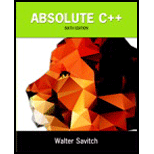
1 . The following variables are used in the program:
- seq variable is of integer type and used to store the user-entered Fibonacci sequence.
- nvariable is of integer type and used as a parameter of the function name findFibonacciNumber.
2. The following functions are used in the program:
- findFibonacciNumber ()function is used to find the Fibonacci number of the user-entered sequence.
- main () function is used to get the user input, call the function, and display the Fibonacci number returned by the function.
Program description:
The main purpose of the program is to create a function named findFibonacciNumber . This function get the sequence of the Fibonacci series as parameter, then calculate the Fibonacci number forsequence, and return the Fibonacci number.

Explanation of Solution
Program:
//including essential header file #include <iostream> //using standard namespace using namespace std; //function to find the Fibonacci number of the given sequence int findFibonacciNumber(int n) { //when the fibonacci sequence is equal to 0 or 1 if (n == 0 || n == 1) { //return 1 return 1; } //call the function recursively to find the fibonacci number return findFibonacciNumber(n-1) + findFibonacciNumber(n-2); } //main function int main() { //variable declaration to store the sequence of Fibonacci number int seq; //prompt the user to enter the fibonacci sequence cout<<"Enter the fibonacci sequence: "; //get the value from the user cin>>seq; //call the function to find and display the fibonacci number of the given sequence cout<<"The fibonacci number of sequence "<<seq<<" is "<<findFibonacciNumber(seq); }
Explanation:
In the above code, a recursive function named findFibonacciNumber is used to find the Fibonacci number of the user-entered sequence. It has a parameter named n of integer type. It gets the sequence of the Fibonacci series from the main () function and stored in the parameter named n. When the value of the Fibonacci sequence is 0 or 1 then this function returns 1. For the other values this function return the Fibonacci number for the given sequence by adding the immediate last two Fibonacci number by calling itself recursivelyand decreasing the value of the parameter by 1 (to get the immediate last value) and 2 (to get the second last value).
In the main function, create a variable named seq of integer type to store the user-entered Fibonacci sequence. Prompt the user to enter the Fibonacci sequence. Store the user-entered Fibonacci sequence in the variable name seq. Call the function named findFibonacciNumber with seq as parameter. Display the function returned value.
Sample output:
Want to see more full solutions like this?
Chapter 13 Solutions
Absolute C++
- (Program) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters, such as zerocount, onecount, twocount, and so forth, to 0. Then generate a large number of pseudorandom integers between 0 and 9. Each time 0 occurs, increment zerocount; when 1 occurs, increment onecount; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of time they occurred.arrow_forwardIn C programming Mathematically, given a function f, we recursively define fk(n) as follows: if k = 1, f1(n) = f(n). Otherwise, for k > 1, fk(n) = f(fk-1(n)). Assume that there is an existing function f, which takes in a single integer and returns an integer. Write a recursive function fcomp, which takes in both n and k (k > 0), and returns fk(n). int f(int n);int fcomp(int n, int k){arrow_forwardImplement a recursive C++ function which takes a character (ch) and a positive integer (n) and prints thecharacter ch, n times on the screen. The prototype of your function should be:void printChar (char ch, int n)For example, calling printChar('*',5) should display ***** on screen.Note: There should NOT be any loop in your function.arrow_forward
- Write a recursive function, reverseDigits, that takes an integer as a parameter and returns the number with the digits reversed. Also, write a program to test your function.arrow_forwardfor C++ write a progam for the greatest common divisor of integers x and y is the largest integer that evenly divides both x and y. Write a recursive function gcd that returns the greatest common divisor of x and y. The gcd of x and y is defined recursively as follows. If y is 0 the answer is x; otherwise gcd(x, y) is gcd(y, x%y).arrow_forwardWrite a recursive function in f#, named specialSum, that has the followeing signature: int * int -> int, where sum(m,n) = m + (m +1) + (m+2) + ... + (m + (n-1)) + (m+n) for m >= 0 and n >= 0: (Hint use two clauses with (m,0) and (m,n) A PATTERNS.) start code with let rec specialSum (m,n) match m,n with | m,0 -> | m,n - >arrow_forward
- Write a recursive function using python for the problem: A palindrome is a sequence of characters which is the same when the sequence is reversed. Given an array A indexed from p to q containing characters, determine if the sequence of characters in A[p..q] forms a palindrome. Return True is a palindrome is formed and return False if it does not.arrow_forwardIn programming, a recursive function calls itself. The classical example is factorial(n), which can be defined recursively as n*factorial(n-1). Nonethessless, it is important to take note that a recursive function should have a terminating condition (or base case), in the case of factorial, factorial(0)=1. Hence, the full definition is: factorial(n) = 1, for n = 0 factorial(n) = n * factorial(n-1), for all n > 1 For example, suppose n = 5: // Recursive call factorial(5) = 5 * factorial(4) factorial(4) = 4 * factorial(3) factorial(3) = 3 * factorial(2) factorial(2) = 2 * factorial(1) factorial(1) = 1 * factorial(0) factorial(0) = 1 // Base case // Unwinding factorial(1) = 1 * 1 = 1 factorial(2) = 2 * 1 = 2 factorial(3) = 3 * 2 = 6 factorial(4) = 4 * 6 = 24 factorial(5) = 5 * 24 = 120 (DONE) Exercise (Factorial) (Recursive): Write a recursive method called factorial() to compute the factorial of the given integer. public static int factorial(int n) The recursive algorithm is:…arrow_forwardThe Polish mathematician Wacław Sierpiński described the pattern in 1915, but it has appeared in Italian art since the 13th century. Though the Sierpinski triangle looks complex, it can be generated with a short recursive function. Your main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth. API specification. When writing your program, exercise modular design by organizing it into four functions, as specified in the following API: public class Sierpinski { // Height of an equilateral triangle whose sides are of the specified length. public static double height(double length) // Draws a filled equilateral…arrow_forward
- Write a recursive function that takes a positive integer and returns the factorial of that integer. Attention, the function must be recursive, and its name must be "fatorial".arrow_forwardConsider the recursive procedure which computes the nth Fibonacci number is the one below. procedure Fl (n) //a function which returns the nth Fibonacci number.// if n < 2 then return(n) else return (F2(2,n,1,1)) endif end Fl procedure F2(i,n,x,y) if iarrow_forwardWrite a recursive function PrintPattern1 to print pattern that takes two integer arguments n and k. n is the starting number while k is the ending limit.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
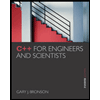
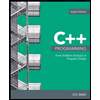