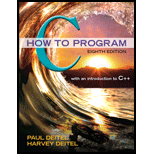
Program plan:
- Item, start variablesare used for input. There is structure listnode having data, nextPtr member variables which represents the linked list node.
- void insert(node **head, int value) function inserts the node in the a linked list.
- node *concat(node *flist, node *slist) function concat the two linked list and return the resultant linked list.
- void printList(node *head) function display the contents of the linked list.
Program description:
The main purpose of the program is to perform the concatenation of two linked list by implanting the concept which is same as strcat() function of string.

Explanation of Solution
Program:
#include <stdio.h> #include <conio.h> #include <alloc.h> #include <ctype.h> //structure of node of the linked list typedefstruct listnode { int data; struct listnode *nextPtr; } node; //function to insert a node in a linked list void insert(node &head,int value); //recursive function to concate the linked list node *concat(node *flist, node *slist); //function to print the content of linked list void printList(node *head); //main starts here void main() { int item; node *flist,*slist; clrscr(); //initialization of start node of linked list flist =NULL; slist =NULL; //loop to getting input from the user for first linked list while(1) { printf("\nEnter value to insert in a First List: 0 to End "); scanf("%d",&item); //check condition to terminate while loop if(item ==0) break; //insert value in a linked list insert(&flist, item); } //loop to getting input from the user for second linked list while(1) { printf("\nEnter value to insert in a Second List: 0 to End "); scanf("%d",&item); //check condition to terminate while loop if(item ==0) break; //insert value in a linked list insert(&slist, item); } // print the contents of linked list printf("\n Content of First List are as follows : "); printList(flist); // print the contents of linked list printf("\n Content of Second List are as follows : "); printList(slist); //call the function to reverse the linked list // and store the address of first node flist = concat(flist, slist); //print the contents of concated list printf("\n Concatenated List contents are as follows : "); printList(flist); getch(); } //function definition to insert node in a linked list void insert(node &head,int value) { node *ptr,*tempnode; //memory allocation for the new node ptr = malloc(sizeof(node)); //copy the value to the new node ptr->data = value; //set node's pointer to NULL ptr->nextPtr =NULL; //if the list is empty if(*head ==NULL) //make the first node *head = ptr; else { //copy the address of first node tempnode =*head; //traverse the list using loop until it reaches //to the last node while(tempnode->nextPtr !=NULL) tempnode = tempnode->nextPtr; //make the new node as last node tempnode->nextPtr = ptr; } } //function defintion to concat the list node *concat(node *flist, node *slist) { node *ptr; //check if list is empty if(flist ==NULL|| slist ==NULL) { printf("\none of the lists is empty"); //return the node returnNULL; } else { //store first node address ptr = flist; //traverse list until last node is not encountered while(ptr->nextPtr !=NULL) ptr = ptr->nextPtr; //store the address of first node of second list ptr->nextPtr = slist; } //return new list return flist; } //function definition to display linked list contents void printList(node *head) { node *ptr; //stores the address of first node ptr = head; //traverse the list until it reaches to the NULL while(ptr !=NULL) { //print the content of current node printf("%d ", ptr->data); //goto the next node ptr = ptr->nextPtr; } }
Explanation:In the above code, a structure is created which represents the node of the linked list. Two starting nodes are initialized which contains the address of first node of each list. User is asked to enter the values for first linked list and linked list is created using insert() function by passing the starting pointer and value. This process is repeated to create the second linked list. printList() function is used to display the contents of both lists. Both lists are concatenated using the concat() function by passing the both list. In this function first list is traversed up to last node and then last node pointer points to the first node of second list. Starting node address is returned and stored in the pointer. Finally, concatenated list is displayed using printList() function.
Sample output:
Want to see more full solutions like this?
Chapter 12 Solutions
C How to Program (8th Edition)
- - in this exercise, please do not include and use string class. The function is using only array notation and manipulation.- string functions such as strlen is not allowed.- it should not have multiple return statements in the same function- there should be no global variable.- the function should not traverse the arrays more than once (e.g. looping through the array once only) A C++ PROGRAM named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become{'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forwardUsing the following instruction below, write a header class for unorderedLinkedList with a function of search, insertFirst, insertLast and deleteNode. Use the library to write a program to show an operation on an unordered linked list. write in C++ program. - Include the unorderedLinkedList.h library in the codes - Initialize a list1 and list2 as unorderedLinkedList type - Declare a variable num as integer data type - Print out an instruction for user to input a numbers ending with -99 - Get a numbers from user input - Use a while loop to insert the numbers into list1 ending with -99 - Print out the current elements in List 1 - Assign list 2 to list 1 - Print out the elements in list 2 - Print the length of list 2 - Get user input for the number to be deleted - Delete the number requested by user in list2 - Print out the elements in the list after delete operation - Print the length of list 2 - Declare the intIt variable to be linked list iterator - Print out the elements in List 1…arrow_forward5(b) Give the two arrays that will be merged by the final step of mergesort on [8, 2, 1, 4, 5, 3, 7, 9].arrow_forward
- Using the following instruction below, write a header class for orderedLinkedList with a function of search,insert, insertFirst, insertLast and deleteNode. Use the library to write a c++ program to show an operation on an ordered linked list. - include the orderedLinkedList.h library in the codes - Create main function and include your information details - initialize a list1 and list2 as orderedLinkedList type - Declare a variable num as integer data type - Print out an instruction for user to input a numbers ending with -1 - Get a numbers from user input - Use a while loop to insert the numbers into list1 ending with -1 - Assign the list2 to list1 - Print out the current elements in List 1 and 2 - Get user input for the number to be deleted - Delete the number requested by user in list2 - Print out the elements in the list1 and list2 after delete operationarrow_forwardThe question should be done in C++, please give explanation and running result. 1. For this question, we need to provide more information about where the mismatch of the text file is happening. Write a recursive function called list_mismatched_lines that takes 2 filenames as input arguments and displays to the screen all mismatched lines in those files. This function should use hashing techniques and shall not compare strings to detect mismatch. The signature of this function should be: void list_mismatched_lines(std::string file1, std::string file2); In file1.txt, it contains "My dear C++ class. I hope that you enjoy this assignment. " In file2.txt, it contains "My dear C++ class. I hope that you like this assignment. " Example: Running the following line of code, should print to the screen the mismatched lines only, from both files. list_mismatched_lines(file1, file2); The following output should be seen on the screen: file1.txt: I hope that you enjoy this assignment.file2.txt:…arrow_forwardWhat's the distinction between void and NULL pointers? Give appropriate examples to back up your answer. Give the distinctions in TABULAR form.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
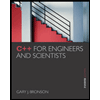
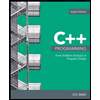