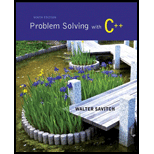
Problem Solving with C++ plus MyProgrammingLab with Pearson eText-- Access Card Package (9th Edition)
9th Edition
ISBN: 9780133862218
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 8PP
Program Plan Intro
Creation of program to simulate customer arrivals at motor vehicles department
Program Plan:
- Define a class “Queue” to denote methods and operations required for queue.
- Declare variables that are required for program.
- Define a constructor “Queue()” and assign values.
- Define a method “Enqueue()” to insert values into a queue.
- Define a method “Dequeue()” to remove value from a queue.
- Define a method “Front()” to return front element of queue.
- Define a method “Size()” to return size of queue.
- Define a method “isEmpty()” to check whether queue is empty or not.
- Define a main method
- Call method “Enqueue()” to insert values into a queue.
- Call method “Dequeue()” to remove value from a queue.
- Call method “Front()” to return front element of queue.
- Call method “Size()” to return size of queue.
- Call method “isEmpty()” to check whether queue is empty or not.
- Loop until the user needs to compute result.
- To simulate customer arrival call “Enqueue()” method with required parameters.
- To help next customer call “Dequeue()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
You need to insert the numbers2,4,3,7, one at a time in that order into to an initially empty queue. Represent that process using the standard constructors EmptyQueue and push.
Show, in the standard two-cell notation, the resulting queue.
What is the result of the operation top on that queue?
What is the result of the operation pop on the original queue you created?
What is the result of the operation pop followed by pop followed by top on the original queue you created?
Write a Java public static general method that doesn't belong to the Queue class(assuming the Queue type is Book and Book has a clone method, Book also has a method called getGenre() which returns the genre of the book):
Queue findOneGenreBooks(Queue Q, String genreType) that searches in the first parameter Q for all books that has the same genre type as defined by the second parameter genreType, return all of the books that have the specified genre type as a Queue. (For example, you may want to find all cartoon books and return them as a Queue). Please note: You are only allowed to use the Queue class which has constructors, enqueue, dequeue, front, isEmpty, size, toString, and clone methods.
Modify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run.
CODE TO MODIFY IN JAVA:
public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in…
Chapter 13 Solutions
Problem Solving with C++ plus MyProgrammingLab with Pearson eText-- Access Card Package (9th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Similar questions
- Implement solution for remove(int id) removes the Student (classt type) associated with this id; if the id is not found in the table or on the waitlist, then it should return null; otherwise, it should return the Student associated with the id. If the student that is removed was registered, then this student should be replaced by the student who is first in the waitlist queue. If the student who is removed was on the waitlist, then they should just be removed from the waitlist. You should go directly to slot id % m rather than iterating through all the slots. ****note that return is null**** public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList<Student>();…arrow_forwardImplement solution for remove(int id) removes the Student (classt type) associated with this id; if the id is not found in the table or on the waitlist, then it should return null; otherwise, it should return the Student associated with the id. If the student that is removed was registered, then this student should be replaced by the student who is first in the waitlist queue. If the student who is removed was on the waitlist, then they should just be removed from the waitlist. You should go directly to slot id % m rather than iterating through all the slots. public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList<Student>(); this.capacity = 10; }…arrow_forwardGiven the linked list data structure, implement a sub-class TSortedList that makes sure that elements are inserted and maintained in an ascending order in the list. So, given the input sequence {1,7,3,11,5}, when printing the list after inserting the last element it should print like 1, 3, 5, 7, 11. Note that with inheritance, you have to only care about the insertion situation as deletion should still be handled by the parent class.arrow_forward
- design a java program that will use a Linked list with an iterator to add new coaches and maintain the ascending order pattern of the good trainarrow_forwardProgramming in Java. What would the difference be in the node classes for a singly linked list, doubly linked list, and a circular linked list? I attached the node classes I have for single and double, but I feel like I do not change enough? Also, I use identical classes for singular and circular node which does not feel right. Any help would be appreciated.arrow_forwardConsider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons. We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list. Your implementation should be O(n) for add, and O(1) for the remaining methods. Consider the Person class in Problem 1. Implement the interface PersonPriorityQueueInterface provided in the assignment. In your implementation, you must use an instance of AList (which you used in Problem 1) to store the list of persons. We consider that a person whose age is higher than a second person also has a higher priority. Thus, the method peek(), for example, should return the person who is the oldest in the list. Your implementation should be O(n) for add, and O(1)…arrow_forward
- Implement the Double-Ended Queue class as it's been explained in section 6.3 of our textbook. Plz give me an examplearrow_forwardComplete the implementation of the isEmpty(), first(), size() and toString() methods. Also create a main() method class to write two or more test scenarios that will demonstrate the queue operation of all public methods including a scenario that adds values beyond the original capacity of the queue. Instantiating each queue with a relatively small capacity is recommended. Demonstrate that you can instantiate CircularArrayQueue classes with different data types, enqueue several items, dequeue several items, display the queue contents after each operation, attempt dequeueing from an empty queue. Please add comments so I can understand your code. CircularArrayQueue.java public class CircularArrayQueue<T> implements QueueADT<T>{private final static int DEFAULT_CAPACITY = 100;private int front, rear, count;private T[] queue; public CircularArrayQueue (int initialCapacity){front = rear = count = 0;queue = (T[]) (new Object[initialCapacity]);} public…arrow_forwardThe implementation for the priority queue in this chapter starts by modifying theHeap class so that the order of the elements can be determined by the programmer.Earlier the heap kept the highest value on the top of the container, but now it willbe altered to allow for either the highest or the lowest. The changes made to thisclass involve specifying another template parameter, which will be used for thecomparison operator. Altering the push() and pop() functions to make use of thecomparison operator instead of assuming that the largest value element will be ontop. use c++ to code.arrow_forward
- Programming homework1) Simulated waiting queue in an Indian post office: In an Indian post office that not only distributes mail but also performs some financial activities like a savings bank, a lone postal worker attends to a single lineup of clients. As soon as a consumer enters the line, they are given a token # (serial number). After receiving assistance, the consumer exits the queue while the postal worker receives the token back. The employee could inquire as to how many consumers are still unattended at any one time.i) Put the system into practise using a suitable queue data structure, simulating the arrival and departure of consumers at random when the service is finished.arrow_forwardThis is a java question Trace through the state of the queue q in the following code fragment in the main method. You need to show the queue with its values for credit. Queue <Integer> q = new ArrayDeque<Integer>( );q.add(14);q.add(-47);q.offer(75);q.poll( );Integer v = q.peek( );q.add( v - 32);q.remove( );q.add(q.poll( ));System.out.println ("q has " + q);System.out.println ("v has " + v); Final Result for q: v:arrow_forwardWrite a recursive instance method named findBad that takes no parameters and returns a reference to a Link. The result of calling findBad is a reference to the first link that has a value greater than the next link's value. If no such link exists return null. For example, for {5→6→7→3→2} it would return a reference to the third link ("7").arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
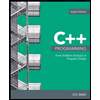
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning