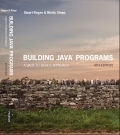
Explanation of Solution
Method definition:
//All the sub-lists of the given list of Strings gets printed.
/*elements!= null and elements contains no duplicates are the pre-condition*/
//method definition
public static void subsets(List<String> elements)
{
//define the list
List<String> chosen = new ArrayList<String>();
//call the method explore
explore(elements, chosen);
}
/*Helper recursive function defined to explore all the sub list from the given list of elements, choice is made assuming the given list of string is already chosen*/
//method definition
private static void explore(List<String> elements, List<String> chosen)
{
// base case; nothing left to choose
//validate whether the elements are empty
if (elements.isEmpty())
{
//display the chosen
System.out.println(chosen);
}
else
{
//define the choice that is for first element
String first = elements.remove(0);
// two explorations: one with this first element, one without
//add the first element
chosen.add(first);
//call the explore method
explore(elements, chosen);
//remove the element
chosen.remove(chosen.size() - 1);
//call the method
explore(elements, chosen);
// backtrack! 1st element is put back
elements...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
EBK BUILDING JAVA PROGRAMS
- Write a program in python with a function isPal(L), where L is a list of integers, and the function returns True if the list is a palindrome, False otherwise. For example [5, 2, 9, 9, 2 5] is a palindrome. Use the reverse() method of list and check if the reversed list is the same as the original list.arrow_forwardImplement a recursive function void deleteMax() on the IntList class (provided). The function will delete from the IntList the IntNode containing the largest value. If there are multiple nodes containing this largest value, only delete the 1st one. Be careful not to cause any memory leaks or dangling pointers. You may NOT use any kind of loop (must use recursion). You may NOT use global or static variables. You may NOT use any standard library functions. Ex: list: 5->7->1->16->4->16->3 list.deleteMax(); list: 5->7->1->4->16->3 IntList.h #ifndef __INTLIST_H__#define __INTLIST_H__ #include <ostream> using namespace std; struct IntNode {int value;IntNode *next;IntNode(int value) : value(value), next(nullptr) {}}; class IntList { private:IntNode *head; public: /* Initializes an empty list.*/IntList() : head(nullptr) {} /* Inserts a data value to the front of the list.*/void push_front(int val) {if (!head) {head = new IntNode(val);} else {IntNode…arrow_forwardWrite a java method to search for an element in an array using a linear search. Many list processing tasks, including searching, can be done recursively. The base case typically involves doing something with a limited number of elements in the list (say the first element), then the recursive step involves doing the task on the rest of the list. Think about how linear search can be viewed recursively; if you are looking for an item in a list starting at index i:o ¬If i exceeds the last index in the list, the item is not found (return -1).o ¬If the item is at list[i], return i.o ¬If the is not at list[i], do a linear search starting at index i+1arrow_forward
- Need help writing a static method in Python that does the following: Given any two lists A and B, determine if:List A is equal to list B; orList A contains list B (A is a superlist of B); orList A is contained by list B (A is a sublist of B); orNone of the above is true, thus lists A and B are unequalSpecifically, list A is equal to list B if both lists have the same values in the sameorder. List A is a superlist of B if A contains a sub-sequence of values equal to B.List A is a sublist of B if B contains a sub-sequence of values equal to A.arrow_forwardIn Java: Modify the attached program code below According to the question a, b and c a. Replace the appendNode() method by an insertNode() method which inserts the new node in such a way to keep the list always sorted in increasing order. b. Add a recursive method displayReverse() which displays the list in reverse order. c. Do the needed changes to the main()in order to reflect the above two changes. public class DoublyLinkedList { private Node head;private Node tail;private int size; DoublyLinkedList() {tail = head = null;size = 0;} public void addNode(String item) {//adding a node at the endNode newNode = new Node(item);if(head == null) {head = tail = newNode;}else {newNode.prev = tail;tail.next = newNode;tail = newNode;}size++;} public boolean remove(String item) {Node current = head;boolean found = false;while((current != null)&&(!found)) {if(current.element.equals(item))found = true;elsecurrent = current.next;}if(found){if(current == head) head =…arrow_forwardWrite a recursive method that gets three parameters as input: an array of integers called nums, an integer called index,and an integer called The purpose of this method is to return true if value is stored in the array starting at nums[index]. That is, you have to check if value is equal to nums[index] or nums[index +1] or nums[index +2 ] …. nums[nums.length -1]. Do not use loops.(java code)arrow_forward
- Implement this algorithm: Implement a function cross_out_multiples that takes as arguments a list of boolean values (true/false) called is_prime and a number n. The function sets the boolean values at all multiples of n (2*n, 3*n, 4*n ...) that are in the list to false. Implement a function sieve(n) which gives back a list of all primes below n.arrow_forwardWrite a recursive method that gets two parameters as input: an array of integers called nums and an integer called index. The purpose of this method is to return the following: nums[index] * nums[index+ 1] * nums[index+ 2] * .. nums[nums.length-1]. Do not use loops. (java code)arrow_forward(a) Write a method public static void insert(int[] a, int n, int x) that inserts x in order among the first n elements of a, assuming these elements are arranged in ascending order. Do NOT use arraylists. x is the last element in a. n does not include x. (b) Using the insert method from Part (a), write a recursive implementation of Insertion Sort.arrow_forward
- Complete the function getLetterFrequency() to return a list containing the number of times each letter of the alphabet occurs in the string passed to it. The Oth index corresponds to the letter "a" or "A", the 1st corresponds to the letter "b" or "B", ..., the 25th corresponds to "z" or "Z". You should ignore any non-alphabet characters that occur in the string. But make sure that both lowercase and uppercase letters contribute to the same count. You must use the ord() function to convert letters into indexed based values. Note that you can offset a number by ord("a") or ord("A") to get the index that you need. You should use at least some of the (or all of) following string methods: • isalpha() lower() • upper() • isupper() • islower() and the following string functions: ord() len()arrow_forwardIt is known that a matrix can be understood (in python) by a list of lists. In this context, make a program that receives 9 numbers, organize them in a matrix 3 x 3 and print this matrix and its transpose. In Phyton3arrow_forwardUse the programming language python to solve the following task You and your neighbor both have equally sized gardens. The gardens are represented as 2D lists, where the garden [i] [j], gives an element to the position (i, j). Each element of a garden is represented as a string, and can be any of the following: "grass", "moss", "strawberry", "rock", "raspberry". You have recently seen that there has been a lot of moss and stone in your garden, and suspect that it is your neighbor who has put it there. To fix this, complete the clean_garden (my_garden, neighbors_garden) function. Here you will first create small functions to make it easier to solve the actual task. Create a function find_item that takes in two arguments: (garden, item), which returns an (i, j) position as a tuple, whose item is in the garden. If it does not exist, return None Create a function swap_items that exchanges two elements between two gardens, let it take in these arguments: (garden1, garden2, pos1, pos2),…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
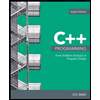