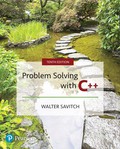
Concept explainers
Explanation of Solution
Recursive version of the function “indexOfSmallest”:
The recursive version of the function “indexOfSmallest” is shown below:
/* Recursive function definition for compute index of smallest element */
int indexOfSmallest(const int a[], int startIndex, int numberUsed)
{
/* Assign minimum to array of starting index */
int min = a[startIndex];
/* If starting index is equal to "numberUsed-1", then */
if(startIndex == numberUsed - 1)
/* Returns the starting index */
return startIndex;
/* Otherwise recursively call the function "indexOfSmallest" */
int indexOfMin = indexOfSmallest(a, startIndex+1, numberUsed);
/* If the value of "min" is greater than "a[indexOfMin]" */
if(min > a[indexOfMin])
/* Returns index of minimum value */
return indexOfMin;
//Otherwise
else
/* Returns the starting index */
return startIndex;
}
Complete executable program code:
The modified complete code is implemented for given function is given below:
//Header file
#include <iostream>
//Function declaration
void fillArray(int a[], int size, int& numberUsed);
//Precondition: size is the declared size of the array a.
//Postcondition: numberUsed is the number of values stored in a.
//a[0] through a[numberUsed - 1] have been filled with
//nonnegative integers read from the keyboard.
void sort(int a[], int numberUsed);
//Precondition: numberUsed <= declared size of the array a.
//The array elements a[0] through a[numberUsed - 1] have values.
//Postcondition: The values of a[0] through a[numberUsed - 1] have
//been rearranged so that a[0] <= a[1] <= ... <= a[numberUsed - 1].
void swapValues(int& v1, int& v2);
//Interchanges the values of v1 and v2.
int indexOfSmallest(const int a[], int startIndex, int numberUsed);
//Precondition: 0 <= startIndex < numberUsed. Referenced array elements have
//values.
//Returns the index i such that a[i] is the smallest of the values
//a[startIndex], a[startIndex + 1], ..., a[numberUsed - 1].
//Main function
int main( )
{
//For standard input and output
using namespace std;
//Prompt statement
cout << "This program sorts numbers from lowest to highest.\n";
//Declare variables
int sampleArray[10], numberUsed;
//Call fill array function
fillArray(sampleArray, 10, numberUsed);
//Prompt statement
cout << "Index of minimum number in given array: ";
//Call the function "indexOfSmallest"
cout << indexOfSmallest(sampleArray, 0, numberUsed) << endl;
//Call the function "sort"
sort(sampleArray, numberUsed);
//Prompt statement
cout << "In sorted order the numbers are:\n";
//Display the sorted number using "for" loop
for (int index = 0; index < numberUsed; index++)
cout << sampleArray[index] << " ";
cout << endl;
return 0;
}
//Uses iostream:
//Function definition for fill array
void fillArray(int a[], int size, int& numberUsed)
{
//For standard input and output
using namespace std;
//Prompt statement
cout << "Enter up to " << size << " nonnegative whole numbers.\n" << "Mark the end of the list with a negative number...

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Problem Solving with C++ (10th Edition)
- Write a function in a racket code that takes as input a list of elements and returns a list with elements and their position after. For example, if the input to the function is (list ’a ’b ’c) then it will return (list a 1 b 2 c 3). Make sure to use recursionarrow_forwardWrite a recursive function named Multiply2 that multiples by 2 and prints each element in a given list. For example: mylist=[1,2,3,4] Multiply2(mylist) prints: 2 4 6 8arrow_forwardBelow,enter code to complete implementation of a recursive function sum_all_integers(), which takes an input n and adds all intergers preceding it, up to n: add_all_integers(n):arrow_forward
- implement two recursive versions of the linear search that gets an array of songs and a title and searches for a song with the given title in the array. In this version, the function returns the first index of the array containing the number. If the number is not in the array, the function returns -1. int linear_search_rec_first(int* ar, int length, int number);arrow_forwardIn C language, implement two recursive versions of the linear search that gets an array of songs and a title and searches for a song with the given title in the array. 1. In this version the function returns the first index of the array containing the number. If the number is not in the array, the function returns -1. int linear_search_rec_first(int* ar, int length, int number); 2. In this version the function returns the last index of the array containing the number. If the number is not in the array, the function returns -1. int linear_search_rec_last(int* ar, int length, int number); Example of test for case 1: void test_q3() { int ar1[]={1,2,3,4,5,4,3,2,1}; if (linear_search_rec_first(ar1,9,2)==1) printf("Q3-1 ok\n"); else printf("Q3-1 ERROR\n"); } Example of test for case 2: void test_q3() { int ar1[]={1,2,3,4,5,4,3,2,1}; if (linear_search_rec_last(ar1,9,2) == 7) printf("Q3-3 ok\n"); else printf("Q3-3 ERROR\n"); }arrow_forwardWrite a recursive function named reverseWithinBounds that has an argument that is an array of characters and two arguments that are bounds on array indices. The function should reverse the order of those entries in the array whose indices are between the two bounds (including the bounds). For example, if the array is: a[0] == 'A' a[1] == 'B' a[2] == 'C' a[3] == 'D' a[4] == 'E' and the bounds are 1 and 4, then after the function is run the array elements should be: a[0] == 'A' a[1] == 'E' a[2] == 'D' a[3] == 'C' a[4] == 'B' Embed the function in a program and test it. After you have fully debugged this function, define another function named reverseCstring that takes a single argument that is a C string and modifies the argument so that it is reversed. This function will include a call to the recursive definition you did for the first part of this project, and need not be recursive. Embed this second function in a program and test it.arrow_forward
- Write a recursive function named reverseWithinBounds that has an argument that is an array of characters and two arguments that are bounds on array indices. The function should reverse the order of those entries in the array whose indices are between the two bounds (including the bounds). For example, if the array is: a[0] == 'A' a[1] == 'B' a[2] == 'C' a[3] == 'D' a[4] == 'E' and the bounds are 1 and 4, then after the function is run the array elements should be: a[0] == 'A' a[1] == 'E' a[2] == 'D' a[3] == 'C' a[4] == 'B' Embed the function in a program and test it. After you have fully debugged this function, define another function named reverseCstring that takes a single argument that is a C string and modifies the argument so that it is reversed. This function will include a call to the recursive definition you did for the first part of this project, and need not be recursive. Embed this second function in a program and test it. Turn in only this final result (with output,…arrow_forwardWrite a recursive function named reverseWithinBounds that has an argument that is an array of characters and two arguments that are bounds on array indices. The function should reverse the order of those entries in the array whose indices are between the two bounds (including the bounds). For example, if the array is: a[0] == 'A' a[1] == 'B' a[2] == 'C' a[3] == 'D' a[4] == 'E' and the bounds are 1 and 4, then after the function is run the array elements should be: a[0] == 'A' a[1] == 'E' a[2] == 'D' a[3] == 'C' a[4] == 'B'arrow_forwardImplement a recursive C++ function which takes an integer array (A) and the starting (start) and ending(end) indices of that array, and returns the sum of all elements present in that array. The prototype ofyour function should be:int findSum (int* A, int start, int end)arrow_forward
- By using java, Write a recursive function that finds the maximum element in an array ofintegers, based on comparing the first element in the array against themaximum in the rest of the array recursively.arrow_forwardWrite a recursive function that finds the minimum value in an ArrayList. Your function signature should be public static int findMinimum(ArrayList<Integer>) One way to think of finding a minimum recursively is to think “the minimum number is either the last element in the ArrayList, or the minimum value in the rest of the ArrayList”. For example, if you have the ArrayList [1, 3, 2, 567, 23, 45, 9], the minimum value in this ArrayList is either 9 or the minimum value in [1, 3, 2, 567, 23, 45] ================================================ import java.util.*; public class RecursiveMin{public static void main(String[] args){Scanner input = new Scanner(System.in);ArrayList<Integer> numbers = new ArrayList<Integer>();while (true){System.out.println("Please enter numbers. Enter -1 to quit: ");int number = input.nextInt();if (number == -1){break;}else {numbers.add(number);}} int minimum = findMinimum(numbers);System.out.println("Minimum: " + minimum);}public static int…arrow_forwardConstruct recursive versions of the library functions that: a. calculate the "sum" of a list of numbers. b. "take" a given number of elements from the beginning of a list. c. select the "last" element of a non-empty list .arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
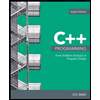