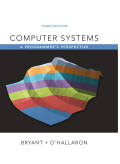
A.
Explanation of Solution
C code:
//Include Header file
#include <stdio.h>
#include "csapp.h"
//Define the maximum entry for blocked list
#define MAXIMUMSIZE 100
//Function declaration
int divideURI(char *URIName, char *hostName, char *portNumber, char *pName);
void parseBlockList(char *fName, char list[MAXIMUMSIZE][MAXLINE], int limit);
int blockedURIList(char *URIName, char list[MAXIMUMSIZE][MAXLINE]);
//Main function
int main(int argc, char **argv)
{
//Declare required variable
int i, listenfd, connfd;
int clientfd;
//Create socket
socklen_t clientlen;
//Create structure for client address
struct sockaddr_storage clientaddr;
//Create rio function
rio_t cRio, sRio;
char cbuffer[MAXLINE], sbuffer[MAXLINE];
ssize_t ssn, ccn;
char methodName[MAXLINE], URIName[MAXLINE], versionNo[MAXLINE];
char hostName[MAXLINE], portNumber[MAXLINE], pName[MAXLINE];
char block_list[MAXIMUMSIZE][MAXLINE];
int logFileDes;
char logFileBuffer[MAXLINE];
//Check command line arguments
if (argc != 2)
{
//Display message
fprintf(stderr, "usage: %s <port>\n", argv[0]);
fprintf(stderr, "use default portNumber 5000\n");
listenfd = Open_listenfd("5000");
}
else
{
listenfd = Open_listenfd(argv[1]);
}
//Open log files
logFileDes = Open("log.list", O_WRONLY | O_APPEND, 0);
//Set memory for block list
memset(block_list, '\0', MAXLINE * MAXIMUMSIZE);
//Call function for parse file
parseBlockList("block.list", block_list, MAXIMUMSIZE);
//Check condition
while (1)
{
/* wait for connection as a server */
clientlen = sizeof(struct sockaddr_storage);
//Call accept method
connfd = Accept(listenfd, (SA *) &clientaddr, &clientlen);
Rio_readinitb(&sRio, connfd);
/* Check URI Name full path */
if (!Rio_readlineb(&sRio, sbuffer, MAXLINE))
{
Close(connfd);
continue;
}
//Display the uri path
sscanf(sbuffer, "%s %s %s", methodName, URIName, versionNo);
/* Check blocked uri */
if (blockedURIList(URIName, block_list))
{
printf("%s is blocked\n", URIName);
Close(connfd);
continue;
}
//Display the visit uri
sprintf(logFileBuffer, "visit url: %s\n", URIName);
Write(logFileDes, logFileBuffer, strlen(logFileBuffer));
memset(hostName, '\0', MAXLINE);
memset(portNumber, '\0', MAXLINE);
memset(pName, '\0', MAXLINE);
//Declare variable
int res;
/* Check if the given uri is separate */
if ((res = divideURI(URIName, hostName, portNumber, pName)) == -1)
{
fprintf(stderr, "is not http protocol\n");
Close(connfd);
continue;
}
else if (res == 0)
{
fprintf(stderr, "is not a abslute request path\n");
Close(connfd);
continue;
}
//Connect server as a client
clientfd = Open_clientfd(hostName, portNumber);
Rio_readinitb(&cRio, clientfd);
//Send the first request
sprintf(sbuffer, "%s %s %s\n", methodName, pName, versionNo);
Rio_writen(clientfd, sbuffer, strlen(sbuffer));
printf("%s", sbuffer);
do
{
/* Call next http requests */
ssn = Rio_readlineb(&sRio, sbuffer, MAXLINE);
printf("%s", sbuffer);
Rio_writen(clientfd, sbuffer, ssn);
}
while(strcmp(sbuffer, "\r\n"));
//For server send reply back
while ((ccn = Rio_readlineb(&cRio, cbuffer, MAXLINE)) != 0)
Rio_writen(connfd, cbu...
B.
Explanation of Solution
C code:
#include <stdio.h>
#include "csapp.h"
//Define the maximum entry for blocked list
#define MAXIMUMSIZE 100
//Function declaration
int divideURI(char *URIName, char *hostName, char *portName, char *pathName);
void parseBlockList(char *fName, char list[MAXIMUMSIZE][MAXLINE], int limit);
int blockedURIList(char *URIName, char list[MAXIMUMSIZE][MAXLINE]);
void *proxyThreadFunction(void *vargp);
//Declare variable for blocked url list
static char bList[MAXIMUMSIZE][MAXLINE];
//Declare variable for log file fd
static int logFileDesc;
//Main function
int main(int argc, char **argv)
{
//Declare required variable
int listenfd;
//Create socket
socklen_t clientlen;
//Create structure for client address
struct sockaddr_storage clientaddr;
int *connfdp;
pthread_t tid;
//Check command line arguments
if (argc != 2)
{
//Display message
fprintf(stderr, "usage: %s <port>\n", argv[0]);
fprintf(stderr, "use default port 5000\n");
listenfd = Open_listenfd("5000");
}
else
{
listenfd = Open_listenfd(argv[1]);
}
//Open log files
logFileDesc = Open("log.list", O_WRONLY | O_APPEND, 0);
//Set memory for block list
memset(bList, '\0', MAXLINE * MAXIMUMSIZE);
//Call function for parse file
parseBlockList("block.list", bList, MAXIMUMSIZE);
//Check condition
while (1)
{
/* wait for connection as a server */
clientlen = sizeof(struct sockaddr_storage);
connfdp = Malloc(sizeof(int));
//Call accept method
*connfdp = Accept(listenfd, (SA *) &clientaddr, &clientlen);
//Generate new thread
Pthread_create(&tid, NULL, proxyThreadFunction, connfdp);
}
//Close log file descriptor
Close(logFileDesc);
}
//Function definition for proxy thread
void *proxyThreadFunction(void *vargp)
{
//Create thread id
pthread_t tid = Pthread_self();
Pthread_detach(tid);
int connfd = *(int*)vargp;
Free(vargp);
//Create rio function
rio_t cRio, sRio;
/* Declare required variable for client and server buffer */
char cBuffer[MAXLINE], sBuffer[MAXLINE];
ssize_t ssn, ccn;
/* Declare variable for method name, uri, version number, host name, port number and path name*/
char methodName[MAXLINE], URIName[MAXLINE], versionNumber[MAXLINE];
char hostName[MAXLINE], portName[MAXLINE], pathName[MAXLINE];
char logFileBuffer[MAXLINE];
int clientfd;
Rio_readinitb(&sRio, connfd);
/* Check URI Name full path */
if (!Rio_readlineb(&sRio, sBuffer, MAXLINE))
{
Close(connfd);
return NULL;
}
//Display the uri path
sscanf(sBuffer, "%s %s %s", methodName, URIName, versionNumber);
/* Check blocked uri */
if (blockedURIList(URIName, bList))
{
printf("Thread %ld: %s is blocked\n", tid, URIName);
Close(connfd);
return NULL;
}
//Print the log visit
sprintf(logFileBuffer, "Thread %ld: visit url: %s\n", tid, URIName);
Write(logFileDesc, logFileBuffer, strlen(logFileBuffer));
memset(hostName, '\0', MAXLINE);
memset(portName, '\0', MAXLINE);
memset(pathName, '\0', MAXLINE);
int res;
/* Check if the given uri is separate */
if ((res = divideURI(URIName, hostName, portName, pathName)) == -1)
{
fprintf(stderr, "tid %ld: not http protocol\n", tid);
Close(connfd);
return NULL;
}
else if (res == 0)
{
fprintf(stderr, "tid %ld: not a abslute request path\n", tid);
Close(connfd);
return NULL;
}
//Connect server as a client
clientfd = Open_clientfd(hostName, portName);
Rio_readinitb(&cRio, clientfd);
//Send the first request
sprintf(sBuffer, "%s %s %s\n", methodName, pathName, versionNumber);
Rio_writen(clientfd, sBuffer, strlen(sBuffer));
printf("tid %ld: %s", tid, sBuffer);
do
{
/* Call next http requests */
ssn = Rio_readlineb(&sRio, sBuffer, MAXLINE);
printf("Tid %ld: %s", tid, sBuffer);
Rio_writen(clientfd, sBuffer, ssn);
} while(strcmp(sBuffer, "\r\n"));
//For server send reply back
while ((ccn = Rio_readlineb(&cRio, cBuffer, MAXLINE)) != 0)
Rio_writen(connfd, cBuffer, ccn);
�...

Trending nowThis is a popular solution!

Chapter 12 Solutions
EBK COMPUTER SYSTEMS
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
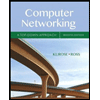
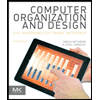
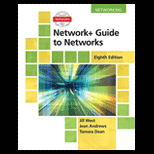
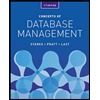
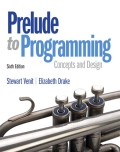
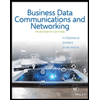