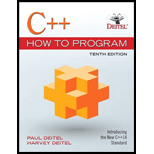
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 13.14E
Program Plan Intro
Program Plan:
- Insert required header Files
- Create new file PhoneNumber.h.
- Make a class PhoneNumber and declare extraction and insertion operators as friend to class.
- Declare three string variable “ areacode ”, “ exchange ”, “ line ” and one character array “ phno ” to get phone number in an array.
- End header class.
- Close and save header file.
- Create new file Phonenumber.cpp. This will contain definitions and modification required in extraction operator ">>".
- Include header files, must include PhoneNumber.h
- Overload the insertion operator to display areacode, exchange and line.
- Make changes the extraction operator definition.
- use "getline()" function to get input into "phno". The "getline()" function is used to identify psaces also.
- Use if construct to check various conditions
- Check for length of input entered which should be 14, use input.clear(ios::failbit) to set failbit.
- Check that areacode does not start with either 0 or 1, if it does then use input.clear(ios::failbit) to set failbit.
- Check that exchange does not start with either 0 or 1, if it does then use input.clear(ios::failbit) to set failbit.
- Check that middle digit of areacode should be either 0 or 1, if not then use input.clear(ios::failbit) to set failbit.
- If all the conditions are false, this means that input is correct, thus put them into areacode, exchange and line of number object passed through the function.
- return input
- end function
- Make new C++ file for main() function.
- Include header files, must include PhoneNumber.h.
- Start main function.
- Declare an object of PhoneNumber class phone.
- Display message to enter phone number.
- Use cin>>phone which will call overloaded extraction operator.
- Check whether state returned is goodbit(incase failbit is not set in the function) then display phone number else display error.
- Return and exit.
Program Description:
Program to get correct input by overloading extraction operator.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
- in this exercise, please do not include and use string class. The function is using only array notation and manipulation.- string functions such as strlen is not allowed.- it should not have multiple return statements in the same function- there should be no global variable.- the function should not traverse the arrays more than once (e.g. looping through the array once only)
A C++ PROGRAM named "isTheLastNumberTheMax" that accepts an array of integers and its size. It will return true if the last number in the array is the maximum number in that array and false otherwise. In addition, it also returns another boolean to indicate whether this list contains more than one maximum value.For example, if this is called with the array of {10, 20, 30, 40, 50}, it will return true and falseIf this is called with the array of {50, 10, 20, 30, 40, 50}, it will return true and true.
A C++ PROGRAM named "changeCase" that takes an array of characters terminating by NULL character (C-string) and…
- in this exercise, please do not include and use string class. The function is using only array notation and manipulation.- string functions such as strlen is not allowed.- it should not have multiple return statements in the same function- there should be no global variable.- the function should not traverse the arrays more than once (e.g. looping through the array once only)
write a c++ program named "isTheLastNumberTheMax" that accepts an array of integers and its size. It will return true if the last number in the array is the maximum number in that array and false otherwise. In addition, it also returns another boolean to indicate whether this list contains more than one maximum value.For example, if this is called with the array of {10, 20, 30, 40, 50}, it will return true and falseIf this is called with the array of {50, 10, 20, 30, 40, 50}, it will return true and true.
I need the answer quickly
What is the meaning of the following declaration?
int(*p[5])();
a) p is pointer to functionb) p is array of pointer to functionc) p is pointer to such function which return type is the arrayd) p is pointer to array of function
Chapter 13 Solutions
C++ How to Program (10th Edition)
Ch. 13 - (Write C ++ statements) Write a statement for each...Ch. 13 - (Inputting Decimal, Octal and Hexadecimal Values)...Ch. 13 - Prob. 13.8ECh. 13 - (Printing with field Widths) Write a program to...Ch. 13 - (Rounding) Write a program that prints the value...Ch. 13 - (Length of a String) Write a program that inputs a...Ch. 13 - (Converting Fahrenheit to Celsius) Write a program...Ch. 13 - In some programming language, string are entered...Ch. 13 - Prob. 13.14ECh. 13 - Prob. 13.15E
Knowledge Booster
Similar questions
- dissolve in c ++. Below, write the code of the program that calls the desired functions in the main function and performs the desired operations. a) random (random number range should be set to be in the range of 5-15) 10 an integer with an element produces numbers and transfers them to an array called array A and displays them enter a function that prints. b) then this sequence takes the arithmetic mean of the elements of the sequence A, round the found average value to the nearest large integer and return it to variable A. enter a function that assigns and prints the variable A on the screen. c) Then write a function that finds the factorial of the variable A value and assigns it to factorial A and prints it on the screenarrow_forwardFix an error and show it please? And here are the information about the homework and for the error too. def kwargs_to_args_decorator(*args, **kwargs): This question is meant to test your knowledge of creating a decorator that accepts an arbitrary number of positional and keyword arguments, to decorate a function that accepts an arbitrary number of positional and keyword arguments, and alters the arguments before passing them to the decorated function. When the decorated function is invoked, this decorator should modify the arguments the decorated function receives. This decorator should filter out all positional arguments passed to the decorated function, which are found in the positional arguments passed to the decorator when the decorator was initialized. It should also filter out all keyword arguments with keys that are found in the keyword arguments given to the decorator when the decorator was initialized. After performing the modifications to the arguments, the decorator should…arrow_forward8. using c++, Write a function method that determines the mean of all the values in an array of integers. Your mean function should call a separate function that you write that determines the sum. Don’t use built-in sum or mean gadgets, but roll your own.arrow_forward
- Generate the array inside main and print it before and after the function call inside the main function itself. C++arrow_forwardWhat is wrong in the following function?arrow_forward- In C++ using Visual Studio - Seperate the files if there is any .cpp, .main or .h files. PART 1Read the contents of the text file and store them in a C++ data structure called std::map<string, int>This map is a collection of sorted <key, value> pairsIn this case, the keys are unique words found in the text file, and the values represent the number of times each word appearsFor example, since the word "cat" appears twice in the file, then map["cat"] = 2 PART 2Iterate through the map and print each key in the order they appearThis will give you a sorted list of unique wordsHere's the expected result: "a big cat does everything feeding goats helping injured juvenile kangaroos locating missing notorious objects playing quietly reading superb tales upvoting videos with xylophone yielding zebras" PART 3Iterate through the map again, this time printing each associated valueThe values represent how many times each word was found in the text filePay attention to the sequence of…arrow_forward
- Finish the swap function below using pointers and write the call to swap in the reverse function using the address of the array positions array[i] and array[size-i-1].using pointers. DON'T FORGET TO DECLARE THE PARAMETERS in the function. (Hint: if you can't figure it out with pointers, do it with the references #include <iostream>using namespace std; // Function to swap two ints using pointers// @param pointer to an int// @param pointer to an intvoid swap( ) {// TODO: Add code that swaps two integers using pointers } // Function to reverse arrayvoid reverse(int a[], int size) {for (int i = 0; i < size/2; i++) {// TODO: write the call to swap in the reverse function using the // address of the array positions array[i] and array[size-i-1].arrow_forwardWhat is the output of following code.arrow_forwardWhat is the output of following code segment?arrow_forward
- c++, anyone able to help with this, no vectors, no sstream, no pointers, also need a toLower function loop within to account for case insensivity, NEED ASAP pleasearrow_forwardIs that right?For functions that are flexibly bound, you need pointers or references.arrow_forwardWhat is the result of multiplying ptr by four? Assuming ptr is an int reference, the following occurs:arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
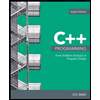
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
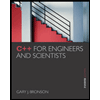
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr