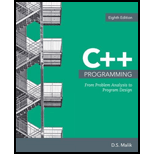
Explanation of Solution
The program execution is explained in the in-lined comments:
#include <iostream>
using namespace std;
int main()
{
//declare variables dec1, dec2 and initialize
double dec1 = 2.5;
double dec2 = 3.8;
//declare double pointers p and q
double *p, *q;
//assign the address of variable dec1 to p
p = &dec1;
//assign the value of dec2 - dec1 to the memory
//location pointed to by p which is dec1
//so dec1 now holds the value (3.8-2.5 =) 1.3
*p = dec2 - dec1;
//q is assigned the value of p so both the pointers
//now point to variable dec1
q = p;
//content of the memory location pointed to by q
//is assigned 10.00 so dec1 holds the value 10.0
*q = 10.0;
//the contents of the memory location pointed to by p
//i.e dec1 is assigned the value of the RHS expression
//RHS = 2 &*#x00A0;10 + 10...

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
C++ Programming: From Problem Analysis to Program Design
- i). Write a C++ program to prompt the user to input her/his age and print it on the screen, asshown below.Your age is 20 years old. ii). Write a C++ program that asks the user for a number n and gives him the possibility to choosebetween computing the sum and computing the product of 1,...,n iii). Write a program that asks the user for a number n and gives him the possibility to choosebetween computing the sum and computing the product of 1,...,n. Expected output: 0,30,25,28arrow_forwarda) What is the risk of using strcpy() in C given the code below? void f1(char *str2) { int y; char str1[10]; strcpy(str1, str2);arrow_forwardWrite a C program that reads a double value (x) from the user and then uses it to compute the value of the mathematical constant e to the power of x (ex) and print it in main using the following infinite series: ex = 1 + x/1! + x2/2! + x3/3! + x4/4! + … Your program should include the following three functions: 1- function called power which takes two parameters: x (double) and y (integer) and returns the value of xy . Function power MUST use a while loop. ( Not allowed to use the predefined function pow in math.h). 2- function called factorial which takes one parameter x (integer) and returns its factorial. Function factorial MUST use a for loop. 3- function called compute_ex which receives one parameter x (double) and returns the value of ex for the given x using the series above as well as functions power and factorial ( 1 and 2 above) . Function computer_ex MUST use a do/while loop. The loop should stop when the new term added ( term = Xn/n! where n=0,1,2,3,…) to the series is less…arrow_forward
- Write a “C” program to find the summation of the following series: 1/8+ 1/27 + 1/64 + 1/125 + 1/216 + .............. + 1/(n+1)3Hint: To compute the power of a number, you can apply basic mathematics multiplications equations or use the pow() function computes the power of a number.The basic mathematics multiplications equations:For example: [In Mathematics] x4 = x*x*x*x [In programming]The pow() function:The pow() function takes two arguments (base value and power value) and, returns the power raised to the base number.For example, [In Mathematics] xy = pow(x, y) [In programming]The pow() function is defined in <math.h> header file (#include<math.h>)arrow_forwardWrite a Haskell program numBulls that takes as input two positive integers as input and returns -1 if either the two integers have different number of digits or either of them have repeating digits. However, if the two integers have the same number of digits and also do not have any repeating digits, the function returns the number of digits that match in the exact same positions in the integers.arrow_forwardWhat is the output of the following C++ code? #include<iostream> using namespace std; class X { int m; public: X() : m(10) { } X(int mm): m(mm) { } int getm() { return m; } }; class Y : public X { int n; public: Y(int nn) : n(nn) {} int getn() { return n; } }; int main() { Y yobj( 100 ); cout << yobj.getm() << " " << yobj.getn() << endl; }arrow_forward
- What is the output of the following C++code? int x = 15; int y = 3; if (x + y > {y = x -x = y +cout << x << " " << y << " " << x + y << " " << y - x << endl;}else{x = y - x + y %5;cout << x << " " << y << " " << x - y << " " << x + y << endl; }arrow_forwardwhat is the output of the following c++ statement: int a, b, c, d; a = 5; b = 4; c = (++b + a--); cout << a << " " << b << " " << c << endl;arrow_forwardQ.4. Write a C++ program which inputs two numbers and an operator (+, -, *, /) from the user,and based on the user selected operator, performs the required function.For output display, enter the first number by taking the last two digits of your registrationnumber, and second number as the reverse of the first number.A sample output is of the following form;Sample Output 1Enter the first number : 17Enter the second number : 20Enter the operator (+,-,*,/) : *Result : 340Sample Output 2Enter the first number : 15Enter the second number : 6 Page 2 of 2 Enter the operator (+,-,*,/) : /Result : 2arrow_forward
- I). Write a program in c++ with class that will help an elementary school student learn multiplication. Use the rand function to produce two positive one-digit integers. The program should then prompt the user with a question, such as How much is 6 times 7? The student then inputs the answer. Next, the program checks the student’s answer. If it’s correct, display the message "Very good!" and ask another multiplication question. If the answer is wrong, display the message "No. Please try again." and let the student try the same question repeatedly until the student finally gets it right. A separate function should be used to generate each new question. This function should be called once when the application begins execution and each time the user answers the question correctly.arrow_forwardConsider the following C++ function. int mystery(int num1, int num2){if (num1 > 0){for (int i = 1; i <= num1; i++)num2 = num2 * i;return num2;}else if (num2 > 0){for (int i = 0; i <= num2; i++)num1 = num1 + i;return num1;}return 0;}What is the output of the following statements?a. cout << mystery(4, -5) << endl;b. cout << mystery(-8, 9) << endl;c. cout << mystery(2, 3) << endl;d. cout << mystery(-2, -4) << endl;arrow_forwardin c++ how can we limit the amaount of number we can put on the output? for example, we allready had the program and we run it, and we run it. the ouput need us to fill down handphone number. but when i wrote my number its error because my number have 12 number. how i can fix this?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
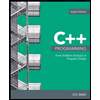