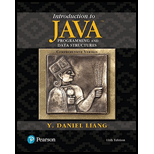
Show the output of following
public class Test {
public static void main(String[] args) {
new A();
new B();
}
}
class A {
int i = 7;
public A() {
setI(20);
System.out.println(“1 from A is ” + i);
}
public void setI(int i) {
this.i = 2 * i;
}
}
class B extends A {
public B() {
System.out.println(“i from B is ” + i);
}
public void setI(int i) {
this. i = 3 * i;
}
}

Trending nowThis is a popular solution!

Chapter 11 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (8th Edition)
Concepts Of Programming Languages
Modern Database Management (12th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
Starting Out with Java: From Control Structures through Objects (6th Edition)
- Trace and document every step of execution of the following program: public class Trace { public static void main(String[] args) { int[] num = {1, 3, 6, 9, 12 }; for (int i = 4; i >= 0; i--) { num[i] +=1; } num[0] *=num[4]; } }arrow_forwardWrite down the outputs of the following java program public class Exam { String X = "Programming"; double Y = 3; static int N; Exam ( ) { N++ ; } public static void main(String args[]) { Exam s1 = new Exam ( ); Exam s2 = new Exam ( ); System.out.println("1. " + (s1.Y)); System.out.println("2. " + s1.X.replaceFirst("r", "S")); System.out.println("3. " + s2.N); } }arrow_forwardpublic class Soru32{ // Returns length of LCS for X[0..m-1], Y[0..n-1] static void lcs(String X, String Y, int m, int n) { int[][] L = new int[m+1][n+1]; // Following steps build L[m+1][n+1] in bottom up fashion. Note // that L[i][j] contains length of LCS of X[0..i-1] and Y[0..j-1] for (int i=0; i<=m; i++) { for (int j=0; j<=n; j++) { if (i == 0 || j == 0) L[i][j] = 0; else if (X.charAt(i-1) == Y.charAt(j-1)) L[i][j] = L[i-1][j-1] + 1; else L[i][j] = Math.max(L[i-1][j], L[i][j-1]); } } // Following code is used to print LCS int index = L[m][n]; int temp = index; // Create a character array to store the lcs string char[] lcs = new char[index+1]; lcs[index] = '\u0000'; // Set the terminating character // Start from the right-most-bottom-most…arrow_forward
- public class Soru32{ // Returns length of LCS for X[0..m-1], Y[0..n-1] static void lcs(String X, String Y, int m, int n) { int[][] L = new int[m+1][n+1]; // Following steps build L[m+1][n+1] in bottom up fashion. Note // that L[i][j] contains length of LCS of X[0..i-1] and Y[0..j-1] for (int i=0; i<=m; i++) { for (int j=0; j<=n; j++) { if (i == 0 || j == 0) L[i][j] = 0; else if (X.charAt(i-1) == Y.charAt(j-1)) L[i][j] = L[i-1][j-1] + 1; else L[i][j] = Math.max(L[i-1][j], L[i][j-1]); } } // Following code is used to print LCS int index = L[m][n]; int temp = index; // Create a character array to store the lcs string char[] lcs = new char[index+1]; lcs[index] = '\u0000'; // Set the terminating character // Start from the right-most-bottom-most…arrow_forwardWhat will be the output of the following Java Program? public class Main { public static void main(String args[]) { int arr[][] = new int[4][]; arr[0] = new int[1]; arr[1] = new int[2]; arr[2] = new int[3]; arr[3] = new int[4]; int i, j, k = 0; for (i = 0; i < 4; i++) { for (j = 0; j < i + 1; j++) { arr[i][j] = k; k++; } } for (i = 0; i < 4; i++) { for (j = 0; j < i + 1; j++) { System.out.print(" " + arr[i][j]); k++; } System.out.println(); } }}arrow_forwardRe-write this program using Iterators but without changing the meaning and consequently producing the same output. class TwoDArray { public static void main(String args[]) { int i, j, k = 0, twoD[][] = new int[4][5]; for(i = 0; i < 4; i++) for (j = 0; j < 5; j++) twoD[i][j] = k++; for(i = 0; i < 4; i++) { for(j = 0; j < 5; j++) System.out.print(twoD[i][j] + " "); System.out.println(); } }arrow_forward
- Change Java code below so that it outputs a 9x9 board and not a 3x3 board. import java.util.*; public class Tic_Tac_Toe{ static String[] board;static String turn;static String checkWinner(){for (int a = 0; a < 8; a++) {String line = null; switch (a) {case 0:line = board[0] + board[1] + board[2];break;case 1:line = board[3] + board[4] + board[5];break;case 2:line = board[6] + board[7] + board[8];break;case 3:line = board[0] + board[3] + board[6];break;case 4:line = board[1] + board[4] + board[7];break;case 5:line = board[2] + board[5] + board[8];break;case 6:line = board[0] + board[4] + board[8];break;case 7:line = board[2] + board[4] + board[6];break;}//For X winnerif (line.equals("XXX")) {return "X";} // For O winnerelse if (line.equals("OOO")) {return "O";}} for (int a = 0; a < 9; a++) {if (Arrays.asList(board).contains(String.valueOf(a + 1))) {break;}else if (a == 8) {return "draw";}} // To enter the X Or O at the exact place on board.System.out.println(turn + "'s turn; enter…arrow_forwardWRITE A JAVA PROGRAM Methods can be used to define reusable code and organize and simplify code. Problem:- Suppose that you need to find the sum of integers from 5 to 15, from 16 to 30, and from 31 to 39, respectively. Define a public static sum method that take two int parameters -> (int start, int end) In the method, use for loop to add all the number from start to end. Later, invoke the sum inside the main method. Do the sum for each a) 5 to 15; b) 16 to 30; and c) 31 to 39 separately and later print the results. Output: Sum from 5 to 15 is 110 Sum from 16 to 30 is 345 Sum from 31 to 39 is 315arrow_forwardwhat is the problem with the following code. Give reason for your answer. public class MyClass\{ public static void main(String[] args) \{ int n[]={1,3,5,6} ; int b[]; b=n; \} \}arrow_forward
- Write a method (in C++), called CheckLetter. The method receives a letter as a parameter and returns whether the letter is a lowercase vowel (a, e, i, o, u) or not. Sample outputs are: The entered letter is: aa is a vowel. The entered letter is: bb is not a vowel.arrow_forwardShow the output of the following programs. (Hint: Draw a table and list the variables in the columns to trace these programs.) (java language)(a)public class Test {public static void main(String[] args) {for (int i = 1; i < 5; i++) {int j = 0;while (j < i) {System.out.print(j + " ");j++;}}}} (b)public class Test {public static void main(String[] args) {int i = 0;while (i < 5) {for (int j = i; j > 1; j--)System.out.print(j + " ");System.out.println("****");i++;}}} (c)public class Test {public static void main(String[] args) {int i = 5;while (i >= 1) {int num = 1;for (int j = 1; j <= i; j++) {System.out.print(num + "xxx");num *= 2;} System.out.println();i--;}}} (d)public class Test {public static void main(String[] args) {int i = 1;do {int num = 1;for (int j = 1; j <= i; j++) {System.out.print(num + "G");num += 2;} System.out.println();i++;} while (i <= 5);}}arrow_forwardWRITE THE BODY OF Java program - THE checkWinner METHOD BELOW SO THAT THE GAME STOPS WHEN SOMEONE WINS, OR WHEN THE BOARD IS FULL. AS SHOWN IN THE SAMPLE OUTPUT AT THE BOTTOM. Code: import java.util.Scanner; public class TicTacBoard { private char[][] board; // 2-D array of characters private char curPlayer; // the player whose turn it is (X or O) // Constructor: board will be size x size public TicTacBoard(int size) { board = new char[size][size]; // initialize the board with all spaces: for(int row=0; row < board.length; row++) for(int col=0; col < board[row].length; col++) board[row][col] = ' '; curPlayer = 'X'; // X gets the first move } public void playGame() { display(); do { takeTurn(); display(); }while(!checkWinner()); } /////// display //////// // Display the current status of the board on the // screen, using hyphens (-) for horizontal lines // and pipes (|) for vertical lines. public void…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
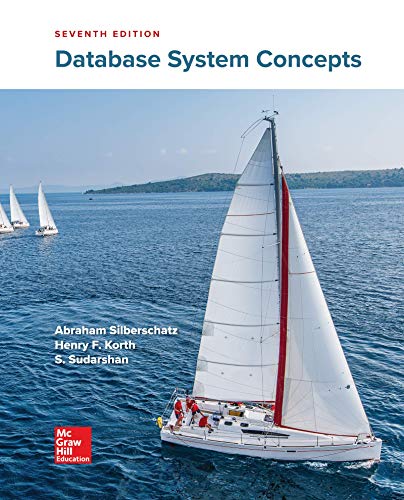
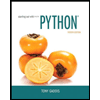
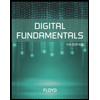
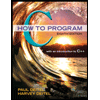
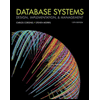
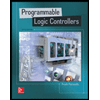