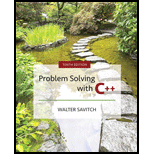
This Practice
File user.cpp:
namespace Authenticate
{
void inputUserName()
{
do {
cout << “Enter your username (8 letters only)” <<
endl;
cin >> username;
} while (!isValid());
}
string getUserName()
{
return username;
}
}
Define the username variable and the isValid() function in the unnamed namespace so the code will compile. The isValid() function should return true if username contains exactly eight letters. Generate an appropriate header file for this code.
Repeat the same steps for the file password.cpp, placing the password variable and the isValid() function in the unnamed namespace. In this case, the isValid() function should return true if the input password has at least eight characters including at least one nonletter:
File password.cpp:
namespace Authenticate
{
void inputPassword()
{
do {
cout << “Enter your password (at least 8 characters “
<<
“and at least one nonletter)” << endl;
cin >> password;
} while (!isValid());
}
string getPassword()
{
return password;
}
}
At this point you should have two functions named isValid(), each in different unnamed namespaces. Place the following main function in an appropriate place. The program should compile and run.
int main()
{
inputUserName();
inputPassword();
cout << “Your username is ” << getUserName() <<
“ and your password is: ” <<
getPassword() << endl;
return 0;
}
Test the program with several invalid usernames and passwords.

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Starting out with Visual C# (4th Edition)
Concepts of Programming Languages (11th Edition)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
Concepts Of Programming Languages
Modern Database Management (12th Edition)
- What would be the printout of the following code if the same file.txt was used as above? int main() { fstream file("file.txt", ios::in); char ch; file.get(ch); while (!file.eof()) { cout << ch << " "; file.get(ch); } file.close(); return 0;} Group of answer choices I have a dream. I h a v e a d r e a m . Ihaveadream. I have a dream.I have a dream.arrow_forwardWrite a program to read two file names from the command line. If the number of arguments in the command line are more than or less than two, then the program should terminate with an appropriate error message. Otherwise, it should open the first file and copy its contents into the second file, in effect overwriting its contents, if any.arrow_forwardWrite a Python program to combine each line from the first file with the corresponding line in the second file and then save it in a 3rd file. Consider, both the files have same number of lines. Assume, each line has a newline(\n) at the end. ========================================================= Example:Input from file 1:HelloHiNice Input from file 2:BadWorseWorst Output in 3rd FIle:Hello BadHi WorseNice Worst ========================================================= Hint(1):Use the write() function what will be the answer of this in python programming languagearrow_forward
- Solve in Python Include Screenshots of Input and Output For this lab, you will write a program that creates and edits a test file called users.txtYour program should implement the following functions: add_user – Accepts a username as an argument and adds it to the file update_user – Accepts an old username and a new username as separatearguments and replaces any instance of that the old username with the newusername in the file. remove_user – Accepts a username as an argument and removes the userfrom the fileUse the following code to test your program:add_user(“bob”)add_user(“joe”)add_user(“ann”)add_user(“mike”)update_user(“joe”, “jo”)remove_user(“ann”)Your final file should look like:bobjomikeYour submission should include your source code (.py file) and either yourfinal text file or a screenshot of it.arrow_forwardThe iostream library provides mechanisms for error handling, such as the failbit, badbit, and eofbit. However, if these are not checked after an input/output operation, it can lead to incorrect program behavior. For example, if you attempt to read past the end of a file without checking eofbit, the program may enter an infinite loop. *Can you provide a simple program of the above paragraph? How do you correct this vunerability?*arrow_forward(Practice) a. Write a set of two statements declaring the following objects as ifstream objects, and then opening them as text input files: inData.txt, prices.txt, coupons.dat, and exper.dat. b. Rewrite the two statements for Exercise 2a, using a single statement.arrow_forward
- Please help me modify my code according to the error prompt #include <stdio.h> void processData(const char* inputFilePath, const char* outputFilePath) { FILE* inputFile = fopen(inputFilePath, "r"); FILE* outputFile = fopen(outputFilePath, "w"); int n; fscanf(inputFile, "%d", &n); // Read the number of data points fprintf(outputFile, "%d\n", n); // Write the number of data points to output int value; for (int i = 0; i < n; i++) { fscanf(inputFile, "%d", &value); // Read the data point int squaredValue = value * value; // Compute the square of the data point fprintf(outputFile, "%d\n", squaredValue); // Write the squared value to output } fclose(inputFile); fclose(outputFile);} int main() { processData("input_file.dat", "output_file.dat"); return 0;}arrow_forwardCreate a program that reads three lines from a text file. Each line should be read one at a time. For each word on a line, the user should be asked to specify how many syllables are in that word. The program should then inform the user if the file contains a valid Hiku using the function below: def isHiku (firstLine, secondLine, thirdLine):if (firstLine == 5 and secondLine == 7 and thirdLine == 5): return True return False print("The file contains a Hiku:", isHiku(5,7,5))Note: You must replace the values in the function with the numbers counted by your program.arrow_forwardThe following code is for the code file namedpipe_p1.c. // This process writes first, then reads #include <stdio.h> #include <string.h> #include <fcntl.h> #include <sys/stat.h> #include <sys/types.h> #include <unistd.h> int main() { int fd; // FIFO file path char * myfifo = "/tmp/myfifo"; // Creating the named file(FIFO) // mkfifo(<pathname>, <permission>) mkfifo(myfifo, 0666); char arr1[80], arr2[80]; // Open FIFO for write only fd = open(myfifo, O_WRONLY); // Take an input arr2ing from user. // 80 is maximum length printf("your message: "); fgets(arr2, 80, stdin); // Write the input arr2ing on FIFO // and close it write(fd, arr2, strlen(arr2)+1); close(fd); // Open FIFO for Read only fd = open(myfifo, O_RDONLY); // Read from FIFO read(fd, arr1, sizeof(arr1)); // Print the read message printf("Received: %s\n", arr1); close(fd);…arrow_forward
- Write a CPP program to do the following: Read the attached text file "DISTRICT-COSC-1437.txt". Uppercase each line and write the uppercase results to a file named "UpperCased1437.txt". This is the target file. If the target file exists when the program starts, prompt to overwrite the file. If declined, then do not process. Deliverable is a working CPP program. Textfile DISTRICT-COSC-1437.txt COSC-1437 Tarrant County College Page 1 of 2 COSC-1437 Programming Fundamentals II TARRANT COUNTY COLLEGE DISTRICT MASTER SYLLABUS COURSE DESCRIPTION This course focuses on the object-oriented programming paradigm, emphasizing the definition and use of classes along with fundamentals of object-oriented design. The course includes basic analysis of algorithms, searching and sorting techniques, and an introduction to software engineering processes. Students will apply techniques for testing and debugging software. This course is included in the Field of Study Curriculum for Computer Science.…arrow_forwardIn C write a program that takes a file name and two strings as command line arguments, and writes the longer of the two strings to the file with the given name. If the given strings are the same length, write the first string given to the file. If the user does not provide the correct number of command line arguments, print an error message and exit the program with a non-zero exit code.arrow_forwardThrough this programming assignment, the students will learn to do the following: Practice processing command line arguments. Perform basic file I/O. Use structs, pointers, and strings. Use dynamic memory. This assignment asks you to sort the letters in an input file and print the sorted letters to an output file (or standard output) which will be the solution. Your program, called codesolve, will take the following command line arguments: % codesolve [-o output_file_name] input_file_name Read the letters in from the input file and convert them to upper case if they are not already in uppercase. If the output_file_name is given with the -o option, the program will output the sorted letters to the given output file; otherwise, the output shall be to standard output. In addition to parsing and processing the command line arguments, your program needs to do the following: You need to construct a doubly linked list as you read from input. Each node in the list will link to the one in…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
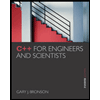
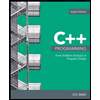