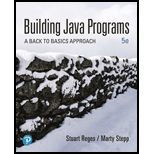
Rearrange stack of integers
Program plan:
- Import necessary packages.
- Create a class “StackSplit”,
- Define the method “splitstack ()” that accepts stack of integers,
- Construct “LinkedList” object.
- Calculate the stack size.
- Execute till stack is empty,
- Transfer all the stack elements from stack to queue.
- Execute loop till the stack size,
- Assign the element removed from the queue to the variable.
- Check whether the element is negative,
- Insert the element into the stack.
-
- Otherwise,
- Insert the element into the queue.
- Otherwise,
- Execute till queue is empty,
- Transfer non-negative integers from queue to stack.
- Print stack of integers after rearranged.
- Define the method “main()”,
- Create object for “Stack”.
- Add integers into the stack.
- Print the stack of integers.
- Call the method “splitStack()” with stack as parameter.
- Define the method “splitstack ()” that accepts stack of integers,

This program demonstrates the method that accepts a stack of integers and rearranges its elements.
Explanation of Solution
Program:
File name: “StackSplit.java”
//Import necessary packages
import java.util.LinkedList;
import java.util.Queue;
import java.util.Stack;
//Create a class
class StackSplit
{
//Define the method
public static void splitStack(Stack<Integer> s)
{
//Construct LinkedList object
Queue<Integer> q1 = new LinkedList<Integer>();
//Calculate the stack size
int old_Length = s.size();
//Execute till stack is empty
while (!s.isEmpty())
{
//Transfer all elements from stack to queue
q1.add(s.pop());
}
//Execute till the stack size
for (int i = 1; i <= old_Length; i++)
{
//Assign the element removed from the queue
int n1 = q1.remove();
//check whether the element is negative
if (n1 < 0)
{
//Insert the element into the stack
s.push(n1);
}
else
{
//Insert the element into the queue
q1.add(n1);
}
}
//Execute till queue is empty
while (!q1.isEmpty())
{
/*Transfer non-negative integers from queue to stack*/
s.push(q1.remove());
}
//Print stack of integers
System.out.println("The elements of a stack of integers after rearranged: "+s);
}
//Define the main() method
public static void main(String[] args)
{
//Create object for Stack
Stack<Integer> s1=new Stack<Integer>();
//Add the integers into the stack
s1.push(3);
s1.push(-5);
s1.push(1);
s1.push(2);
s1.push(-4);
//Print the stack of integers
System.out.println("The elements of a stack of integers: "+s1);
//Call the method
splitStack(s1);
}
}
Output:
The elements of a stack of integers: [3, -5, 1, 2, -4]
The elements of a stack of integers after rearranged: [-4, -5, 2, 1, 3]
Want to see more full solutions like this?
Chapter 14 Solutions
MyProgrammingLab with Pearson eText -- Access Code Card -- for Building Java Programs
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
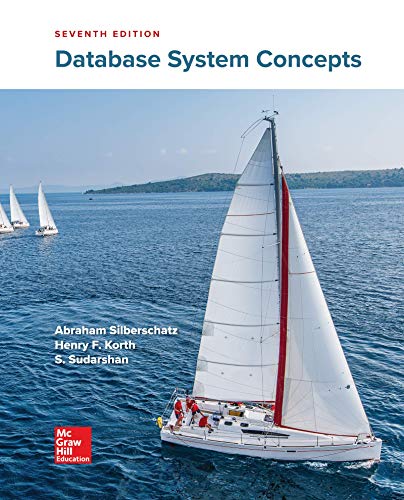
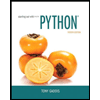
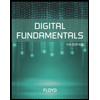
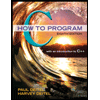
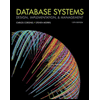
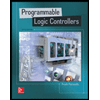