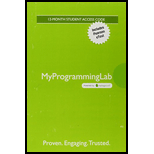
Program Plan:
- Include the required import statement.
- Define the main class.
- Declare the necessary variables
- Using start initialize the required.
- Create the border pane, radio button, text field, toggle group and button.
- Set everything into the panel.
- Add the actions event to the button.
- Create a scene and place the pane in the stage.
- Set the title.
- Place the scene in the stage.
- Display the stage.
- Define the main method using public static main.
- Initialize the call.

The below program will display the statement in different colors and move the statement left and right using GUI as follows:
Explanation of Solution
Program:
//import statement
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
//definition of "Test" class
public class Test extends Application
{
//declare the required variables
private double pane_width = 500;
private double pane_height = 150;
@Override
/*start method gets overridden in the application class*/
public void start(Stage pri_stage)
{
//create a text
Text t = new Text(20, 40, "
//set the font
t.setFont(new Font("Times", 20));
//create a text
Pane p = new Pane();
//create a label
p.getChildren().add(t);
//set the style
p.setStyle("-fx-border-color: gray");
//create a radio buttons
RadioButton r_red = new RadioButton("Red");
RadioButton r_yellow = new RadioButton("Yellow");
RadioButton r_black = new RadioButton("Black");
RadioButton r_orange = new RadioButton("Orange");
RadioButton r_green = new RadioButton("Green");
//create a toggle group
ToggleGroup group = new ToggleGroup();
//set the toggle groups
r_red.setToggleGroup(group);
r_yellow.setToggleGroup(group);
r_black.setToggleGroup(group);
r_black.setSelected(true);
r_orange.setToggleGroup(group);
r_green.setToggleGroup(group);
//create a box
HBox h_box = new HBox(5);
//create a label
h_box.getChildren().addAll(r_red, r_yellow, r_black, r_orange, r_green);
//set the alignment
h_box.setAlignment(Pos.CENTER);
//create a button
Button bt_left = new Button("<=");
Button bt_right = new Button("=>");
//create a box for button
HBox h_boxForButtons = new HBox(5);
//create a label
h_boxForButtons.getChildren().addAll(bt_left, bt_right);
//set the alignment
h_boxForButtons.setAlignment(Pos.CENTER);
//create a border pane
BorderPane border_pane = new BorderPane();
//set the border pane
border_pane.setTop(h_box);
border_pane.setCenter(p);
border_pane.setBottom(h_boxForButtons);
// create a scene and place it in the stage
Scene scene = new Scene(border_pane, pane_width, pane_height + 40);
//set the stage title
pri_stage.setTitle("Exercise16_01");
//place the scene in the stage
pri_stage.setScene(scene);
//display the stage
pri_stage.show();
// action event for the buttons gets created
r_red.setOnAction(e->t.setStroke(Color.RED));
r_yellow.setOnAction(e->t.setStroke(Color.YELLOW));
r_black.setOnAction(e->t.setStroke(Color.BLACK));
r_orange.setOnAction(e->t.setStroke(Color.ORANGE));
r_green.setOnAction(e->t.setStroke(Color.GREEN));
bt_left.setOnAction(e->t.setX(t.getX() - 1));
bt_right.setOnAction(e->t.setX(t.getX() + 1));
}
//definition of main method
public static void main(String[] args)
{
//initilaize calls
launch(args);
}
}
Want to see more full solutions like this?
Chapter 16 Solutions
MyLab Programming with Pearson eText -- Access Card -- for Introduction to Java Programming and Data Structures, Comprehensive Version
- Using JAVA code: Please make a GUI that will convert infix to postfix expression. In the interface, please show also the process of the conversion (line by line).arrow_forwardcreate a flowchart of the following each buttom in GUI:arrow_forwardMake a python code that will show the GUI belowarrow_forward
- can you please run this and show me the gui productarrow_forwardUsing square braces. In the previous question you had used the dot notation to add key values in an object and in this you have to add squares braces and print the object before and after addingarrow_forward- Challenge Activity 1: Stadium Seating Revenue Calculation Tool There are three seating categories at a stadium. Class A seats cost $20, Class B seats cost $15, and Class C seats cost $10. Write a program (a GUI program) ON *PYTHON*that asks how many tickets for each class of seats were sold, then displays the amount of income generated from ticket sales.arrow_forward
- Add additional functionalites to this code. For one room add this riddle, "The shorter I am, the bigger I am. What am I?" And the only way to continue to the next room is to type, "A temper," or "temper." And for another room, a help command. For example, the user will type, "help," and they will be told where to go next so they can each the winning room.arrow_forwardHow do you change the background color of a component? How do you change the color of text displayed by a label or a button?arrow_forwardI need help with creating a Java program described in the image belowarrow_forward
- Use java how to make my reset button work? I'm looking for implementation code step by step to activate the resert button When user clicks on the "Reset" button, form fields should reset to default values.arrow_forwardUse the given Java code on the left side of the photo:arrow_forwardPlease help with the below using java. Include getters and setters and comment where they are on the code. Please also comment the whole codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
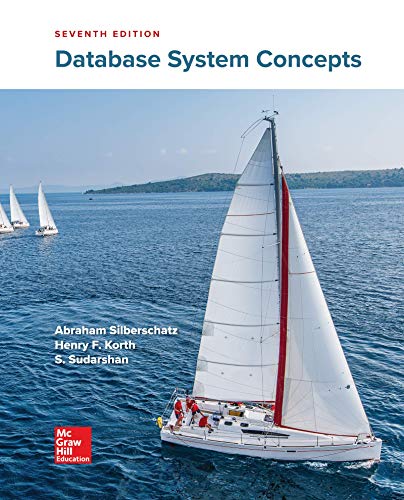
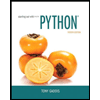
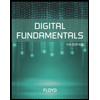
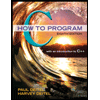
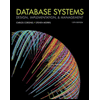
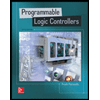