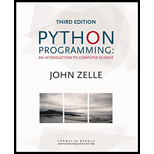
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 11, Problem 15PE
Program Plan Intro
Program to deal out a sequence of cards
Program plan:
- Import the required packages
- A class named “Deck” is defined.
- The function named “__init__()” is defined and inside it,
- Declare and initialize the required array variables.
- A loop is initialized to derive the values of cards.
- Calculate the store the values in “x” and “y”.
- Then the cards are appended in the array named “cardSpecs[]”.
- Declare another array named “cards[]”.
- A loop is initialized to append the cards in the array named “cards[]”.
- The function named “__shuffle()” is defined and inside it,
- Declare and initialize the required array variables.
- A loop is initialized to store the shuffled cards.
- Calculate the store the values in “x”.
- Then the cards are appended in a new array named “newList[]”.
- Remove that value of x from previous array named “cards[]”.
- Return the value stored in newList.
- The function named “shuffle()” is defined and inside it,
- Assign the value stored in “self.__shuffle()” to “self.cards”.
- The function named “dealCards()” is defined and inside it,
- Return the value stored in first “self.cards.pop()”.
- The function named “cardsLeft()” is defined and inside it,
- Return the length of the value stored in “self.cards()”.
- In the “main()” function,
- Declare the variable “deck” that creates the class named “Deck()”
- The function named “shuffle()” is called.
- A loop is initialized to store the shuffled cards.
- Print the output value that returned from “deck.dealCard()”.
- Call the function “main()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
pet shop wants to give a discount to its clients if they buy one or more pets and at least five other items. The discount is equal to 20 percent of the cost of the other items, but not the pets.Use a class Item to describe an item, with any needed methods and a constructor public Item(double price, boolean isPet, int quantity)An invoice holds a collection of Item objects; use an array list to store them. In the Invoice class, implement methods public void add(Item anItem)public double getDiscount()Write a program that prompts a cashier to enter each price and quantity, and then a Y for a pet or N for another item. Use a price of –1 as a sentinel. In the loop, call the add method; after the loop, call the getDiscount method and display the returned value.
Can you implement the Student class using the concepts of encapsulation? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first.
You are given a Student class in the editor. Your task is to add two fields:
● String name
● String rollNumber
and provide getter/setters for these fields:
● getName
● setName
● getRollNumber ● setRollNumber
Implement this class according to the rules of encapsulation.
Input #
Checking all fields and getters/setters
Output #
Expecting perfectly defined fields and getter/setters.
There is no need to add constructors in this class.
Write a class that represents a player in a game. The player should have a name, password,experience points, an inventory array of four strings, and a location x,y. Your class shouldhave mutator and accessor methods for all variables. For example: setName(), getName(). Itshould have a suitable display method. Mutators and accessors should be public but allvariables should be private. To implement get inventory, use string * getInv(); Use the scoperesolution operator to implement larger methods such as display(). Use in class methods forshorter methods such as setName(), getName(). (in C++)Example:void setName(string name){this->name = name;}string *getInv();...string* player::getInv(){return inventory;}Note that in the above method setName “this->” is required for disambiguation. If writtenvoid setName(string n){name = n;}“this->” is not required.Write a test program that creates three players and displays them.Example Output:This program generates three player objects and…
Chapter 11 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 11 - Prob. 1TFCh. 11 - Prob. 2TFCh. 11 - Prob. 3TFCh. 11 - Prob. 4TFCh. 11 - Prob. 5TFCh. 11 - Prob. 6TFCh. 11 - Prob. 7TFCh. 11 - Prob. 8TFCh. 11 - Prob. 9TFCh. 11 - Prob. 1MC
Ch. 11 - Prob. 2MCCh. 11 - Prob. 3MCCh. 11 - Prob. 4MCCh. 11 - Prob. 5MCCh. 11 - Prob. 6MCCh. 11 - Prob. 7MCCh. 11 - Prob. 8MCCh. 11 - Prob. 9MCCh. 11 - Prob. 10MCCh. 11 - Prob. 1DCh. 11 - Prob. 2DCh. 11 - Prob. 1PECh. 11 - Prob. 2PECh. 11 - Prob. 3PECh. 11 - Prob. 5PECh. 11 - Prob. 6PECh. 11 - Prob. 7PECh. 11 - Prob. 8PECh. 11 - Prob. 9PECh. 11 - Prob. 10PECh. 11 - Prob. 11PECh. 11 - Prob. 12PECh. 11 - Prob. 15PECh. 11 - Prob. 16PECh. 11 - Prob. 18PECh. 11 - Prob. 19PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Implement the design of the ECustomer class so that the following output is produced:[Your code should work for any number of products added in the setProductDetails method]# Write your codes here.print("Total E-Customer:", ECustomer.count)c1 = ECustomer("James")c1.setProductDetails("TV",35000,"Air Cooler", 9000)c2 = ECustomer("Mike")c2.setProductDetails("Mobile",20000,"Headphone",1200,"Fridge", 45000)c3 = ECustomer("Sarah")c3.setProductDetails("Headphone", 1200)print("=========================")c1.printDetail()print("=========================")c2.printDetail()print("=========================")c3.printDetail()print("=========================")print("Total E-Customer:", ECustomer.count)Output:Total E-Customer: 0=========================Name: JamesProducts: TV, Air CoolerTotal cost: 44000=========================Name: MikeProducts: Mobile, Headphone, FridgeTotal cost: 66200=========================Name: SarahProducts: HeadphoneTotal cost: 1200=========================Total E-Customer:…arrow_forward????????: Implement the design of the Pizza class so that the following output is produced: [Your code should work for any number of parameters added in the set_toppings_info method] # Write your codes here. print("Pizza Count:", Pizza.pizza_count) print("=======================") p1 = Pizza("Chicken") p1.set_toppings_info(25, 1, 4, 0) p1.display() print("------------------------------------") p2 = Pizza("Olives") p2.set_toppings_info(15, 1.5, 0, 0) p2.display() print("------------------------------------") p3 = Pizza("Sausage") p3.set_toppings_info(50, 5, 2, 0) p3.display() print("=======================") print("Pizza Count:", Pizza.pizza_count) Output: Pizza Count: 0 ======================= Toppings: Chicken 25 calories 1 g fat 4 g protein 0 g carbs ------------------------------------ Toppings: Olives 15 calories 1.5 g fat 0 g protein 0 g carbs ------------------------------------ Toppings: Sausage 50 calories 5 g fat 2 g protein 0 g carbs ======================= Pizza…arrow_forwardImplement solutions for the following methods: • getCourseSize() – returns the number of students registered in the course (not in the waitlist). It should maintain the public size variable that keeps track of the number of students registered. • getRegisteredIDs() – returns an array of int[], namely registered student id’s. The length of the array is the size (number of students) in the course. • getRegisteredStudents() – returns an array of type Student[], namely the registered Students. The length of the array is the current size (number of students) of the course. • getWaitlistedIDs() – returns an array of type int[], namely the ids of students in the waitlist. • getWaitlistedStudents() – returns an array of Students in the waitlist. public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) {…arrow_forward
- Complete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the total number of students in the course. Given classes: Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: toString() returns a String representation of the Student object.) Ex: If the following students and their GPA values are added to a course: Henry Cabot with 3.5 GPABrenda Stern with 2.0 GPAJane Flynn with 3.9 GPALynda Robison with 3.2 GPA then the program output is: Henry Cabot (GPA: 3.5) Brenda Stern (GPA: 2.0) Jane Flynn (GPA: 3.9) Lynda Robison (GPA: 3.2) Students: 4 course.java import java.util.ArrayList; // Class representing a studentpublic class Course { private ArrayList<Student> roster; // Collection of Student objects public Course() {…arrow_forwardPlease complete the task by yourself only in JAVA with explanation. Don't copy. Thank you. Only finish the (d) question, Thank you ! Using quicksort to sort an array of car objects by various criteria. Define a class Car as follows: class Car { public String make; public String model; public int mpg; // Miles per gallon } a) Implement a comparator called CompareCarsByMakeThenModel that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByMakeThenModel should return a value that will cause quicksort to sort an array of cars in ascending order (from smallest to largest) by make and, when two cars have the same make, in ascending order by model. b) Implement a comparator called CompareCarsByDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByDescendingMPG should return a value that will cause quicksort to sort an array of cars in descending order (from largest to smallest) by mpg. c)…arrow_forwardImplement the design of the Purchaser and AarongProducts classes so that the following code generates the output below: #Write your code here p1 = Purchaser('Alex Light') print('1.===============================') print(p1) product1 = AarongProducts('Decor', 'Photo-Frame') print('2.===============================') print(product1) print('3.===============================') print(AarongProducts.products) print('4.===============================') AarongProducts.include_products('Souvenirs',['Brass-Flowervase 2170', 'Terracotta-Vase 3500']) print(f'Updated Item List:\n{AarongProducts.products}') print('5.===============================') product2 = AarongProducts('Souvenirs', 'Terracotta-Vase', 2) print(product2) print('6.===============================') p1.create_invoice(product1, product2) print('7.===============================') product3 = AarongProducts('Decor', 'Candles', 5) print(product3) print('8.===============================') product4 = AarongProducts('Souvenirs', 'Brass…arrow_forward
- 1)The program should be written in JAVA. Create a "Car" class that keeps car ids and prices. And create a "Galleries" class that holds the car list for a particular gallery. In this class there should be methods for get / set and print for car name, car number and car list. Adding / Removing Cars to the List in This Class should have methods. And create another method to find and print the IDs of Cars with Car Segment equal to X. (print_id(X)). Car Prices are as follows according to the segments. 0$-19999$ -> Z20000$-29999$ -> Y30000$-44999$ -> T45000$-100000$ -> P Apply the Car list using "Singly Linked List"(Node, newNode, head).arrow_forwardWe have two classes; these classes are "Car" and "Price".The "car" class is a class that keeps the id and price of the car.If the "Price" class is, there will be get / set methods for the segment of the fee (For example Y segment Z segment), the number of cars, and the "Car" class list.In the "Price" class, there should be a print method to add / remove cars to the list and find the ids of Vehicles whose segments are equal to X.The segments are as follows. 0$ -15999$ -> Y Segment16000$-24999$ -> Z Segment25000$-50000$ -> T Segment It is necessary to use "Single Linked List".The program should be written in JAVA.arrow_forwardPlease complete the task by yourself only in JAVA with explanation. Don't copy. Thank you. Using quicksort to sort an array of car objects by various criteria. Define a class Car as follows: class Car { public String make; public String model; public int mpg; // Miles per gallon } a) Implement a comparator called CompareCarsByMakeThenModel that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByMakeThenModel should return a value that will cause quicksort to sort an array of cars in ascending order (from smallest to largest) by make and, when two cars have the same make, in ascending order by model.arrow_forward
- Please complete the task by yourself only in JAVA with explanation. Don't copy. Thank you. Using quicksort to sort an array of car objects by various criteria. Define a class Car as follows: class Car { public String make; public String model; public int mpg; // Miles per gallon } a) Implement a comparator called CompareCarsByMakeThenModel that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByMakeThenModel should return a value that will cause quicksort to sort an array of cars in ascending order (from smallest to largest) by make and, when two cars have the same make, in ascending order by model. b) Implement a comparator called CompareCarsByDescendingMPG that can be passed as an argument to the quicksort method from the lecture notes. CompareCarsByDescendingMPG should return a value that will cause quicksort to sort an array of cars in descending order (from largest to smallest) by mpg. c) Implement a comparator called…arrow_forwardAdd a method called multiplesOfFive to the Exercise class. The method must have a void return type and take a single int parameter called limit. In the body of the method, write a while loop that prints out all multiples of 5 between 10 and limit (inclusive) on a single line. For instance, if the value of limit were 15 then the method will print: 10 15 You can use the printEvenNumbers method that is already in the Exercise class as an example to help you work out how to complete this method. In the main method of the Main class, add a call on the Exercise object to your multiplesOfFive method that prints the multiples of 5 between 10 and 25. Add a method called sum to the Exercise class. The method must have a void return type and take no parameters. In the body of the method, write a while loop to sum the values 1 to 10 and print the sum once the loop has finished. In the main method of the Main class, add a call on the Exercise object to your sum method. Check that it prints: 55 Add…arrow_forward) Modify the BookStore and BookSearchEngine classes explained in the class to include the following additional methods and test them:a) A method returning the book with the lowest price in the library.b) A method searching the library for Books of a given author and returning an ArrayList of such Books. c) A method returning an ArrayList of Books whose price is less than a given number.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
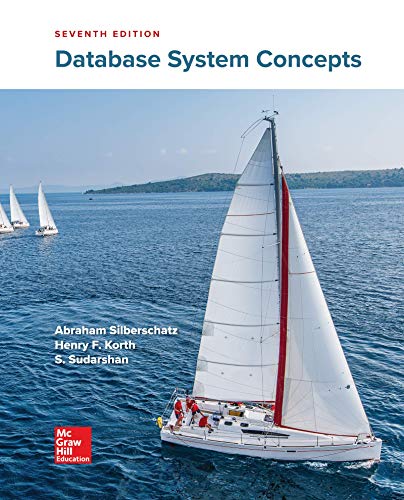
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
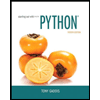
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
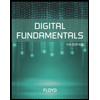
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
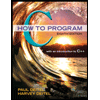
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
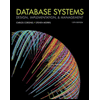
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
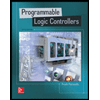
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License