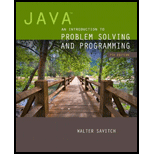
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 13PP
Program Plan Intro
Birds Survey
Program Plan:
- Import required package.
- Define “BirdSurvey” class.
- Create object “headNode” from “ListNode”.
- Create constructor for “BirdSurvey” class.
- Define the method “getReport()”.
- Compute position of node.
- Check condition using “while” loop.
- Display bird name and count.
- Define the method “computeLength()”.
- Set count to “0”.
- Set “position” to “headNode”.
- Check condition using “while” loop.
- Increment count
- Finally returns count value.
- Define the method “addANodeToStart” which is used to add bird name at start of list by using “ListNode” class.
- Define the method “add” with the argument of “bird”.
- If head node is null, then add bird to the front of list.
- Otherwise
- Compute given bird name by calling the method “find” and then store result to “n”.
- If “n” is null, then increment count value.
- Otherwise, find the last node in the list.
- Add in the node by using “ListNode” class.
- Define the method “getCount” with the argument of “bird”.
- Declare required variable.
- Create list for nodelist.
- If the bird is not on the list, then assign result of count to “0”.
- Otherwise, compute the result associated with bird species.
- Finally returns the value of result.
- Define the method “onList” with the argument of “t”.
- This method is used to check whether target “t” is on the list or not
- Define the method “find” with the argument of “t”.
- Assign required variables.
- Check condition using “while” loop.
- Define inner node class “ListNode”.
- Declare required variables.
- Create parameterized constructor for “ListNode” class.
- Assign values to required variables.
- Define main function.
- Create object “bs” from “BirdSurvey” class.
- Create object for scanner class.
- Display statement.
- Assign “moreValue” to “true”.
- Check condition using “while” loop.
- Read bird name from user.
- If the bird name is “done”, then set “moreValue” to “false”.
- Otherwise, add the bird name to “bs”.
- Display the birds report by calling the method “getReport”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Given the MileageTrackerNode class, complete main() in the MileageTrackerLinkedList class to insert nodes into a linked list (using the insertAfter() method). The first user-input value is the number of nodes in the linked list. Use the printNodeData() method to print the entire linked list. DO NOT print the dummy head node.
Ex. If the input is:
3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18
the output is:
2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18
public class MileageTrackerNode { private double miles; // Node data private String date; // Node data private MileageTrackerNode nextNodeRef; // Reference to the next node
public MileageTrackerNode() { miles = 0.0; date = ""; nextNodeRef = null; }
// Constructor public MileageTrackerNode(double milesInit, String dateInit) { this.miles =…
There’s a somewhat imperfect analogy between a linked list and a railroad train, where
individual cars represent links. Imagine how you would carry out various linked list
operations, such as those implemented by the member functions insertFirst(),
removeFirst(), and remove(int key) from the LinkList class in this hour. Also implement
an insertAfter() function. You’ll need some sidings and switches. You can use a
model train set if you have one. Otherwise, try drawing tracks on a piece of paper and
using business cards for train cars.
Programming in Java.
What would the difference be in the node classes for a singly linked list, doubly linked list, and a circular linked list? I attached the node classes I have for single and double, but I feel like I do not change enough? Also, I use identical classes for singular and circular node which does not feel right. Any help would be appreciated.
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- The implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forwardGiven the following definitions that we have used in class to represent a doubly-linked, circular List with a dummy-header node that contains data of type "struct Student". typedef struct { char *name; char *major; } Student;typedef struct node { Student *data; struct node *next; struct node *prev; } Node;typedef struct { Node *header; int size; } LinkedList;LinkedList *roster; Here is a picture of what the Linked List might look like, although you should answer the following question for a general list (ie, that does not necessarily contain 3 data items). What TYPE of data is the following: *roster Group of answer choices int int * Node Node * Student Student * char char * This is legal code, but it does not match any of the types listed above. This is illegal code, and would produce a syntax error No hand written solution and no imagearrow_forwardGiven the following definitions that we have used in class to represent a doubly-linked, circular List with a dummy-header node that contains data of type "struct Student". typedef struct { char *name; char *major; } Student; typedef struct node { Student *data; struct node *next; struct node *prev; } Node; typedef struct { Node *header; int size; } LinkedList; LinkedList *roster; Here is a picture of what the Linked List might look like, although you should answer the following question for a general list (ie, that does not necessarily contain 3 data items). What TYPE of data is the following: roster->header->data->next->next Group of answer choices int int * Node Node * Student Student * char char * This is legal code, but it does not match any of the types listed above. This is illegal code, and would produce a syntax error.arrow_forward
- 1. According to the following LinkedList, write pseudo code for the question belowHow do you insert a node with the data, "Brandon" between the node of "Chu" and the node of "Bethany"? 2.Assume that there is a linked list that connected several names.How do you set up a "while" loop to print the whole list? 3.What is the advantage of using a LinkedList over an ArrayList?arrow_forwardImplement a class “LinkedList” which has two private data members head: A pointer to the Node class length: length of the linked listImplement the following private method: 1. bool InsertHead(int data); // Use InsertAt FunctionInsert at the start of the linked list. Return true2. bool InsertEnd(int data); // Use InsertAt FunctionInsert at the end of the linked list. Return true3. bool RemoveAt(int index);Remove and delete the node at position “index”. Return true if successful, otherwise, false. this in c++arrow_forwardAdd a method findMiddle() that finds the middle node of a doubly linked list by link hopping, without relying on explicit knowledge of the size of the list. Counting nodes is not the correct way to do this. In the case of an even number of nodes, report the node slightly left of center as the middle. You can assume the list has at least one item. Your findMiddle() code will be located in DoublyLinkedList.java. If you’re using size(), you’re doing it wrong. Starter code for the findMiddle() method: // Janet McGregor 4/20/2053 public E findMiddle() { Node<E> middleNode = header.next; Node<E> currentNode = header.next; // the rest of your code goes here return middleNode.element; } You will test findMiddle() with the following three tests cases. Sample program output: Finding the middle of the list: (1, 2, 3, 4, 5, 6, 7) The middle value is: 4 Finding the middle of this list: (1, 2, 3, 4) The middle value is: 2 Finding the middle of this…arrow_forward
- Create a simple java program with a linked list that will hold 5 nodes (names of your teacher in the CICS this semester including me, V. Agcaoili). In the program, show how to add nodes by adding a name of faculty from other College as the third element in the linked list. Show also how to access the node(name) V.Agcaoili. Also, show how to change/update nodes by changing the element V.Agcaoili into V.Sinco. Finally, show how to remove nodes by removing the name of the faculty who is not from the CICS Department.arrow_forwardYou might have heard about the "Guess the Word Game", so in this game the user has to guess the letter(s) of the word one by one. There are n chances given to guess its letters where n is the number of letters in that word. You have to implement given scenario with the help of “Doubly Linked List”. Create two linkedlist, first linkedlist will be containing of complete word e.g. ELEPHANT and second linkedlist will be containing of word but having some blank letters e.g. _LEP_AN_. You have to ask user for input for every letter and insert into second linkedlist as follows: Step 1: Letter 1: Insert into second linkedlist on first place and compare 1st value of first and second linkedlist if same then go to step 2 else go to step 1 Step 2: Letter 2: Insert into second linkedlist on fifth place and compare 5th value of first and second linkedlist if same then go to step 3 else go to step 2 Step 3: Letter 3: Insert into second linkedlist on last place and compare last value of…arrow_forwardYou may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next. We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as: 1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index() Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null. 4. delete_at_start() 5. delete_at_end() . 6. display() the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display(). hint on how to start thee code # Initialize the Node class Node: def __init__(self, data): self.item = data…arrow_forward
- Write a method replace to be included in the class KWLinkedList (for doubly linked list) that accepts two parameters, searchItem and repItem of type E. The method searches for searchItem in the doubly linked list, if found then replace it with repItem and return true. If the searchItem is not found in the doubly linked list, then insert repItem at the end of the linked list and return false. Assume that the list is not empty. You can use ListIterator and its methods to search the searchItem in the list and replace it with repItem if found. Do not call any method of class KWLinkedList to add a new node at the end of the list. Method Heading: public boolean replace(E searchItem, E repItem) Example: searchItem: 15 repItem: 17 List (before method call): 9 10 15 20 4 5 6 List (after method call) : 9 10 17 20 4 5 6arrow_forwardGiven the following definition of a single linked list, write a method that calculates and returns the sum of even integers in a linked list. public class SLL<E> { private static class Node<E>{ private E data; private Node<E> next; private Node(E dataItem) { data = dataItem; next = null; } private Node(E dataItem, Node<E> nodeRef) { data = dataItem; next = nodeRef; } } private Node<E> head = null; private int size = 0; }arrow_forwardAdd a new public member function to the LinkedList class named reverse() which reverses the items in the list. You must take advantage of the doubly-linked list structure to do this efficiently as discussed in the videos/pdfs, i.e. swap each node’s prev/next pointers, and finally swap headPtr/tailPtr. Demonstrate your function works by creating a sample list of a few entries in main(), printing out the contents of the list, reversing the list, and then printing out the contents of the list again to show that the list has been reversed. Note: your function must actually reverse the items in the doubly-linked list, not just print them out in reverse order! Note: we won't use the copy constructor in this assignment, and as such you aren't required to update the copy constructor to work with a doubly-linked list. This is what I have so far but its not working! template<class ItemType>void LinkedList<ItemType>::reverse(){ Node<ItemType>*curPtr,*prev,*next;…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
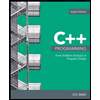
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning