(Triangle class) Design a new Triangle class that extends the abstract GeometricObject class. Draw the UML diagram for the classes Triangle and GeometricObject then implement the Triangle class. Write a test

Program to create Triangle class
Program Plan:
- Include a class name named “Exercise_13_01”.
- Include the scanner package.
- Declare the main() function.
- Create a scanner object.
- Prompt the user to enter three sides of the triangle.
- Prompt the user to enter a color.
- Prompt the user to enter a Boolean value on whether the triangle is filled.
- Create triangle object with the entered user input.
- Set color and set filled in the triangle.
- Print the output.
- Close the main() function.
- Close the class “Exercise_13_01”.
- Include an abstract class “GeometricObject”.
- Declare all the data types of the variables.
- Construct a default geometric object.
- Construct a geometric object with color and filled value.
- Assign color and fill to the object.
- Set a new color.
- Define an isFilled method returns filled and since filled is Boolean; the get method is named isFilled.
- Set a new filled and getDateCreated method.
- Override toString function to return output.
- Define Abstract method getArea.
- Define Abstract method getPerimeter.
- Close class.
- Include a class “Triangle” that extends the class GeometricObject.
- Declare the three sides of the triangle.
- Include a triangle constructor.
- Assign values to all three sides of the triangle.
- Assign triangle values to all attributes.
- Return side1 and Set side1 to a new length.
- Return side2 and Set side2 to a new length.
- Return side3 and Set side3 to a new length.
- Create a Function to return area of the Triangle.
- Create a function to return a string description of the object.
- Close class.
The java code to design a new Triangle class that extends the abstract GeometricObject class and to design a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled or not.
Explanation of Solution
Program:
File name: Exercise_13_01.java
//package to have user input
import java.util.Scanner;
//class Definition
public class Exercise_13_01
{
// Main method
public static void main(String[] args)
{
// Create a Scanner object
Scanner input = new Scanner(System.in);
/* Prompt the user to enter three sides of the triangle */
System.out.print("Enter three side of the triangle: ");
double side1 = input.nextDouble();
double side2 = input.nextDouble();
double side3 = input.nextDouble();
// Prompt the user to enter a color
System.out.print("Enter a color: ");
String color = input.next();
/*Prompt the user to enter a boolean value on whether the triangle is filled*/
System.out.print("Is the triangle filled (true / false)? ");
boolean filled = input.nextBoolean();
/* Create triangle object with the entered user input */
Triangle triangle = new Triangle(side1, side2, side3);
// set color
triangle.setColor(color);
// set filled
triangle.setFilled(filled);
// print the output
System.out.println(triangle.toString());
System.out.println("Area: " + triangle.getArea());
System.out.println("Perimeter: " + triangle.getPerimeter());
System.out.println("Color: " + triangle.getColor());
System.out.println("Triangle is" + (triangle.isFilled() ? "" : " not ")
+ "filled");
}
}
Filename: GeometricObject.java
//abstract class GeometricObject definition
public abstract class GeometricObject
{
//data type declaration
private String color = "while";
private boolean filled;
private java.util.Date dateCreated;
// Construct a default geometric object
protected GeometricObject()
{
dateCreated = new java.util.Date();
}
/* Construct a geometric object with color and filled value */
protected GeometricObject(String color, boolean filled)
{
dateCreated = new java.util.Date();
//assign color and fill
this.color = color;
this.filled = filled;
}
// Return color
public String getColor()
{
return color;
}
// Set a new color
public void setColor(String color)
{
this.color = color;
}
/* isFilled method returns filled and Since filled is boolean,the get method is named isFilled */
public boolean isFilled()
{
return filled;
}
// Set a new filled
public void setFilled(boolean filled)
{
this.filled = filled;
}
// Get dateCreated
public java.util.Date getDateCreated()
{
return dateCreated;
}
//override toString function to return output
@Override
public String toString()
{
return "created on " + dateCreated + "\ncolor: " + color +
" and filled: " + filled;
}
// define Abstract method getArea
public abstract double getArea();
//Define Abstract method getPerimeter
public abstract double getPerimeter();
}
Filename: Triangle.Java
/*Class definition of Triangle that extends the class GeometricObject */
public class Triangle extends GeometricObject
{
//declare the three sides of the triangle
private double side1;
private double side2;
private double side3;
//triangle constructor
public Triangle()
{
}
//assign values to all three sides of the triangle
public Triangle(double side1, double side2, double side3)
{
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
//assign triangle values to all attributes
public Triangle(double side1, double side2, double side3, String color, boolean filled)
{
this(side1, side2, side3);
setColor(color);
setFilled(filled);
}
// Return side1
public double getSide1()
{
return side1;
}
// Set side1 to a new length
public void setSide1(double side1)
{
this.side1 = side1;
}
// Return side2
public double getSide2()
{
return side2;
}
// Set side2 to a new length
public void setSide2(double side2)
{
this.side2 = side2;
}
// Return side3
public double getSide3()
{
return side3;
}
// Set side3 to a new length
public void setSide3(double side3)
{
this.side3 = side3;
}
// Function to Return area of the Triangle
@Override
public double getArea()
{
double s = (side1 + side2 + side3) / 2;
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
/* override function to Return perimeter of the triangle */
@Override
public double getPerimeter()
{
return side1 + side2 + side3;
}
/*override function to Return a string description of the object*/
@Override
public String toString()
{
return super.toString() + "\nArea: " + getArea() + "\nPerimeter: " + getPerimeter();
}
}
UML Diagram:
Explanation:
- Here, GeometricObject is an abstract class that extends the triangle class which is represented using an arrow. The triangle class then contains a rectangle that represents the objects used to input the three sides of the triangle.
- The remaining part is used to represent all the methods and functions used in the triangle class.
- The Triangle() method creates a triangle with default sides. The method Triangle(side1:double, side2; double,side3;double) creates a triangle of the specified sides.
- Then the respective sides of the triangles are returned using getSide() methods.
- Then the method getArea() returns the area of the triangle and the method getPerimeter() returns the perimeter of the triangle and the function toString() returns a string description of the object.
Enter three side of the triangle: 3
4
5
Enter a color: red
Is the triangle filled (true / false)? false
created on Sun Jul 08 13:24:03 IST 2018
color: red and filled: false
Area: 6.0
Perimeter: 12.0
Area: 6.0
Perimeter: 12.0
Color: red
Triangle is not filled
Want to see more full solutions like this?
Chapter 13 Solutions
INTRO TO JAVA PROGRAMMING: COMPREHENSIV
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Starting Out with C++: Early Objects
C How to Program (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Computer Systems: A Programmer's Perspective (3rd Edition)
- Reuse your Car class . In a main, build an object of that class, and print out the object using System.out.println(). Notice that this simply reports the memory address of the object in question, and we’d like to do something more useful. To replace (or override) the toString (or equals) function. Now, build a toString function that prints out the make, model, and odometer reading for a vehicle object. public class Car { //instance variables private int odometer; private String make; private String model; //overloading //constructors public Car(int odometer,String make, String model) { this.odometer = odometer; this.make = make; this.model = model; } public Car(String make, String model) { this.make = make; this.model = model; } public Car(String make) { this.make = make; } /* *getter & setter methods */ public int getOdometer() { return odometer; } public void…arrow_forwardsame function which prints "Rectangle is a polygon" and "Triangle is a polygon" respectively. Again, make another class named Square having the same function which prints "Square is a rectangle". Now, try calling the function by the object of each of these classes.arrow_forwardWhat are the three things you need to do when working with classes that include pointer variables as member variables?arrow_forward
- can someone help me with this problem in C++ In this exercise, you will work with 3 classes: Shape, Triangle and Rectangle. The first class is the class Shape. The Shape class has two float type properties: center_x and center_y. The Shape class also has the following methods: a constructor Shape(), that will set center_x and center_y to zero. set/get functions for the two attributes 1) You need to implement two additional functions for Shape: setCenter(float x, float y), that will set the new center and print: Figure moved to [<center_x>, <center_y>] draw(), that will print: Drawing Figure at [<center_x>, <center_y>] 2) You will have to implement another class, called Triangle, which inherits from the Shape class. The Triangle class has one int attribute: side. The Triangle class has the following methods: a constructor that will receive one int parameters (side) set/get for its attribute setCenter(float x, float y), that will set the new center and…arrow_forwardCan you implement the Derived Class Parameterized constructor? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first. Implement the constructor Dell(String name) of the Derived Class Dell which takes a string, name. We have already implemented the Base Class Laptop with the member function getName() and a parameterized constructor. Input# Laptop name is being passed through the parameterized constructor. Output# getName() method is returing Laptop name. Sample Input# Dell dell = new Dell("Dell Inspiron"); Sample Output# "Dell Inspiron"arrow_forwardJava- Suppose that Vehicle is a class and Car is a new class that extends Vehicle. Write a description of which kind of assignments are permitted between Car and Vehicle variables.arrow_forward
- Can a class be a super class and a sub-class at the same time? Give example.arrow_forwardUse abstract classes and pure virtual functions to design classes to manipulate various types ofaccounts. For simplicity, assume that the bank offers three types of accounts: savings, checking, andcertificate of deposit, as described next.Savings accounts: Suppose that the bank offers two types of savings accounts: one that has no minimumbalance and a lower interest rate and another that requires a minimum balance and has a higherinterest rate.Checking accounts: Suppose that the bank offers three types of checking accounts: one with a monthlyservice charge, limited check writing, no minimum balance, and no interest; another with no monthlyservice charge, a minimum balance requirement, unlimited check writing and lower interest; and a thirdwith no monthly service charge, a higher minimum requirement, a higher interest rate, and unlimitedcheck writing.Certificate of deposit (CD): In an account of this type, money is left for some time, and these accountsdraw higher interest rates than…arrow_forwardCan we call the constructor of a class more than once for an object?arrow_forward
- Which three actions are obligatory when dealing with classes whose member variables are pointers?arrow_forward#6 To use Parameterized.class with the @RunWith annotation, we need to import _______arrow_forwardHow can you prevent the derived class from accessing methods from the base class?arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
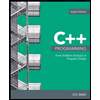