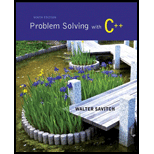
Explanation of Solution
Recursive function for checking palindrome:
The recursive function definition for checking the given string is a palindrome or not is shown below:
/* Function definition for checkPalindromeRecursion function */
bool checkPalindromeRecursion(string str)
{
/* If length is "0" or "1", then return "true" */
if(str.length() == 0 || str.length() == 1)
return true;
/* Check the first character*/
if(isdigit(str.at(0)))
{
/* If the first character is equal to the last character, then */
if(str.at(0) == str.at(str.length()-1))
/* Recursively call the function "checkPalindromeRecursion" with remaining characters */
return checkPalindromeRecursion(str.substr(1, str.length()-2));
}
//Otherwise
else
{
//For checking the lower characters
if(tolower(str.at(0)) == tolower(str.at(str.length()-1)))
return checkPalindromeRecursion(str.substr(1, str.length()-2));
}
//Otherwise returns "false"
return false;
}
Explanation:
The above function is used to check the given string is a palindrome or not using recursive function.
- If the length of string is “0” or “1”, then returns “true”.
- Check the first digit of given string. If it is, then check if the first character is equal to the last character, then recursively call the function “checkPalindromeRecursion” for remaining characters in given string.
- Otherwise, return “false” that is for not palindrome string.
Complete Executable code:
The complete code is implemented for checking the palindrome is shown below:
//Header file
#include <iostream>
#include <iomanip>
//For standard input and output
using namespace std;
/* Function definition for checkPalindromeRecursion function */
bool checkPalindromeRecursion(string str)
{
/* If length is "0" or "1", then return "true" */
if(str...

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Problem Solving with C++ plus MyProgrammingLab with Pearson eText-- Access Card Package (9th Edition)
- Write a recursive function PrintPattern1 to print pattern that takes two integer arguments n and k. n is the starting number while k is the ending limit.arrow_forwardWrite a recursive function called print_num_pattern() to output the following number pattern. Given a positive integer as input (Ex: 12), subtract another positive integer (Ex: 3) continually until 0 or a negative value is reached, and then continually add the second integer until the first integer is again reached. For coding simplicity, output a space after every integer, including the last. Do not end output with a newline. Ex. If the input is: 12 3 the output is: 12 9 6 3 0 3 6 9 12 # TODO: Write recursive print_num_pattern() function if __name__ == "__main__": num1 = int(input()) num2 = int(input()) print_num_pattern(num1, num2)if (num1<=0): print(num1,end= '')arrow_forwardFor function log, write the missing base case condition and the recursive call. This function computes the log of n to the base b. As an example: log 8 to the base 2 equals 3 since 8 = 2*2*2. We can find this by dividing 8 by 2 until we reach 1, and we count the number of divisions we make. You should assume that n is exactly b to some integer power. Examples: log(2, 4) -> 2 and log(10, 100) -> 2 public int log(int b, int n ) { if <<Missing base case condition>> { return 0; } else { return <<Missing a Recursive case action>> }}arrow_forward
- The Polish mathematician Wacław Sierpiński described the pattern in 1915, but it has appeared in Italian art since the 13th century. Though the Sierpinski triangle looks complex, it can be generated with a short recursive function. Your main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth. API specification. When writing your program, exercise modular design by organizing it into four functions, as specified in the following API: public class Sierpinski { // Height of an equilateral triangle whose sides are of the specified length. public static double height(double length) // Draws a filled equilateral…arrow_forwardConsider the following function: void fun_with_recursion(int x) { printf("%i\n", x); fun_with_recursion(x + 1); } What will happen when this function is called by passing it the value 0?arrow_forwardRefine the is_palindrome function to work with arbitrary strings, by ignoring non-letter characters and the distinction between upper- and lowercase letters. For example, if the input string is "Madam, I’m Adam!" then you’d first strip off the last character because it isn’t a letter, and recursively check whether the shorter string "Madam, I’m Adam" is a palindrome. c++arrow_forward
- Below,enter code to complete implementation of a recursive function sum_all_integers(), which takes an input n and adds all intergers preceding it, up to n: add_all_integers(n):arrow_forwardA palindrome is a sentence that contains the same sequence of letters reading it either forwards or backwards. A classic example is '1\.ble was I, ereI saw Elba." Write a recursive function that detects whether a string is apalindrome. The basic idea is to check that the first and last letters of thestring are the same letter; if they are, then the entire string is a palindromeif everything between those letters is a palindrome.There are a couple of special cases to check for. If either the first orlast character of the string is not a letter, you can check to see if the restof the string is a palindrome with that character removed. Also, when youcompare letters, make sure that you do it in a case-insensitive way.Use your function in a program that prompts a user for a phrase andthen tells whether or not it is a palindrome. Here's another classic fortesting: '1\. man, a plan, a canal, Panama!"arrow_forwardI just need the method they are asking for Write a recursive function named checkPalindrome that takes a string as input, and returns true if the string is a palindrome and false if it is not a palindrome. A string is a palindrome if it reads the same forwards or backwards. Recall that str.charAt(a) will return the character at position a in str. str.substring(a) will return the substring of str from position a to the end of str, while str.substring(a, b) will return the substring of str starting at position a and continuing to (but not including) the character at position b. Examples: checkPalindrome("madam") -> true public boolean checkPalindrome(String s) { }arrow_forward
- Write a recursive function that returns the sum of the digits of an integer.int sumOfDigits(int x);arrow_forwardWrite a Lisp function called reverse that recursively reverses a string. In order to put the recursive call at the end of the function, do it this way: concatenate the last character in the string with the result of making a recursive call and sending everything *but* the last letter. Test your function with this code and paste both the code and the output into the window (reverse "")(reverse "a")(reverse "ab")(reverse "abc")(reverse "abcd")arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
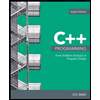