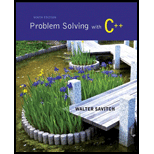
Concept explainers
Listed below are definitions of two classes that use inheritance, code for their implementation, and a main function. Put the code into appropriate files with the necessary include statements and preprocessor statements so that the program compiles and runs. It should output “Circle has radius 2 and area 12.5664”.
class Shape { public: Shape(); Shape(string name); string getName(); void setName(string newName); virtual double getArea() = 0; private: string name; }; Shape::Shape() { name=""; } Shape::Shape(string name) { this->name = name; } string Shape::getName() { return this->name; } void Shape::setName(string newName) { this->name = newName; } class Circle : public Shape { public: Circle(); Circle(int theRadius); void setRadius(int newRadius); double getRadius(); virtual double getArea(); private: int radius; }; Circle::Circle() : Shape("Circle"), radius(0) { } Circle::Circle(int theRadius) : Shape("Circle"), radius(theRadius) { } void Circle::setRadius(int newRadius) { this->radius = newRadius; } double Circle::getRadius() { return radius; } double Circle::getArea() { return 3.14159 * radius * radius; } int main() { Circle c(2); cout << c.getName() << " has radius " << c.getRadius() << " and area " << c.getArea() << endl; return 0; } |
Add another class, Rectangle, that is also derived from the Shape class. Modify the Rectangle class appropriately so it has private width and height variables, a constructor that allows the user to set the width and height, functions to retrieve the width and height, and an appropriately defined getArea function that calculates the area of the rectangle.
The following code added to main should output “Rectangle has width 3 has height 4 and area 12.0”.
Rectangle r(3,4); cout << r.getName() << " has width " << r.getWidth() << " has height " << r.getHeight() << " and area " << r.getArea() << endl; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ plus MyProgrammingLab with Pearson eText-- Access Card Package (9th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (12th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Software Engineering (10th Edition)
Digital Fundamentals (11th Edition)
- Question: 3Implement the design of the Ironman and DoctorStrange class derived from Avengers class so that the following code generates the output below:class Avengers: numberOfAvengers = 0 def __init__(self, name = None): self.name = name print('Welcome to Multiverse!') if self.name == None: print('Who is your favourite Super Hero?') else: print(f'{self.name} is your favourite Super Hero!') def hasSuperPower(self): print('All Avengers have super powers.') def fightThanos(self): return 'No avenger can fight Thanos'#Write your code here#-------------------------------------------------------------------------------------print('1.===============================')print('Number of Avengers:', Avengers.numberOfAvengers)print('2.===============================')a1 =…arrow_forwardWrite a java program to create a class Employee which contains member variables as name (String object)empid (Integer object)salary (Float object)age (Integer object)Define a default as well as a parameterized constructor to initialize member variables with default and specified values respectively. Define and overload a method search as defined below boolean search (Employee e[ ], String name) To search an employee with name in an array of employee objects, if found return true, else false.boolean search (Employee e[ ], Integer empid) To search an employee with the empid in an array of employee objects, if found return true, else false.Also define another method to get the name of employee having highest salary in a set of employees. String getHighestSalary(Employee e[ ]) To return the name of the employee with highest salary in an array of employee objects.Input: At least 3 employee details like name, empid, salary and age.Name of employee to searchId of employee to searchOutput:…arrow_forwardModify the above code to use the alternative method for multithreading (i.e., implementing the Runnable interface). Name your main class RunnableHelloBye and your subsidiary classes Hello and Goodbye respectively. The fi rst should display the message ‘Hello!’ ten times (with a random delay of 0–2 s between consecutive displays), while the second should do the same with the message ‘Goodbye!’. Note that it will NOT be the main class that implements the Runnable interface, but each of the two subsidiary classes.arrow_forward
- Take this the Java Gringrots problem below turn it into a running program. where the main should find out how many galleons are from 100,000 knuts. : " Write a Java program using a class identified as Gringots.This Java class has a main, and the following protected member functions: Member function galleons2Sickles has an integer parameter value, and returns an integer value*17Member function sickles2galleons has an integer parameter value, and returns an integer value / 17Member function sickles2nuts has an integer parameter value, and returns an integer value*29Member function knuts2sickles has an integer parameter value, and returns an integer value/29 Member function Galleons2Knuts has an integer parameter value, and then uses member functions Galleons2Sickles and Sickles2Knuts to convert, and then returns the integer. Member function Knuts2Galleons has an integer parameter value, and then uses member functions Knuts2Sickles and Sickles2Galleons to convert, an dthen returns the…arrow_forwardCreate a class MyTime which has the datamembers as follows: 1. hour: integer (1 to 12)2. minute: integer (0 to 59)3. second: integer (0 to 59)4. pm: bool variable. True means PM time and false means AM time. Implement a default constructor, a parameterized constructor and a copy constructor. Write set and get functions for all the four members of the class. Overload these operators: 1. Extraction operator >> : Prompt the user for hours, minutes, seconds and for am/pm and initialize the structure. Left operand istream object and right operand MyTime2. Assignment = : Assign the right object to the left object. Both operands are of type MyTime.3. Insertion << : Print the time in the format HH:MM SS PM. Left operand of type ostream and right operand of type MyTime. this in c++.arrow_forwardWrite a class definition that creates a class called leverage with one private data member, crowbar, of type int and one public function whose declaration is void pry(). Opp Javaarrow_forward
- Write a java program: Write the definition of a class named Employee to hold the data of the working hours of employees and their salaries in a company. The class includes the following private data members: • Name: to represent employee's name • ID: to represent employee's ID • NumberHours: an array of type integer of size 24 to save the number of hours worked by the employee during the days of the month. • Price: to represent the price per hour. Additionally, the class should include: • A constructor that takes three parameters to initialize the instance variables Name, ID and price and create the array NumberHours. • A method SetHours. The method should ask the user to enter the number of hours worked in each day (fill the array). · A method TotalAmount that returns the amount which should be paid by the company to the employee. If the total worked hours exceeds 150, the company should pay an additional bonus of 10% of the total amount. Thus, normal payment is calculated as =…arrow_forwardThis question concerns writing a Student class to model a student's name, composed of a first name, middle name and last name. The Student class should meet the following specification:Class StudentA Student object represents a student. A student has a first name, middle name and last name.Methodspublic void setNames(String first, String middle, String last)// Set the first, middle and last names of this Student object.public String getFullName()// Obtain the full name of this Student with the middle name converted to an initial only.2Thinking of the problem in terms of testing, there are three requirements that must be met:1. The setNames() method sets the names stored in the object i.e. the values of firstName, middleName, lastName.2. The getFullName() method obtains the name of the student with the middle name converted to an initial only.3. The getFullName() method does not change anything – i.e. it does not modify the values of firstName, middleName, lastNameHINT: To start your…arrow_forwardDesign Naruto class and MyHeroAcademia class which inherit from Anime class so that the following code provides the expected output. You can not change any of the given code. Do not modify the given parent class.Note: add_character() method in both child classes should work for any number of parameters and assume parameters will be even numbers.class Anime: def __init__(self, name, rating, genre): self.name = name self.rating = rating self.genre = genre def add_character(self, *info): pass def __str__(self): s = f"Name: {self.name}\nRating: {self.rating}\nGenre: {self.genre}" return s# Write your codes here.naruto = Naruto("Naruto", 10, "Adventure Fiction", 2007)naruto.add_character("Naruto Uzumaki", "Main", "Itachi Uchiha", "Main", "Madara Uchiha", "Anti Hero", "Pain", "Supporting", "Shikamaru Nara",…arrow_forward
- Q2: Consider the following statements: public class YClass { private int a; private int b; public void one() { } public void two(int x, int y); { } public YClass() { } } class XClass extends YClass { private int z; public void one() { } public XClass() { } } YClass yObject; XClass xObject; Write the definition of the default constructor of YClass so that the instance variables of YClass are initialized to 0. Write the definition of the default constructor of XClass so that the instance variables of XClass are initialized to 0. Write the definition of the method two of YClass so that the instance variable a is initialized to the value of the first parameter of two and the instance variable b is initialized to the value of the second parameter of two. please I want solution :(arrow_forwardBuild a class Pet having the following data membersa. Name (String)b. Gender (char)c. Type (String)d. Age (int)e. Weight (float)f. healthCondition (int)Imagine this class Pet represents your pet as a virtualobject. Provide constructors with arguments for name,gender, type, age and weight. Initialize healthCondition to 5(which means healthy). Provide getter for each of these but setter for only weight. Provide a function eat(String food). This function represents the action of eating thatwould increase the weight of the pet according to following rulesFood Increase In WeightRed Meat 0.14 kgChicken 0.12 kgPet Food 0.17 kgSupplement 0.1 kgFish 0.09 kgThe eat function returns the new Weight of the pet object.Provide another function fallSick() that reduces the healthCondition of the pet 1 but it cannot gobelow 0. Provide a function bool isDead() this function returns true if the healthCondition of a pet is 0and false otherwise. Add another function recover(). This function increases…arrow_forward???????? 2: Design the required class(es) so that the following expected output is generated. Do not change any given code. Your code should work for any number of arguments in init and setToppings method of pizza class. For simplicity, you can consider there will be only 3 categories of pizzas and they are: Chicken, Beef, Cheese. #Write your code here dom = Dominos("Domino's Pizza") print("1.=================================") p1 = Pizza("Spicy Chicken","Spicy Chicken","Onion","Capsicum") dom.addPizza(p1) p2 = Pizza("Margherita","Mozzarella Cheese") print("2.=================================") p3 = Pizza("Beef Kala Bhuna") print("3.=================================") p3.setToppings("Curried Beef","Capsicum","Paprika","Onion") print("4.=================================") dom.addPizza(p2,p3) p4 = Pizza("Chicken Dominator","Barbecue Chicken,Spicy Chicken,Grilled Chicken") p5 = Pizza("Beefzza","Minced Beef","Beef Pepperoni","Onion","Jalapeno") dom.addPizza(p4,p5)…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
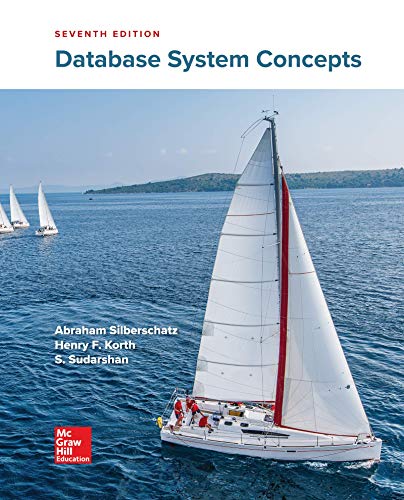
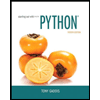
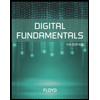
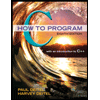
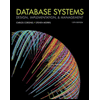
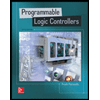