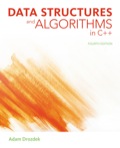
Concept explainers
Class Access Modifiers:
The feature of object oriented
There are three access specifiers used in the class to restrict the access of members inside the class. They are as follows:
- Public
- Protected
- Private
For classes and members, the default access specifier is “private”.
Sample code:
/* class Base defines the member functions and variables with appropriate access modifiers */
class Base
{
public:
//public members
protected:
// protected members
private:
// private members
};
Explanation of Solution
Private members:
From outside of a class, a member variable that is private could not be accessed, or viewed. Class could access members that are private and friend functions only.
By default, class members are private, for example in class given below “width” denotes a private member, which denotes that until a label is provided to a member, it would be assumed as a private member:
/* class lBox defines member functions “lsetWidth” and “lgetWidth”*/
class lBox
{
// Variable declarations
double lwidth;
public:
// Variable declarations
double length;
// Member function declarations
void lsetWidth( double lwid );
double lgetWidth( void );
};
The data is defined in private members, and related functions are defined in public section, so that it could be called from outside of a class, as shown in below program.
This program shows the usage of “Private” access modifier, in which member variables could not be accessed from outside of class.
// Select header files
#include <iostream>
using namespace std;
Declare the class lBox that defines the member functions “lsetWidth()” and “lgetWidth()” to set and return the width for box.
//Create the class
class lBox
{
public:
// Variable declarations
double length;
// Member function declarations
void lsetWidth( double lwid );
double lgetWidth( void );
private:
// Variable declarations
double lwidth;
};
Define the member function lgetWidth() to return width of box...
Explanation of Solution
Protected members:
A protected member function or variable denotes similarity to a private member. That is, from outside of a class, accessing a member variable or function that is protected is not possible.
- An additional benefit is that they can be accessed within child classes which are known as derived classes.
- In below example, “width” member could be accessed by the derived class “SmallBox” member function.
This program shows the usage of “Protected” access modifier, in which member variables could not be accessed from outside of a class, but it could be accessed within child classes which are termed as derived classes.
// Select header files
#include <iostream>
using namespace std;
Declare the class lBox that defines the variable “width” to set and return the width for box.
// class lBox defines “width” as a protected variable”
class lBox
{
protected:
// Variable declarations
double width;
};
Declare the class “SmallBox” that defines the member functions “lsetSmallWidth()” and “lgetSmallWidth()” to set and return the width for box...

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- What are the three responsibilities that come with working with classes that include member variables that are pointers and for which you are responsible?arrow_forwardSo, what are "static members" of a class, exactly? To what extent and when may you benefit from them?arrow_forwardDefine the term classes.arrow_forward
- Please explain the advantages of using interfaces rather than abstract classes.arrow_forwardThe pillar that allows you to build a specialized version of a general class, but violates encapsulation principles is ______________.arrow_forwardDescribe the most significant characteristics of classes and constructors that should be remembered.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
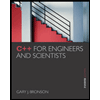
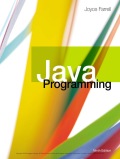