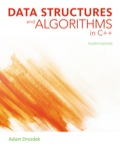
Concept explainers
String functions:
The string functions and their purpose is shown below:
- The function “strcpy(s1,s2)” copies string “s2” into “s1”.
- The function “strcat(s1,s2)” concatenates string “s2” on end of “s1”.
- The function “strlen(s1)” returns length of “s1”.
- The function “strchr(s1,ch)” would return a pointer to first presence of character “ch” in string “s1” .
Explanation of Solution
//(b)strcmp function
The function “strcmp()” compares two strings. It takes header pointer of two strings as function arguments, it checks until the string reaches null and compares both strings by comparing each character at corresonding position ...
Explanation of Solution
//(c) Strcat function
The function “Strcat()” copies data of “s2” to “s1”. To do so, it first reaches end of the string “s1” using recursive calls “Strcat(++s1, s2)”...
Explanation of Solution
//(d) Strchr function
The function “Strchr()” searches for a particular character in string. It iterates through character array and compares each character with search character, if it matches then the index of match is returned.
char* Strchr(char *s, char ch)
{
/*It checks for each character in string and compares it with that of the search string until the character array reaches null or it becomes empty. If a match is obtained, then store index of match*/
for ( ; *s != ch && *s != '\0'; s++);
//Return index of matched character
return *s == ch ? s : 0;
}
The main function defines two character array and tests the functions “Strlen()”, “Strcmp()”, “Strchr()”, “Strcat()” and displays the final result based on the return values of each functions
int main()
{
//Declare the variable
int ret;
//Declare character arrays
char str1[100] = "Drowning";
char *str2 = "Boat";
//Declare pointer of string
char *pch;
//Declare variables
int length ;
//Call the function "strlen()" and store the return value
length = Strlen(str1);
//Display first string
cout<<"String1: ";
puts(str1);
//Display second string
cout<<"String2: ";
puts(str2);
//Display the length of first string
cout<<"\nLength of String1 :"<< length;
//Call the function "Strcmp()" and store the return value of function
ret = Strcmp(str1,str2);
If return value is less than 0, then “String1” is less than “String2”. If return value is greater than 0, then “String2” is less than “String1”, else “String1” equals “String2”.
if(ret < 0)
{
//Display the result
cout<<"\nString1 is less than String2";
}
If return value is greater than 0, then “String2” is less than “String1”
else if(ret > 0)
{
//Display the result
cout<<"\nString2 is less than String1";
}
If “String1” equals “String2”, display the equal message
//"String1" equals "String2"
else
{
//Display the result
cout<<"\n String1 equals String2";
}
The function “Strchr()” is called with “str1” and search character “r” as argument, function’ sreturn value is stored, that is , the value of matched index...

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- a) Write a c++ program which contains a function isPalindrome, the function checks a stringusing pointers whether it is a palindrome or not and based on the decision return Ture orFalse. A string/word that reads the same backwards as forward is known as palindrome.E-g MADAM , POP , BOB etcb) Write a program that takes a char array (char *) that take your name as input, a charpointer point it and convert lower to upper and vice versa.arrow_forwardC++ Pointers must be used to solve the following problem. Write a function createPassword() with no return value to randomly select 8 capital letters from the alphabet. The function receives the address of the first characters of the password string as parameter. Hint: Declare a characters string that contains all the capital letters of the alphabet and use the index values of the characters in the string for random selection of characters, e.g.char alpha [27] = “ABCDEFGHIJKLMNOPQRSTUVWXYZ”;char *pAlpha = alpha; Display the password from the main() function. Write a function replaceVowels() with no return value to replace all the vowels (A, E, I, O, U) that have been selected with a symbol from the list ($ % @ # &). That is, A must be replaced by $, E replaced by %, I mustbe replaced by @, O replaced by #, and U must be replaced by &. The function receives the address of the first characters of the password string as parameter. Display the password agin from the main()…arrow_forwardIn C++ commas must be there. main.cpp #include <vector>#include <string>#include <iostream> using namespace std; // TODO: Write method to create and output all permutations of the list of names.void PrintAllPermutations(const vector<string> &permList, const vector<string> &nameList) { } int main() { vector<string> nameList; vector<string> permList; string name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. return 0;}arrow_forward
- What is the difference between void and NULL pointers .Give suitable examples in support of your answer.Give the differences in TABULAR form.arrow_forwardIn C Programming, write a program that creates an array of N names. Each name has 5 exam scores. Output the names with the highest and lowest average scores. No using of Struct, no using of Pointers and No Global Declaration.arrow_forwardPointers and references are fully equivalent True Falsearrow_forward
- The following code C++ uses pointers and produces two lines of output. What is the output? int v1 = 10; int v2 = 25; int* p1 = &v1; int* p2 = &v2; *p1 += *p2; p2 = p1; *p2 = *p1 + *p2; cout << v1 << " " << v2 << endl; cout << *p1 << " " << *p2 << endl; find line 1 and 2.arrow_forward(Pointers + Dynamic 1D Arrays) c++ program please use only pointers and dynamic 1D array and please don't use recursion and vector or otherarrow_forwardIN C PROGRAMMING LANGUAGE: Please write a pointer version of strncpy() named pstr_ncpy(char *dest, char *src, int n) which copies n characters from src and copies them into dest.arrow_forward
- USING FILE POINTERS IN C PLEASE . Write a program that prints a table of all the Roman numeral equivalents of the decimal numbers in the range 1 to 50. Knowing that the roman numbers (from 1 to 10) are as follows: I, II, III, IV, V, VI, VII, VIII, IX, X. Note that 50 is represented by L. COMMENT ALL OF YOUR STEPSarrow_forwardWrite a recursive function named “oddRec” that takes the address of the array and the number of elements as a parameter and returns the number of odd numbers in the array. (Do not use global variable)arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
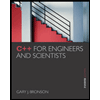
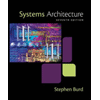