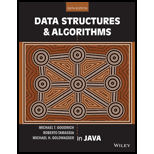
Concept explainers
Explanation of Solution
Implementation of norm(v):
The norm() method takes the input parameter of “vec” to return the Euclidean norm of “vec” array of coordinates.
//Function definition
public static double norm(double[ ] vec)
{
/*Call the norm() by passing the “vec” and “2” and return the result of computed value. */
return norm(vec,2);
}
Explanation:
In norm() method,
- It takes the input parameter of “vec”.
- Call the norm() method by passing the “vec” and “2” and return the result of computed value of p-norm value of “vec” array of coordinates.
Implementation of norm(v, p):
The norm() method takes the input parameter of “vec” and “pow” to return the p-norm value of “vec” array of coordinates.
//Function definition
public static double norm(double[ ] vec, int pow)
{
//Declare the variables
int sum = 0;
double exp = 1.0/pow;
//Loop executes until from “i” to "vec"
for (double i : vec)
//Add the "sum" and the power of number
sum += Math.pow(i,pow);
//Return the return
return Math.pow(sum, exp);
}
Explanation:
In norm() method,
- It takes the input parameter of “vec” and “pow”.
- Loop executes until the “vec” to add the sum and power of input.
- Return the computed p-norm value of “vec” array of coordinates.
Complete Program:
/**********************************************************
* Program demonstrates how to determine the Euclidean norm*
* for two-dimensional

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
Data Structures and Algorithms in Java
- Q1. Given a 2d grid map of '1's (land) and '0's (water),count the number of islands.An island is surrounded by water and is formed byconnecting adjacent lands horizontally or vertically.You may assume all four edges of the grid are all surrounded by water. Example 1: 11110110101100000000Answer: 1 Example 2: 11000110000010000011Answer: 3""" def num_islands(grid): count = 0 for i in range(len(grid)): for j, col in enumerate(grid[i]): if col == 1: dfs(grid, i, j) count += 1 Please code it.arrow_forwardQ. Given a 2d grid map of '1's (land) and '0's (water),count the number of islands.An island is surrounded by water and is formed byconnecting adjacent lands horizontally or vertically.You may assume all four edges of the grid are all surrounded by water. Example 1: 11110110101100000000Answer: 1 Example 2: 11000110000010000011Answer: 3""" def num_islands(grid): count = 0 for i in range(len(grid)): for j, col in enumerate(grid[i]): if col == 1: dfs(grid, i, j) count += 1 Please code it. .arrow_forwardIN PYTHON A tridiagonal matrix is one where the only nonzero elements are the ones on the main diagonal and the ones immediately above and below it.Write a function that solves a linear system whose coefficient matrix is tridiag- onal. In this case, Gauss elimination can be made much more efficient because most elements are already zero and don't need to be modified or added. As an example, consider a linear system Ax = b with 100,000 unknowns and the same number of equations. The coefficient matrix A is tridiagonal, with all elements on the main diagonal equal to 3 and all elements on the diagonals above and below it equal to 1. The vector of constant terms b contains all ones, except that the first and last elements are zero. You can use td to find that x1= −0.10557. The following code format should help: def td(l, m, u, b): '''Solve a linear system Ax = b where A is tridiagonal Inputs: l, lower diagonal of A, n-1 vector m, main diagonal of A, n vector u,…arrow_forward
- A vector is given by x = [9 –1.5 13.4 13.3 –2.1 4.6 1.1 5 –6.1 10 0.2]. Using conditional statements and loops, write a program that rearranges the elements of x in order from the smallest to the largest. solve code in matlabarrow_forwardGive a clear description of an efficient algorithm for finding the k smallest elements of a very large n-element vector. Compare its running time with that of other plausible ways of achieving the same result, including that of applying k times your solution for part (a). [Note that in part (a) the result of the function consists of one element, whereas here it consists of k elements. As above, you may assume for simplicity that all the elements of the vector are different.]arrow_forwardProblem Alice was given a number line containing every positive integer, x, where 1<=x<=n. She was also given m line segments, each having a left endpoint, l (1<=l<=n), a right endpoint, r (1<=r<=n), and an integer weight, w. Her task is to cover all numbers on the given number line using a subset of the line segments, such that the maximum weight among all the line segments in the chosen subset is minimal. In other words, let S=(l1,r1,w1),(l2,r2,w2),....,(lk,rk,wk), represent a set of k line segments chosen by Alice, max(w1,w2,...,wk) should be minimized. All numbers 1,2,....n should be covered by at least one of k the chosen line segments. It is okay for the chosen line segments to overlap. You program should output the minimized maximum weight in the chosen subset that covers all the numbers on the number line, or -1 if it is not possible to cover the number line. Input format The first line of the input contains an integer, n - denoting the range of numbers…arrow_forward
- A matrix is an array of numbers of size m by n (i.e., m x n). When we multiply 2 matrices, we multiply the matching numbers, then sum them up. Multiplying a matrix of size m x n with another matrix of size n x q will result in a matrix of size m x q. An example is shown in Figure 1: [ ? ? ? ? ? ? ] x [ ? ? ? ? ] = [ ?? +?? ?? + ?? ?? + ?? ?? + ?? ?? + ?? ?? + ?? ] Matrix 3 x 2 Matrix 2 x 2 Matrix 3 x 2 Figure 1: Multiplication of Matrix 3 x 2 and 2 x 2 Write a C++ program that multiplies 2 matrices and return the sum of the transposed matrix. Your program shall include the following: a) Define two 2 dimensional arrays, M and N that each represents a matrix of size 3 x 2 and a matrix of size 2 x 2. Request the user to enter the values for the 2 arrays using advanced pointer notations (refer to your slides for the list of array pointer notations). b) Create a function named multMatrix() that passes as arguments, the values of array M and N, multiplies them and store the results in a new…arrow_forwardGiven g = {(1,c),(2,a),(3,d)}, a function from X = {1,2,3} to Y = {a,b,c,d}, and f = {(a,r),(b,p),(c,δ),(d,r)}, a function from Y to Z = {p, β, r, δ}, write f o g as a set of ordered pairs.arrow_forwardComputer Science There is an n × n grid of squares. Each square is either special, or has a positive integer costassigned to it. No square on the border of the grid is special.A set of squares S is said to be good if it does not contain any special squares and, starting fromany special square, you cannot reach a square on the border of the grid by performing up, down,left and right moves without entering a cell belonging to S. 5 3 4 9 4 X 3 6 1 9 X 4 1 2 3 5 - Design an algorithm which receives an arbitrary n × n grid, runs in time poly-nomial in n and determines a good set of squares with minimum total cost.arrow_forward
- Given is a strictly increasing function, f(x). Strictly increasing meaning: f(x)< f(x+1). (Refer to the example graph of functions for a visualization.) Now, define an algorithm that finds the smallest positive integer, n, at which the function, f(n), becomes positive. The things left to do is to: Describe the algorithm you came up with and make it O(log n).arrow_forwardComputer Science Given an N x N matrix M with binary entries i.e every entry is either 1 or 0. You are told that every row and every column is sorted in increasing order. You are required to output a pair (i,j) with 1 <= i and j <= n corresponding to the entry of the matrix satisfying Mij = 1 and Mrs = 0 for all 1 <= r <= i and 1 <= s <= j except for Mij Informally this includes the entry of M = 1 and is closest to the top left corner. for example: M = [ 0 0 0 1 0 0 1 1 0 0 1 1 0 0 1 1] output is (2,3) or (1,4) M = [ 0 1 1 1 1 1 1 1 1] output could be (1,2) or (2,1) Design a divide and conquer algorithm, explain correctness and runtime of the algorithm.arrow_forwardThe Virtual Weight (VW) of an integer number (which consists of more than 1 digit) is the sumof the individual digits that are composing the number. For example, consider the followingtwo numbers; 5638 and 1145, then the VW of each is calculated as following:VW(5638) = 5+6+3+8 = 22VW(1145) = 1+1+4+5 = 11Then, the VW can be classified as L (mean Low) or H (means High) using a specific cut-offvalue. For example, if the cut-off value is 15, then the VW of the number 5638 is H (High),and the VW of the number 1145 is L because 22 > 15 and 11 ≤ 15 respectivelyWrite a C++ program that: reads a positive integer n (where 5≤n≤20) from the user. If the user enters invalidnumber, then the program should continue prompting until he/she enters a valid numberwithin the specified range. Then, the program should generate n random numbers using the built-in rand()function. Every time a random number is generated, the program will classify that number as Lor H using the above method. Use a…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
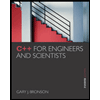