e. *) 4. Print a table to show of the index, value, memory addressarrow_forward
- It's important to note that the name of an array by itself is really a pointer to the first element of the array. Therefore passing an array to function is simple since you can just specify the name of the array. Are these statements true?arrow_forwardC++ Get a value from the user and using a loop, search for the value in the array. Report all the index locations where it is found Report if it is not found anywhere This is part 4 of an ongoing assignment RandArray which consisted of assigning 20 integers with a random value. I am not sure how to write this out so it matches the output ( in the attached pictures ). I have attached the starter code to this problem as well as my code for part 3 which was correct. Thank you ( STARTER CODE ) #include <iostream>using namespace std; #include <cstdlib> // required for rand() int main(){// Put Part3 code here //Part 4// ask cout << "Enter value to find: ";// look through the array. // If it is found:// print // Found 55 at index 12 // if it is not found after looking at all the elements, // print // 0 is not found in randArray } _______________________________________________________________________ ( PART 3 CODE ) #include <iostream> using…arrow_forwardPlease write your own original code. Objective Create a program that initializes and works with string variables. Create a program named LastnameFirstname15.c, that does the following: Note: There is no user input for this program. Declare and initialize a char array that stores your first name in title case. Title case means the first letter is uppercase and the rest is lowercase. Ensure the size/length of the array is equal to the length of your name plus the null character.As an example, my first name is Edward, therefore the size of the array will be 7.char firstname[7] = "Edward"; Declare and initialize another char array that stores your last name in title case. Ensure the size/length of the array is equal to the length of your name plus the null character. Use the strlen function to get the lengths of each name and store them in separate variables. Calculate the total length of your name by adding the lengths of the first name and last name and store it in another…arrow_forward
- 1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forwardPlease type so that i can read you solutionarrow_forwardCan you help me please: have the program know when numbers out of the array boundaries are received and mitigate those problems. The given code intentionally induces numbers out of bounds and the student’s portion must correct for this. As always use a header file and implementation file to do those calculations. Remember not to change the given code below in any way Design and implement the class myArray that solves the array index out of bounds problem and also allows the user to begin the array index starting at any integer, positive or negative. Every object of type myArray is an array of type int. During execution, when accessing an array component, if the index is out of bounds, the program must terminate with an appropriate error message. Consider the following statements:myArray list(5); //Line 1 myArraymyList(2, 13); //Line 2myArray yourList(-5, 9); //Line 3The statement in Line 1 declares list to be an…arrow_forward
- C++ pleasearrow_forwardDefine the array printed below using the np.array() function, and assign it to the variable 'my_array' (only one line of code needed). [[0, 2, 4],[9, -6, 5],[0, 0, -10]]arrow_forwardSection A. Declare and initialize an array myChars containing the following characters: B, C, D, A, M, S and F.Remember to choose the correct data type for the array elements.Section B. Using a WHILE loop, output the elements of the array myChars so that they are displayed on thesame line and separated by a space. Use .length to get the length of the array.Section C. Change the last value of the array myChars to the letter X. Use .length in your code.Section D. Using a FOR loop, output the elements of the myChars array so that they are displayed on the sameline and separated by a dash (an extra dash at the end is okay). Use .length in your code.Section E. Declare an array of int of capacity 10 named thisArray.Section F. Use a FOR loop to populate the array thisArray with the following numbers 5, 10, 15, 20, 25. Thinkof a way to insert these numbers without actually writing “5” or “10” and so on, but by using the variable i ofyour FOR loop. NOTE that this is a partially-filled array and…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
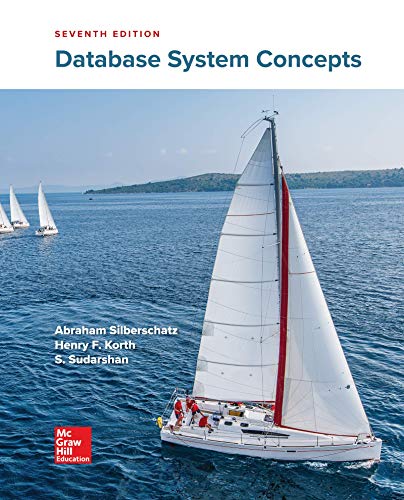
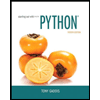
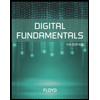
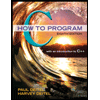
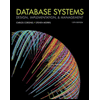
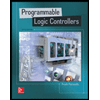