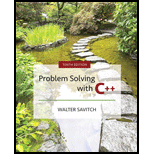
Structure in C++:
Structure is a user defined datatype that is used to combine data items of different data types.
Defining a Structure:
In order to define a structure, the “struct” statement is used. This statement defines a new data type with more than one member.
struct [structure tag] {
member definition;
member definition;
...
Member definition;
}[one or more structure variables];
Here, the “structure tag” is optional and each member definition is a normal variable definition. Specifying one or more structure variables at the end of the structure definition is also optional.
Given:
The type definition for “ShoeType” is as follows.
//Define a structure
struct ShoeType
{
//Declare variables
char style;
double price;
};
The following function declaration is given.
ShoeType discount(ShoeType oldRecord);
//Returns a structure that is the same as its argument,
//but with the price reduced by 10%.

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ (10th Edition)
- Given the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()?Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answer. Remove any space in your answer. If the accessing is wrong, answer INVALID (in all capital letters). void func( ______ );int main(){ double aData[6][4];func(&aData[4]);return 0;}arrow_forwardWrite a function example that takes two parameters and return one single parameter of same data type as output. Test that function in main function.arrow_forwardFor C++, why is it that reference values can change when given to a function? I understand that if you make a const reference parameter like: double functionname(const point& p1) then the function cannot attempt to make a change to the parameter.arrow_forward
- I need help in solving this question Question 10 Given the code segment below, what should be the data type of the formal parameter in the function prototype of func(), given the call from main()?Note that function prototypes need not include the identifier (name of the parameter), so do NOT put any variable name in your answeIf the accessing is wrong, answer INVALID (in all capital letters). void func( ______ ); int main() { double aData[6][4]; func(aData[6]); return 0; }arrow_forwardWhat is the overloading of an operator or a function? What good things could it do?arrow_forwardProvide an example to illustrate the differences between the background process of calling inline functions and that of a regular function.arrow_forward
- Justify your preference for user-defined over built-in functions.arrow_forwardGive an example of how the process of calling inline functions varies from the process of calling a standard function so that we may better understand the difference.arrow_forwardTemplate functions are considered to be an efficient alternative of function overloading. Justify this statement by comparing the pros and cons of both with real-world examples.arrow_forward
- Write a definition for a void-function that has two int value parameters and outputs to the screen the product of these arguments. Write a main function that asks the user for these two numbers, reads them in, calls your function, then terminates. c++arrow_forwardC++ PLEASE WRITE FULL FUNCTION DEFINITION for THE FUNCTION IN PART B Given the Class Definition for ClockType discussed extensively in class, write what would have to be added to the IMPLEMENTATION FILE for the Class ClockType to overload the “= =”, i.e., the comparison “equal-equal sign,” here: That is, write the FULL FUNCTION DEFINITION for THE FUNCTION associated with Class ClockType to overload the “= =” remembering the private members are: b) int hr; // that contains the hours int min; // that contains the minutes int sec; // that contains the secondsarrow_forwardExplain, with the use of an illustration, how the background process of invoking inline functions varies from that of calling a normal function.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
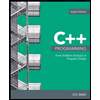
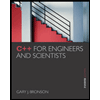