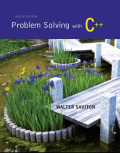
Radio Frequency IDentification (RFID) chips are small tags that can beplaced on a product. They behave like wireless barcodes and can wirelessly broadcast an identification number to a receiver. One application of RFID chips is to use them to aid in the logistics of shipping freight. Consider a shipping container full of items. Without RFID chips, a human has to manually inventory all of the items in the container to verify the contents. With an RFID chip attached to the shipping container, the RFID chip can electronically broadcast to a human the exact contents of the shipping container without human intervention.
To model this application, write a base class called ShippingContainer that has a container ID number as an integer. Include member functions to set and access the ID number. Add a virtual function called getManifest that returns an empty string. The purpose of this function is to return the contents of the shipping container.
Create a derived class called ManualShippingContainer that represents the manual method of inventorying the container. In this method, a human simply attaches a textual description of all contents of the container. For example, the description might be “4 crates of apples. 10 crates of pears.” Add a new class variable of type string to store the manifest. Add a function called setManifest that sets this string. Override the getManifest function so that it returns this string.
Create a second derived class called RFIDShippingContainer that represents the RFID method of inventorying the container. To simulate what the RFID chips would compute, create an add function to simulate adding an item to the container. The class should store a list of all added items (as a string) and their quantity using the data structures of your choice. For example, if the add function were invoked three times as follows:
rfidContainer.add("crate of pears"); // Add one crate of pears rfidContainer.add("crate of apples"); // Add one crate of apples rfidContainer.add("crate of pears"); // Add one crate of pears |
At this point, the data structure should be storing a list of two items: crate of apples and crate of pears. The quantity of apples is 1and the quantity of pears is 2. Override the getManifest function so that it returns a string of all items that is built by traversing the list of items. In the example above, the return string would be “2 crate of pears. 1 crate of apples.“ Finally, write a main
You may need to convert an integer into a string. A simple way to do this in C++11 is: string s = to_string(intVariable);

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
EBK PROBLEM SOLVING WITH C++
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Using MIS (10th Edition)
Starting Out With Visual Basic (7th Edition)
C++ How to Program (10th Edition)
Database Concepts (7th Edition)
Starting Out with C++: Early Objects (9th Edition)
- In PPC, examples of a "Conversion" are (pick all that apply) Group of answer choices talking to a sales person signing up for a newsletter purchasing a new car clicking on an advertisementarrow_forwardThe language must be in python. Just do numbers 5-7 Neural Network Units Implement a single sigmoid neural network unit with weights of [-1.2, -1.1, 3.3, -2.1] Calculate the outputs for two training examples:Example 1: [0.9, 10.0, 3.1, 1]Example 2: [0.9, 2.1, 3.7, 1] Note that you don't have to explicitly include a threshold or bias since the examples include a last element of 1 which means that the last weight effectively operates as a threshold. Assuming that a sigmoid unit response >0.5 denotes a positive class and <0.5 is negative class, is example 1 positive or negative? is example 2 positive or negative? Create a single ReLU unit and provide the outputs for those examples. Calculate the derivative of the sigmoid with respect to net input for both examples Calculate the derivative of the ReLU with respect to net input for both examplesarrow_forwardComputer Science For an image represented by a given large 3d numpy array, use numpy to get a neighborhood filter using the mean of the pixels. For example, look through each of the image’s 3×3 window of pixels and replace outlier pixels with the mean value of the others in the 3×3 window. Set the resulting pixel value to be the mean value of a pixel and its eight neighbors. For pixels on an edge, use the mean value of the pixel and its five neighbors. For pixels on corners, use the mean value of the pixel and its three neighbors. If you loop over every pixel then you must store your input and output arrays separatelyarrow_forward
- Question5: Write a python program to train neural network to classify two clusters in a 2-dimensional space for implementing XOR, where the weight is -0.50 and x>-0.5. Also name the class by your namearrow_forwardThe language for coding must be in python Neural Network Units Implement a single sigmoid neural network unit with weights of [-1.2, -1.1, 3.3, -2.1] Calculate the outputs for two training examples:Example 1: [0.9, 10.0, 3.1, 1]Example 2: [0.9, 2.1, 3.7, 1] Note that you don't have to explicitly include a threshold or bias since the examples include a last element of 1 which means that the last weight effectively operates as a threshold. Assuming that a sigmoid unit response >0.5 denotes a positive class and <0.5 is negative class, is example 1 positive or negative? is example 2 positive or negative? Create a single ReLU unit and provide the outputs for those examples. Calculate the derivative of the sigmoid with respect to net input for both examples Calculate the derivative of the ReLU with respect to net input for both examplesarrow_forwardYou will be given a square chess board with one queen and a number of obstacles placed on it. Determine how many squares the queen can attack. A queen is standing on an chessboard. The chess board's rows are numbered from to , going from bottom to top. Its columns are numbered from to , going from left to right. Each square is referenced by a tuple, , describing the row, , and column, , where the square is located. The queen is standing at position . In a single move, she can attack any square in any of the eight directions (left, right, up, down, and the four diagonals). In the diagram below, the green circles denote all the cells the queen can attack from : There are obstacles on the chessboard, each preventing the queen from attacking any square beyond it on that path. For example, an obstacle at location in the diagram above prevents the queen from attacking cells , , and : Given the queen's position and the locations of all the obstacles, find and print the number of…arrow_forward
- in java using import java.util.Scanner; Bowling involves 10 frames. Each frame starts with 10 pins. The bowler has two throws to knock all 10 pins down. The total score is the sum of pins knocked down, with some special rules. For the first 9 frames: If all 10 pins are knocked down on a frame's first throw (a "strike"), that frame's score is the previous frame plus 10 plus the next two throws. (No second throw is taken). If all 10 pins are knocked down after a frame's second throw (a "spare"), that frame's score is the previous frame plus 10 plus the next throw. In the 10th frame, if the bowler's first throw is a strike, or the first two throws yields a spare, the bowler gets a third throw. The 10th frame's score is the previous frame's score plus the pins knocked down in the 10th frame's two or three throws. Given integers represents all throws for a game, output on one line each frame's score followed by a space (and end with a newline). Note that the number of throws may be as…arrow_forwardComputer Science Given a dataframe with two columns (x and y), I have found a function on Python that gives a local fit value (essentially between two set values x it gives the average of all values of y). Now the questions asks us to create 2 graphs - one that shows the average per value of x and another one that shows the difference to that average that we found. What does this mean?arrow_forwardonly java program, a word search puzzle, where one is presented with a grid of letters and needs to find a word by choosing adjacent letters, is the Knight-move word search puzzle, where you’re presented with a grid of letters and need to find a word in a configuration where adjacent letters in the word are reachable by moving like a knight in chess. A knight in chess moves from its current spot by either two rows and one column, or two columns and one row, in any direction. So, the knight at position "K" in the table below, can move to any of the spaces labeled "X" Write a java program that tries to find a word in a grid of letters where each letter is connected to the next by a knight move. Some specific puzzle rules: •You can never "wrap around" any edge of the grid •You can never use the same letter twice. There will be several input instances from a data file. Each input will begin with values r and c (both ≤ 8), denoting the dimensions of the grid. Values of r or c of 0 signify…arrow_forward
- Suppose a Genetic Algorithm uses chromosomes of the form x=abcdef with a fixed length of six genes.Each gene can be any digit between 0 and 9 . Let the fitness of individual x be calculated as : f(x) =(a+b)-(c+d)-(e+f) And let the initial population consist of four individuals x1, ... ,x4 with the following chromosomes : x1 = 3 5 3 2 6 5, x2 = 9 8 0 1 2 2, x3 = 1 2 2 1 2 3, x4 = 7 9 2 3 1 1 1. 1.Evaluate the fitness of each individual, showing all your workings, and arrange them in order with the fittest first and the least fit last. 2. calculate the average fitness. 3. Perform the following crossover operations:a- Cross the fittest two individuals using one point crossover at the middle point.b- Cross the second and third fittest individuals using a two point crossover (points b and e). Suppose the new population consists of the four offspring individuals received by the crossover operations in the above question. Evaluate the fitness of the new population, showing all your…arrow_forwardImplement the"paint fill"function that one might see on many image editing programs. That is, given a screen (represented by a two-dimensional array of colors), a point, and a new color, fill in the surrounding area until the color changes from the original color.arrow_forwardThe unary numeral system is a numeral system to represent natural numbers as sequences of ones, that is, to represent a number ?, a symbol representing “1” is repeated ? times. For example, the number 4 is represented by 1111. The number 0 would be represented by the empty string. Create a Turing machine which adds 3 to a natural number, that is, writes three times a 1 after the given unary number. Afterwards move the head to the left-most position of input.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
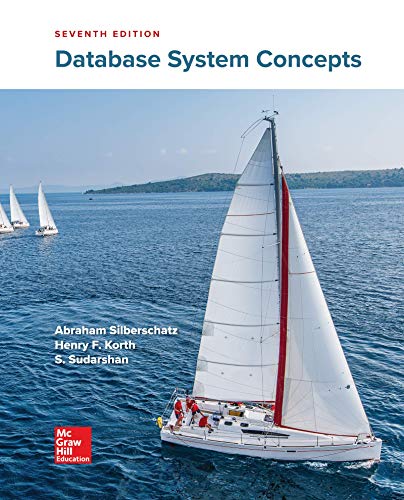
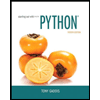
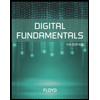
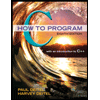
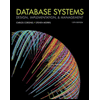
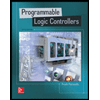