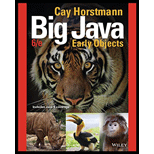
Evaluation of Expression
Program plan:
Filename: “Constant.java”
This program file is used to define a class “Constant”. In the code,
- Define a class “Constant”.
- Declare an integer “number”.
- Define the constructor “Constant()”.
- Set the value of “number”.
- Define the method “value()”.
- Return the value of “number”.
- Define the method “toString()”.
- Return the value of “number” as a string.
- Define the method “derivative()”.
- Return the value.
Filename: “Variable.java”
This program file is used to define a class “Variable”. In the code,
- Define a class “Variable”.
- Define a variable “letter”.
- Define the constructor “Variable ()”.
- If the “letter” is not equal to “x”,
- Throw an exception.
- Set the letter.
- If the “letter” is not equal to “x”,
- Define the method “value()”.
- Return the value of “x”.
- Define the method “toString()”.
- Return the value of “letter”.
- Define the method “derivative()”.
- Return the value.
Filename: “Expression.java”
This program file is used to define an interface “Expression”. In the code,
- Define an interface “Expression”.
- Declare the method “value()” with argument “x”.
- Declare a method “toString()”.
- Declare a method “derivative()”.
Filename: “Difference.java”
This program file is used to define a class “Difference”. In the code,
- Define a class “Difference”.
- Define the class members “leftOp” and “rightOp”.
- Define the constructor “Difference ()”.
- Set the value of class members.
- Define the method “value()” to calculate the difference of two expression.
- Return the difference.
- Define the method “toString()”.
- Return the expression in string format.
- Define the method “derivative()”.
- Return the derivative.
Filename: “Product.java”
This program file is used to define a class “Product”. In the code,
- Define a class “Product”.
- Define the class members “leftOp” and “rightOp”.
- Define the constructor “Product()”.
- Set the value of class members.
- Define the method “value()” to calculate the product of two expression.
- Return the product.
- Define the method “toString()”.
- Return the expression in string format.
- Define the method “derivative()”.
- Return the derivative.
Filename: “Quotient.java”
This program file is used to define a class “Quotient”. In the code,
- Define a class “Quotient”.
- Define the class members “leftOp” and “rightOp”.
- Define the constructor “Quotient()”.
- Set the value of class members.
- Define the method “value()” to calculate the quotient of two expression.
- Return the quotient.
- Define the method “toString()”.
- Return the expression in string format.
- Define the method “derivative()”.
- Return the derivative.
Filename: “Sum.java”
This program file is used to define a class “Sum”. In the code,
- Define a class “Sum”.
- Define the class members “leftOp” and “rightOp”.
- Define the constructor “Sum()”.
- Set the value of class members.
- Define the method “value()” to calculate the sum of two expression.
- Return the sum.
- Define the method “toString()”.
- Return the expression in string format.
- Define the method “derivative()”.
- Return the derivative.
Filename: “ExpressionTokenizer.java”
This program file is used to define a class “ExpressionTokenizer”. In the code,
- Define a class “ExpressionTokenizer”.
- Define the class members “input”, “start” and “end”.
- Define the constructor “ExpressionTokenizer()”.
- Set the values of “input”, “start”, “end” and find the first token using “nextToken()”.
- Define the method “peekToken()”.
- If the “start” is greater than “input.length()”.
- Return “null”.
- Else,
-
- Return the substring.
- Return “null”.
- If the “start” is greater than “input.length()”.
- Define the method “nextToken()”.
- Call the method “peekToken()” to get the token.
- Assign the value of “end” to “start”.
- If the value of “start” is greater than or equal to length of the input,
- Return the value of “r”.
- If the character at “start” is a digit,
-
- Set “end” equal to “start+1”,
- Iterate a “while” loop,
- Increment the “end” by 1.
- Set the value of “end”.
- Increment the “end” by 1.
- Return the value of “r”.
- Return the value of “r”.
Filename: “Evaluator.java”
This program file is used to define a class “Evaluator”. In the code,
- Define a class “Evaluator”.
- Define the class members “tokenizer” which is an object of class “ExpressionTokenizer”.
- Define the constructor “Evaluator()”.
- Define “tokenizer”.
- Define the method “getExpressionValue()”.
- Get terms using “getTermValue()” to “value”.
- Define a Boolean value “done” and assign “false” to it.
- While “true”,
- Call “peekToken()” to get the token and assign to “next”.
- If the value of “next” is “+” or “-”.
- Get the next token using “nextToken()”.
- Get next term to “value2”.
- If the “next” is “+”,
-
- Calculate the sum of “value” and “value2” and assign to “value”.
- Else,
-
- Calculate the difference of “value” and “value2” and assign to “value”.
- Else,
- Set “done” equal to “true”.
-
-
- Return “value”.
-
- Define the method “getTermValue()”.
- Get factors using “getFactorValue()” to “value”.
- Define a Boolean value “done” and assign “false” to it.
- While “true”,
- Call “peekToken()” to get the token and assign to “next”.
- If the value of “next” is “*” or “/”.
- Get the next token using “nextToken()”.
- Get next factor to “value2”.
- If the “next” is “*”,
-
- Calculate the Product of “value” and “value2” and assign to “value”.
- Else,
-
- Calculate the Quotient of “value” and “value2” and assign to “value”.
- Else,
- Set “done” equal to “true”.
-
-
- Return “value”.
-
- Define the method “getFactorValue()”.
- Declare “value”.
- Call “peekToken()” to get the token and assign to “next”.
- If the value of “next” is “(”.
- Get the next token using “nextToken()”.
- Discard “(” using “nextToken()”.
- Get next expression to “value”.
- Discard “)” using “nextToken()”.
-
-
- Else,
- If the “next” is “x”,
- Get the variable “x” to “value”.
- Else,
- Get the next token to “value”.
- If the “next” is “x”,
- Return “value”.
- Else,
-
-
Filename: “ExpressionCalculator.java”
This program file is used to define a class “ExpressionCalculator”. In the code,
- Define a class “ExpressionCalculator”.
- Define the method “main()”.
- Define the object “in” of “Scanner”.
- Prompt the user to enter the expression.
- Read the lines using “nextLine()”.
- Evaluate the expression “input”.
- Call the method “getExprssionValue()” and save to “expr”.
- Print “expr”.
- Prompt the user to enter the value for “x”.
- Scan for the value of “x”.
- Call the method “value()” on “x” and save the result to “value”.
- Print “value”.
- Call the method “derivative()” and save the result to “df”.
- Print “df”.
- Define the method “main()”.

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
Big Java, Binder Ready Version: Early Objects
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
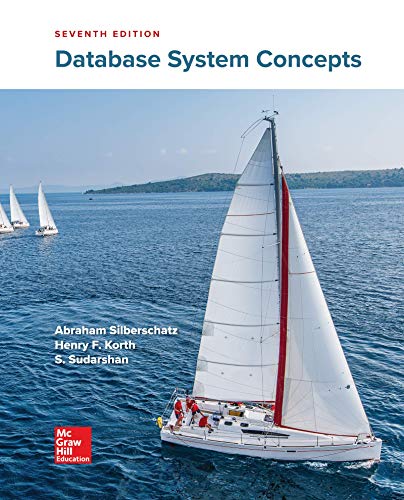
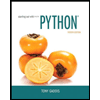
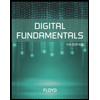
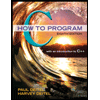
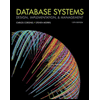
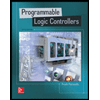