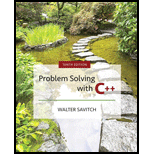
Concept explainers
Suppose your
class Automobile { public: void setPrice(double newPrice); void setProfit(double newProfit); double getPrice(); private: double price; double profit; double getProfit(); }; |
and suppose the main part of your program contains the following declaration and that the program somehow sets the values of all the member variables to some values:
Automobile hyundai, jaguar; |
Which of the following statements are then allowed in the main part of your program?
hyundai.price = 4999.99; jaguar.setPrice(30000.97); double aPrice, aProfit; aPrice = jaguar.getPrice(); aProfit = jaguar.getProfit(); aProfit = hyundai.getProfit(); if (hyundai == jaguar) cout << "Want to swap cars?"; hyundai = jaguar; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ - MyProgrammingLab
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Database Concepts (8th Edition)
Modern Database Management (12th Edition)
Digital Fundamentals (11th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Computer Systems: A Programmer's Perspective (3rd Edition)
- Mark the following statements as true or false. The member variables of a class must be of the same type. (1) The member functions of a class must be public. (2) A class can have more than one constructor. (5) A class can have more than one destructor. (5) Both constructors and destructors can have parameters. (5)arrow_forwardGiven the following code for a class named Employee, complete the following constructor public class Employee ( private int employeeID; private int SSN; public Emplyee(int employeeID, int SSN) {arrow_forwardSuppose that the class Pet has a field called name that is of the type String. Write an assignment statement in the body of the following constructor so that the name field will be initialized with the value of the constructor’s parameter. public Pet(String petsName) { }arrow_forward
- Build a class Sale with private member variablesdouble itemCost; // Cost of the itemdouble taxRate; // Sales tax rateand functionality mentioned below:● Write a default constructor to set the member variable itemCost to 0 andtaxRate to 0. ● Write a parameterized constructor that accepts the parameter for eachmemberSale( double cost, double rate) c++arrow_forwardIn C++ compile a program to fufill purpose mentioned below, not copied from internet please. Write a class named Employee that has the following member variables: name. A string that holds the employee’s name. idNumber. An int variable that holds the employee’s ID number department. A string that holds the name of the department where the employee works Position. A string that holds the employee's job title. The class should have the following constructors: A constructor that accepts the following value as arguments and assigns them to the appropriate member variables: employee’s name, employee’s ID number, department, and position. A constructor that accepts the following values as arguments and assigns them to the appropriate member variables: employee’s name and ID number. The department and position fields should be assigned as empty string (“”). A default constructor that assigns empty strings (“”) to the name, department and position member variables, and 0 to the…arrow_forwardProblem: Create a Fraction class with two private positive integer member variables numerator and denominator, one constructor with two integer parameters num and den with default values 0 and 1, one display function that print out a fraction in the format numerator/denominator in the proper form such as 2/3 or ½ . Note: 2/4 should be displayed as ½.arrow_forward
- Consider the following declarations: class xClass{public:void func();void print() const ; xClass ();xClass (int, double); private:int u;double w;};Sample Output: Program 2 and assume that the following statement is in a user program: xClass x;a. How many constructors does class xClass have?b. Write the definition of the default constructor of the class xClass so that the private member variables are initialized to 0.c. Write the definition of the constructor of the class xClass so that the private member variables u and w are initialized to the values passed in the functions.arrow_forwardMake a class called Employee with attributes for name, hours worked, and pay rate. Make a constructor that takes name, hours worked and pay rate as initializing parameters. Make a function in the class that will calculate their gross pay (multiply number of hours worked by pay rate). Make a function in the class called __str__ that prints out the name, hours worked and pay rate. Output a greeting to the user and then Prompt user to enter name, number of hours worked and pay rate. Make an instance of the employee class with the data they input. Now call the grosspay function to get the grosspay and print that out, Next call the __str__ function to print out the data in the instance (name, number of hours worked and pay rate)arrow_forwardModify the Loan class definition in the program ex91.cpp to include a new class called Bank in its definition as described below. // This will go to Loan.h file class Loan { public: Loan( ); Loan(Bank bank, ID id, float amount, float rate, int term); void set( ); float payment( ); void display( ); private: Bank bank; ID id; // assume an unique integer in three integer parts float amount; // $ amount of the loan float rate; // annual interest rate int term; // number of months, length of the loan }; The Bank class is defined as: // This will go to Bank.h file class Bank // Bank class definition { public: Bank( ); Bank(int bank_ID, CONTACT phone, CONTACT fax); private: int bank_ID; // 4 digit integer Contact phone; // object three integer pieces, # # #, # # #, # # # # Contact fax; // object three integer pieces, # # #, # # #, # # # # }; The class Contact has three integer parts, for Example: part1: 828,…arrow_forward
- Examine the following code and select the correct statements (choose all possibleoptions): class Bottle {Bottle() {}Bottle(WaterBottle w) {}}class WaterBottle extends Bottle {WaterBottle() { }WaterBottle(Bottle w) { } } a) The class Bottle defines two overloaded constructors.b) The class WaterBottle defines two overloaded constructors.c) The class compiles successfully—a base class can use reference variables of its derived class as method parameters.d) Bottle and WaterBottle cause syntax errors.e) The class compiles successfully - A derived class can use reference variables of its base class as method parameters.f) A base class can’t pass reference variables of its defined class, as method parameters in constructors.arrow_forwardProgram SpecificationUsing python, design a class named PersonData with the following member variables: lastName firstName address city state zip phone Write the appropriate accessor and mutator functions for these member variables. Next, design a class named CustomerData , which is derived from the PersonData class. The CustomerData class should have the following member variables: customerNumber mailingList The customerNumber variable will be used to hold a unique integer for each customer. The mailingList variable should be a bool . It will be set to true if the customer wishes to be on a mailing list, or false if the customer does not wish to be on a mail-ing list. Write appropriate accessor and mutator functions for these member variables. Next write a program which demonstrates an object of the CustomerData class in a program. Your program MUST use exception handling. You can choose how to implement the exception handling. Start your program with a welcome message Make sure…arrow_forwardWrite a program IN C# named SalespersonDemo that instantiates objects using classes named RealEstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each object’s data fields. First, create an abstract class named Salesperson. Fields include firstName and lastName; the Salesperson constructor requires both these values. Include properties for the fields. Include a method called GetName that returns a string that holds the Salesperson’s full name—the first and last names separated by a space. Then perform the following tasks: Create two child classes of Salesperson: RealEstateSalesperson and GirlScout. The RealEstateSalesperson class contains fields for the following: TotalValueSold - The total value sold in dollars (an int initialized to 0) TotalCommissionEarned - Total commission earned (a double initialized to 0) CommissionRate -…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
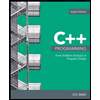
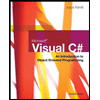