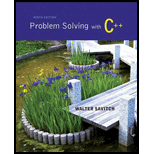
Solution to Programming Project 15.10
Listed below is code to play a guessing game. In the game two players
attempt to guess a number. Your task is to extend the program with objects that represent either a human player or a computer player. The rand() function requires you include cstdlib (see Appendix 4):
bool checkForWin(int guess, int answer) { cout<< "You guessed" << guess << "."; if (answer == guess) { cout<< "You're right! You win!" <<endl; return true; } else if (answer < guess) cout<< "Your guess is too high." <<endl; else cout<< "Your guess is too low." <<endl; return false; } void play(Player &player1, Player &player2) { int answer = 0, guess = 0; answer = rand() % 100; bool win = false; while (!win) { cout<< "Player 1's turn to guess." <<endl; guess = player1.getGuess(); win = checkForWin(guess, answer); if (win) return; cout<< "Player 2's turn to guess." <<endl; guess = player2.getGuess(); win = checkForWin(guess, answer); } } |
The play function takes as input two Player objects. Define the Player class with a virtual function named getGuess(). The implementation of Player::getGuess() can simply return 0. Next, define a class named HumanPlayer derived from Player. The implementation of HumanPlayer::getGuess() should prompt the user to enter a number and return the value entered from the keyboard. Next, define a class named ComputerPlayer derived from Player. The implementation of ComputerPlayer::getGuess() should randomly select a number between 0and 99 (see Appendix 4 for information on random number generation).Finally, construct a main function that invokes play(Player &player1, Player &player2) with two instances of a HumanPlayer (human versus human), an instance of a HumanPlayer and Computer Player (human versus computer),and two instances of ComputerPlayer (computer versus computer).

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
C++ How to Program (10th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with C++: Early Objects
Artificial Intelligence: A Modern Approach
- Write a Java function called SpOdd, so that this function would take an array of integers as input and return an array of integers with all the odd numbers in the original array. Make sure to keep the same order as the original array and also test your function in the main function. Sample output of the main function: The original Array: [3, 8, 5, 7, 1, 9, 2] The odd elements in the array: [3, 5, 7, 1, 9]arrow_forwardWrite a program that uses two classes. The first class is called “dAta” and holds x and y coordinates of a point in 2-d space called p1. The second class is called “cOmpute” and holds an array of two pointers to the “dAta” class. The “cOmpute” class has a function, lOad(float x, float y, int n), which loads x and y coordinate data into the array at index n. It also has a function, sLope(), which computes the slope of the line connecting the two array data coordiantes. It also has a function, pRint(), which prints the slope result to the screen. Implement a divide by zero exception using throw in the sLope() nd/or pRint() function as appropriate. The exception should, when caught, print “Slope calculation error…” to the screen and exit the function. The program should load the array with some example points and print the slope to the screen using the class functions.arrow_forwardWrite a python program that takes input values as integer, checks the number is not less than 10 (otherwise take input again) and prints the previous 10 numbers i.e. 10, 9, 8, … Concatenate the integer and string values and print as a string Write a python code to create a class named as ‘Employee’ with attributes i.e. ID, Name, Designation, Qualification, Salary, Taken-Leaves, Allowed-Leaves and the functions applyLeave(). This function will take number of leaves as an input and check Allowed-Leaves to see whether the employee can take leaves or not. If he can take, deduct these leaves from Allowed-Leaves and add in Taken-Leaves. Otherwise show message ‘you cannot take leave’. Create another class ‘Intern’ and inherit ‘Employee’ as super class.arrow_forward
- In this task, we’re going to be simulating an email message. ●Create a class definition for an Email.The initialiser takes in two arguments and stores them as instance-level variables:○from_address - the sender’s email address.○subject_line - the subject of the email.○email_contents-the content of the email.●In addition,the initialiser will create two more instance-level variables with defaultvalues:○has_been_read - initialised to False.○is_spam - initialised to False.●Create a function in this class called mark_as_read which should change has_been_read to true.●Create a function in this class called mark_as_spam which should change is_spam to true.●Create another class called "Inbox" to store all emails (note that you can have a list of objects). The initaliser doesn’t take any arguments, and only initialises an empty list. This list is where all of your Email objects will be stored.●Within the Inbox class, create the following methods:○add_email (self, from_address, subject_line,…arrow_forwardComputer Science In Java, create a class Die representing a die to roll randomly. -Give Die a public final static int FACES, representing how many faces all dice will have for this run of the program. -In a static block, choose a value for FACES that is randomly chosen from the options 4, 6, 8, 10, 12, and 20. -Give Die an instance variable current, representing the last value it rolled. (cannot be less than 1 or more than FACES) -Give Die int instance variables maxCount and minCount. (cannot be negative) -Give Die a method roll that randomly sets current to a value between 1 and FACES. If the die rolls a 1, increase minCount, if the die rolls FACES, increase maxCount. -Give Die a static method sumDice, which takes a variable length parameter list of Dies and returns the sum of all their current values. -Give Die a static method sameDice, which takes a variable length parameter list of Dies, and returns true only if all of them have the same current value. Do not compare…arrow_forwardReuse your Car class . In a main, build an object of that class, and print out the object using System.out.println(). Notice that this simply reports the memory address of the object in question, and we’d like to do something more useful. To replace (or override) the toString (or equals) function. Now, build a toString function that prints out the make, model, and odometer reading for a vehicle object. public class Car { //instance variables private int odometer; private String make; private String model; //overloading //constructors public Car(int odometer,String make, String model) { this.odometer = odometer; this.make = make; this.model = model; } public Car(String make, String model) { this.make = make; this.model = model; } public Car(String make) { this.make = make; } /* *getter & setter methods */ public int getOdometer() { return odometer; } public void…arrow_forward
- Write a java program to create a class Employee which contains member variables as name (String object)empid (Integer object)salary (Float object)age (Integer object)Define a default as well as a parameterized constructor to initialize member variables with default and specified values respectively. Define and overload a method search as defined below boolean search (Employee e[ ], String name) To search an employee with name in an array of employee objects, if found return true, else false.boolean search (Employee e[ ], Integer empid) To search an employee with the empid in an array of employee objects, if found return true, else false.Also define another method to get the name of employee having highest salary in a set of employees. String getHighestSalary(Employee e[ ]) To return the name of the employee with highest salary in an array of employee objects.Input: At least 3 employee details like name, empid, salary and age.Name of employee to searchId of employee to searchOutput:…arrow_forward1. How can we write a parameter less Lambda expression? a. Need to pass curly braces to denotes that there are no parameter on left side of the arrow.b. Pass empty set of parentheses on the left side of the arrow.c. In this particular case arrow is not required at all.d. No need to pass anything on the left side of the arrow. 2. Which of the following is NOT true about functional interface in Java? a. It has multiple methods that needs to be implemented.b. Lambda expression implicitly implement the single method inside functional interface.c. If a lambda expression is provided then the method name should not be provided.d. It has only a single method that needs to be implemented.arrow_forwardWrite code for. Computes the smallest x that satisfies the chinese remainder theorem for a system of equations. The system of equations has the form: x % nums[0] = rems[0] x % nums[1] = rems[1] ... x % nums[k - 1] = rems[k - 1] Where k is the number of elements in nums and rems, k > 0. All numbers in nums needs to be pariwise coprime otherwise an exception is raised returns x: the smallest value for x that satisfies the system of equations.arrow_forward
- Write a C++ program that will display multiple-choice trivia questions, accept the user's answers, and provide a full key and a score at the end. Design and implement a class called Question. The Question class contains the following information: a stem (the text of the trivia question) an array of 4 multiple choice answers (the text of each possible answer) the letter of the correct answer (A, B, C, or D), called the key. This class will be used to represent trivia questions in a trivia game. The following operations should be available for Question objects (use the provided names). Construct a Question with no values (use empty strings for stem and answers and 'X' for the key). Construct a Question given its 3 components (). setStem: Set the stem question. getStem: Return the stem question. setAnswers: Set the 4 answers given an array. The answers will NOT include the letter (A, B, C, or D). getAnswer(i): Return the single answer at index i. setKey: Set the key letter. getKey:…arrow_forwardWrite some code to generate an instance where variable x has both the static and dynamic types T and D, respectively.arrow_forwardConsider the following C++ code fragment: int a = 3; int b = 2; double r = a/b; Suppose we want r to have the value 1.5, rather than 1. Which cast operator can be used in the third line to achieve this effect? a. static cast b. dynamic cast c. reinterpret cast d. none of the abovearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
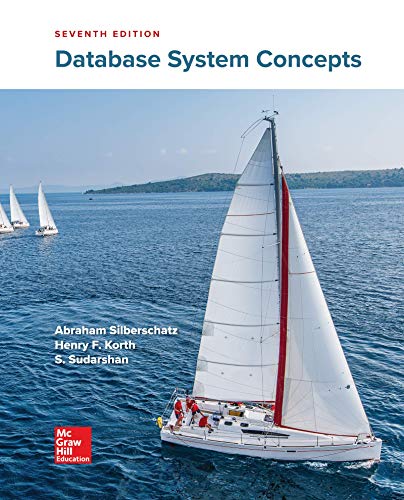
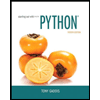
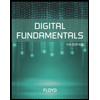
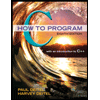
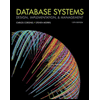
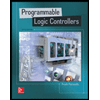