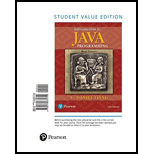
Program Plan:
- Import required packages.
- Declare a main class named “ch14_1” which extends the “Application” class.
- Declare a “start ()” method which overrides the “start ()” method in the “Application” class. Inside this method,
- Create a GridPane in order to display images.
- Place the pane in center position using “setAlignment ()” method.
- Set the horizontal and vertical gap for the pane using “setHgap ()” and “setVgap ()” functions respectively.
- Create four ImageView objects to display all the images from the file.
- Add all the four images on their respective position on the pane.
- Create a scene and place it on the stage.
- Set the title as “Exercise14_01”.
- Display the stage on the window using “primaryStage.show()” method.
- Declare a main method using “public static main”.
- Launch the method using “launch ()” method.
- Declare a “start ()” method which overrides the “start ()” method in the “Application” class. Inside this method,

The below program displays four images on the GridPane as per the given image.
Explanation of Solution
Program:
//Import required packages
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
import javafx.scene.image.ImageView;
//Main class extends Application
public class ch14_1 extends Application
{
//Overrides the start method in the application
@Override
//start method
public void start(Stage primaryStage)
{
//Create a GridPane to display images
GridPane GP = new GridPane();
//Place the pane in center position
GP.setAlignment(Pos.CENTER);
/*set the horizontal and vertical gap for the pane*/
GP.setHgap(5);
GP.setVgap(5);
/*Create 4 image views to display 4 images and pass the url from the file name*/
ImageView IV1 = new ImageView("snaps/germany.gif");
ImageView IV2 = new ImageView("snaps/china.gif");
ImageView IV3 = new ImageView("snaps/fr.gif");
ImageView IV4 = new ImageView("snaps/us.gif");
/*Add image to the pane in the first row, first column*/
GP.add(IV1, 0, 0);
/*Add image to the pane in the second row, first column*/
GP.add(IV2, 1, 0);
/*Add image to the pane in the first row, second column*/
GP.add(IV3, 0, 1);
/*Add image to the pane in the second row, second column*/
GP.add(IV4, 1, 1);
//Create a scene and place it on the stage
Scene scene = new Scene(GP);
//Setting title
primaryStage.setTitle("Exercise14_01");
//Place the scene on the stage
primaryStage.setScene(scene);
//Display the stage on the window
primaryStage.show();
}
//Main method
public static void main(String[] args)
{
//Launch the application
launch(args);
}
}
Screenshot of the output
Want to see more full solutions like this?
Chapter 14 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
- 5.23 LAB: Adjust values in a list by normalizing When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This adjustment can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print('{:.2f}'.format(your_value)) Ex: If the input is: 5 30.0 50.0 10.0 100.0 65.0 the output is: 0.30 0.50 0.10 1.00 0.65 The 5 indicates that there are five floating-point values in the list, namely 30.0, 50.0, 10.0, 100.0, and 65.0. 100.0 is the largest value in the list, so each value is divided by 100.0. Use Python, please.arrow_forward8.15 (Displaying a Sentence with Its Words Reversed) Write a program that inputs a line of text, tokenizes the line with function strtok and outputs the tokens in reverse order Note:solve the program without using pointers and in c languagearrow_forward6.28 In Python, Write a program that replaces words in a sentence. The input begins with word replacement pairs (original and replacement). The next line of input is the sentence where any word on the original list is replaced. Ex: If the input is: automobile car manufacturer maker children kids The automobile manufacturer recommends car seats for children if the automobile doesn't already have one. the output is: The car maker recommends car seats for kids if the car doesn't already have one. You can assume the original words are unique. END WITH A NEW LINEarrow_forward
- 6.28 In Python, Write a program that replaces words in a sentence. The input begins with word replacement pairs (original and replacement). The next line of input is the sentence where any word on the original list is replaced. Ex: If the input is: automobile car manufacturer maker children kids The automobile manufacturer recommends car seats for children if the automobile doesn't already have one. the output is: The car maker recommends car seats for kids if the car doesn't already have one. You can assume the original words are unique.arrow_forward6 43 2 Let A-7 3 4 6 5. 1. Using the column operator (;); create a column vector that contains all the columns of Aarrow_forward7.19 LAB: Adjust list by normalizing When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain less than 20 floating-point values. For coding simplicity, follow every output value by a space, including the last one. And, output each floating-point value with two digits after the decimal point, which can be achieved as follows:printf("%0.2lf ", yourValue); Ex: If the input is: 5 30.0 50.0 10.0 100.0 65.0 the output is: 0.30 0.50 0.10 1.00 0.65 The 5 indicates that there are five floating-point values in the list, namely 30.0, 50.0, 10.0, 100.0, and 65.0. 100.0 is the largest value in the list, so each value is divided by 100.0.…arrow_forward
- 8.7 (Converting Strings to Integers for Calculations) Write a program that inputs six strings t hat represent integers, converts the strings to integers, and calculates the sum and average of the six values. Solve the problem step by step in c without using pointers And Make sure to provide the text based code tooarrow_forward3.6.5: Printing array elements separated by commas. Write a for loop to print all NUM_VALS elements of array hourlyTemp. Separate elements with a comma and space. Ex: If hourlyTemp = {90, 92, 94, 95}, print:90, 92, 94, 95 Your code's output should end with the last element, without a subsequent comma, space, or newline.arrow_forward6.17 LAB: Swapping variables Define a method named swapValues that takes an array of four integers as a parameter, swaps array elements at indices 0 and 1, and swaps array elements at indices 2 and 3. Then write a main program that reads four integers from input and stores the integers in an array in positions 0 to 3. The main program should call function swapValues() to swap array's values and print the swapped values on a single line separated with spaces.arrow_forward
- 13.14 LAB: Library book sorting Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in ArrayList class (ArrayListLibrary arrayListLibrary). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number. Complete main() by inserting a new book into each list using the respective LinkedListLibrary and ArrayListLibrary insertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each insertSorted() returns the number of operations the computer performs. Ex: If the input is: The Catcher in the Rye 9787543321724 J.D. Salinger the output is: Number of linked list operations: 1 Number of ArrayList operations: 1 Which list do you think will require the most operations? Why?arrow_forward13.14 LAB: Library book sorting Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary linkedListLibrary) and the other implemented using the built-in ArrayList class (ArrayListLibrary arrayListLibrary). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number. Complete main() by inserting a new book into each list using the respective LinkedListLibrary and ArrayListLibrary insertSorted() methods and outputting the number of operations the computer must perform to insert the new book. Each insertSorted() returns the number of operations the computer performs. Ex: If the input is: The Catcher in the Rye 9787543321724 J.D. Salinger the output is: Number of linked list operations: 1 Number of ArrayList operations: 1 Which list do you think will require the most operations? Why?…arrow_forwardC++: Using the code in image: 1. Write your own main and insert function to insert the sequence of integers { 138, 99, 16, 134, 42, 0, 6, 9, 4, 53, 47, 66) using a table of size 17. 2.Implement your own rehashing algorithm of choice and run the same sequence of input using a table of size 7.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
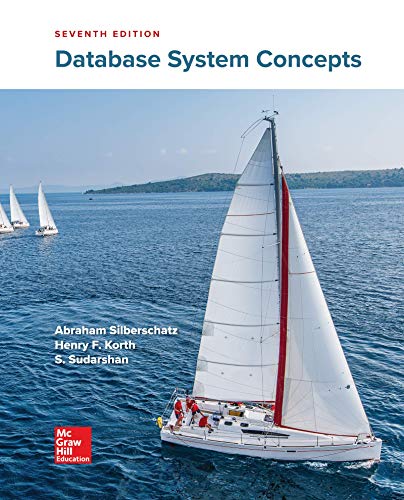
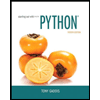
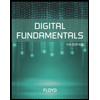
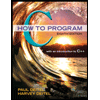
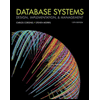
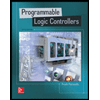